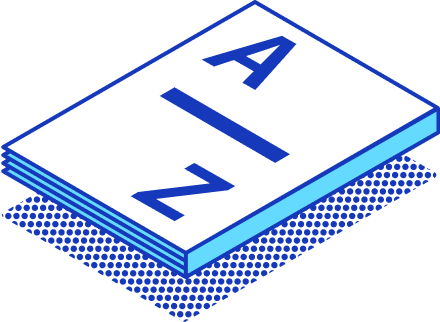
ANSI SQL
What is SQL?
SQL, or Structured Query Language, serves as the standard language for managing and manipulating relational databases. The American National Standards Institute (ANSI) developed SQL to ensure compatibility across different database systems. SQL allows users to perform various operations on data, such as querying, updating, and deleting records. The language provides a unified framework for interacting with databases, making it an essential tool for data professionals.
Key Features of SQL
SQL offers several key features that make it indispensable in database management:
-
Data Retrieval: SQL enables users to retrieve specific data from large datasets efficiently.
-
Data Manipulation: Users can insert, update, and delete data within tables.
-
Data Definition: SQL allows the creation and modification of database structures, including tables and indexes.
-
Data Control: SQL provides mechanisms for controlling access to data through permissions and roles.
These features collectively enhance the functionality and flexibility of relational database management systems (RDBMS).
ANSI SQL Standards
Evolution of ANSI SQL Standards
The standardization of SQL began with the release of SQL-86 by ANSI. This initial standard laid the groundwork for SQL as a universal language for managing and querying data in RDBMS. Subsequent updates in 1989 and 1992 introduced significant enhancements, further solidifying SQL's role in database management. The evolution of ANSI SQL standards has continued, with each iteration incorporating new features and improvements to meet the growing demands of data management.
Importance of Standardization
Standardization plays a crucial role in ensuring the portability and interoperability of SQL applications across different database systems. By adhering to ANSI SQL standards, developers can write SQL code that works seamlessly on various platforms, including MySQL, PostgreSQL, SQL Server, and Oracle. This standardization fosters greater conformity among vendors, reducing the complexity of database management and enhancing career opportunities for data professionals.
SQL Syntax and Structure
Basic SQL Commands
SQL commands fall into several categories, each serving a specific purpose:
-
Data Definition Language (DDL): Commands like
CREATE
,ALTER
, andDROP
define and modify database structures. -
Data Manipulation Language (DML): Commands such as
SELECT
,INSERT
,UPDATE
, andDELETE
manipulate data within tables. -
Data Control Language (DCL): Commands like
GRANT
andREVOKE
manage user permissions and access control.
These basic commands form the foundation of SQL operations, enabling users to interact with relational databases effectively.
SQL Query Structure
An SQL query typically follows a structured format, consisting of several key components:
-
SELECT: Specifies the columns to retrieve.
-
FROM: Identifies the table(s) from which to retrieve data.
-
WHERE: Filters records based on specified conditions.
-
GROUP BY: Groups records sharing common values.
-
HAVING: Filters groups based on specified conditions.
-
ORDER BY: Sorts the result set by specified columns.
This structured approach allows users to construct complex queries for precise data retrieval and analysis.
Core Components of ANSI SQL
Data Definition Language (DDL)
Data Definition Language (DDL) forms the backbone of database structure management in ANSI SQL. DDL commands define and modify the schema of a database, ensuring the proper organization and storage of data.
CREATE, ALTER, DROP Statements
-
CREATE: The
CREATE
statement initializes new database objects. These objects include tables, indexes, and views. For example,CREATE TABLE Employees (ID INT, Name VARCHAR(100));
creates a new table named Employees with columns for ID and Name. -
ALTER: The
ALTER
statement modifies existing database objects. Changes can include adding or dropping columns from a table. For instance,ALTER TABLE Employees ADD COLUMN Age INT;
adds a new column named Age to the Employees table. -
DROP: The
DROP
statement removes database objects. This action is irreversible. An example isDROP TABLE Employees;
, which deletes the Employees table.
Examples of DDL Commands
-
Creating a Table:
CREATE TABLE Departments (
DeptID INT PRIMARY KEY,
DeptName VARCHAR(100)
); -
Altering a Table:
ALTER TABLE Departments ADD COLUMN Location VARCHAR(100);
-
Dropping a Table:
DROP TABLE Departments;
Data Manipulation Language (DML)
Data Manipulation Language (DML) enables interaction with data stored in the database. DML commands allow users to retrieve, insert, update, and delete data.
SELECT, INSERT, UPDATE, DELETE Statements
-
SELECT: The
SELECT
statement retrieves data from one or more tables. For example,SELECT * FROM Employees;
fetches all records from the Employees table. -
INSERT: The
INSERT
statement adds new records to a table. An example isINSERT INTO Employees (ID, Name) VALUES (1, 'John Doe');
. -
UPDATE: The
UPDATE
statement modifies existing records. For instance,UPDATE Employees SET Name = 'Jane Doe' WHERE ID = 1;
changes the name of the employee with ID 1. -
DELETE: The
DELETE
statement removes records from a table. An example isDELETE FROM Employees WHERE ID = 1;
, which deletes the record with ID 1.
Examples of DML Commands
-
Selecting Data:
SELECT DeptName FROM Departments WHERE Location = 'New York';
-
Inserting Data:
INSERT INTO Departments (DeptID, DeptName, Location) VALUES (1, 'HR', 'New York');
-
Updating Data:
UPDATE Departments SET Location = 'San Francisco' WHERE DeptID = 1;
-
Deleting Data:
DELETE FROM Departments WHERE DeptID = 1;
Data Control Language (DCL)
Data Control Language (DCL) manages access to data within the database. DCL commands control permissions and security.
GRANT, REVOKE Statements
-
GRANT: The
GRANT
statement provides specific privileges to users. For example,GRANT SELECT ON Employees TO User1;
allows User1 to execute SELECT queries on the Employees table. -
REVOKE: The
REVOKE
statement removes previously granted privileges. An example isREVOKE SELECT ON Employees FROM User1;
, which revokes the SELECT privilege from User1.
Examples of DCL Commands
-
Granting Privileges:
GRANT INSERT, UPDATE ON Employees TO User2;
-
Revoking Privileges:
REVOKE INSERT ON Employees FROM User2;
Advanced SQL Concepts
Joins and Subqueries
Joins and subqueries are essential components of ANSI SQL. These concepts enable complex data retrieval from multiple tables.
Types of Joins
Joins combine rows from two or more tables based on a related column. ANSI SQL supports several types of joins:
-
Inner Join: Returns rows with matching values in both tables. Inner joins focus on commonality between tables.
-
Left Outer Join: Returns all rows from the left table and matching rows from the right table. Non-matching rows from the right table result in NULL values.
-
Right Outer Join: Returns all rows from the right table and matching rows from the left table. Non-matching rows from the left table result in NULL values.
-
Full Outer Join: Returns rows when there is a match in one of the tables. This join includes rows that do not have matching values in the other table.
For example, an inner join between Employees
and Departments
on the DeptID
column retrieves only employees with corresponding department records.
SELECT Employees.Name, Departments.DeptName
FROM Employees
INNER JOIN Departments ON Employees.DeptID = Departments.DeptID;
Subquery Examples
Subqueries, also known as nested queries, allow embedding one query within another. Subqueries provide a way to perform operations in multiple steps.
For example, a subquery can find employees with salaries above the average salary:
SELECT Name
FROM Employees
WHERE Salary > (SELECT AVG(Salary) FROM Employees);
Subqueries enhance the flexibility and power of ANSI SQL by enabling complex data retrieval scenarios.
Indexes and Views
Indexes and views optimize database performance and simplify data access. ANSI SQL provides robust support for these features.
Purpose and Benefits of Indexes
Indexes improve query performance by allowing faster data retrieval. Indexes create a sorted structure that the database engine can search efficiently.
Benefits of indexes include:
-
Improved Query Performance: Speeds up data retrieval operations.
-
Efficient Sorting: Facilitates quick sorting of data.
-
Enhanced Filtering: Accelerates filtering operations using WHERE clauses.
Creating an index on the Employees
table's Name
column:
CREATE INDEX idx_name ON Employees(Name);
Creating and Using Views
Views are virtual tables representing the result of a query. Views simplify complex queries by encapsulating them into a single object.
Creating a view for active employees:
CREATE VIEW ActiveEmployees AS
SELECT Name, DeptID
FROM Employees
WHERE Status = 'Active';
Using the view to retrieve data:
SELECT * FROM ActiveEmployees;
Views enhance data security and simplify query management in ANSI SQL.
Transactions and Concurrency
Transactions ensure data integrity and consistency in multi-user environments. ANSI SQL provides mechanisms to manage transactions and concurrency.
ACID Properties
Transactions in ANSI SQL adhere to ACID properties:
-
Atomicity: Ensures all operations within a transaction complete successfully or none at all.
-
Consistency: Guarantees the database remains in a valid state before and after the transaction.
-
Isolation: Ensures concurrent transactions do not interfere with each other.
-
Durability: Ensures committed transactions persist even in case of system failures.
These properties maintain data integrity and reliability.
Managing Transactions
ANSI SQL uses commands to manage transactions:
-
BEGIN TRANSACTION: Starts a new transaction.
-
COMMIT: Saves all changes made during the transaction.
-
ROLLBACK: Reverts all changes made during the transaction.
Example of managing a transaction:
BEGIN TRANSACTION;
UPDATE Employees SET Salary = Salary * 1.1 WHERE DeptID = 1;
IF @@ERROR <> 0
ROLLBACK;
ELSE
COMMIT;
Managing transactions ensures data consistency and handles errors effectively in ANSI SQL.
Practical Applications of ANSI SQL
Real-World Use Cases
Business Intelligence and Reporting
Business intelligence (BI) tools rely heavily on ANSI SQL for data analysis and reporting. Organizations use ANSI SQL to extract valuable insights from large datasets. SQL queries enable the generation of detailed reports, dashboards, and visualizations. These tools help businesses make informed decisions based on data trends and patterns.
For example, a company might use ANSI SQL to analyze sales data. The SQL queries can identify top-selling products, customer preferences, and seasonal trends. This information allows the company to adjust marketing strategies and optimize inventory management.
Data Warehousing
Data warehousing involves the consolidation of data from multiple sources into a single repository. ANSI SQL plays a crucial role in this process. SQL commands facilitate the extraction, transformation, and loading (ETL) of data into the warehouse. This ensures that the data is clean, consistent, and ready for analysis.
In a data warehouse, ANSI SQL enables complex queries across vast amounts of data. Analysts can perform aggregations, joins, and subqueries to uncover hidden insights. For instance, a retail chain might use ANSI SQL to analyze customer purchase behavior across different regions. This analysis helps in tailoring marketing campaigns and improving customer service.
SQL in Modern Applications
Integration with Programming Languages
Modern applications often require seamless integration between databases and programming languages. ANSI SQL provides a standardized way to interact with relational databases. Developers use SQL to perform database operations within their code, ensuring data consistency and integrity.
Popular programming languages like Python, Java, and C# offer libraries and frameworks for working with ANSI SQL. These tools allow developers to execute SQL queries, manage transactions, and handle errors programmatically. For example, a web application might use Python's sqlite3
library to interact with a SQLite database. The application can perform CRUD (Create, Read, Update, Delete) operations using ANSI SQL commands embedded in the Python code.
SQL in Web Development
Web development relies on ANSI SQL for managing backend databases. Websites and web applications use SQL to store and retrieve user data, content, and other information. ANSI SQL ensures that the database interactions are efficient and secure.
Content management systems (CMS) like WordPress and Drupal use ANSI SQL to manage their databases. These systems allow users to create, edit, and publish content through a web interface. The underlying SQL queries handle the data storage and retrieval, ensuring that the content is accessible and up-to-date.
E-commerce platforms also depend on ANSI SQL for managing product catalogs, customer orders, and payment transactions. SQL queries enable real-time updates to inventory levels and order statuses. This ensures a smooth shopping experience for customers and efficient operations for the business.
Conclusion
The blog has explored the essential aspects of ANSI SQL. Mastering ANSI SQL proves crucial for anyone working with data. ANSI SQL provides a standardized framework that ensures compatibility across various database systems. This standardization fosters interoperability and enhances career opportunities in the data field.
Practicing and applying ANSI SQL skills can significantly improve data manipulation and analysis capabilities. Continuous learning and hands-on experience with ANSI SQL commands will build proficiency. The future of SQL includes advancements in data warehousing, business intelligence, and integration with modern applications.