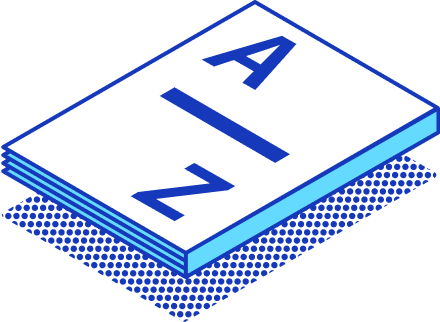
Base64 Encoding
What is Base64 Encoding?
Base64 Encoding represents binary data in an ASCII string format. This encoding scheme transforms binary data into a sequence of printable characters. Base64 Encoding is essential for carrying data stored in binary formats across channels that only reliably support text content. The method ensures the safe transmission of data by converting binary data into a format that can survive transport through layers designed to deal with text.
How Base64 Encoding Works
Base64 Encoding works by dividing binary data into 6-bit chunks. Each chunk is then mapped to a corresponding character in the Base64 index table. The encoding process converts binary data into a stream of ASCII characters, ensuring compatibility across various applications and platforms. Base64 Encoding uses 64 basic characters for encoding, including uppercase and lowercase letters, digits, and special characters.
Technical Details
Encoding Process
The encoding process begins by taking binary data as input. The algorithm divides the binary data into 6-bit segments. Each segment is then converted into a decimal number. These decimal numbers are mapped to corresponding characters in the Base64 alphabet. The result is a string of Base64-encoded characters. This string represents the original binary data in a text format.
Decoding Process
The decoding process reverses the encoding steps. The algorithm takes a Base64-encoded string as input. Each character in the string is mapped back to its corresponding 6-bit binary value. These binary values are concatenated to form the original binary data. The decoding process ensures that the data can be accurately reconstructed from the Base64-encoded string.
Base64 Alphabet
Characters Used
The Base64 alphabet consists of 64 characters. These characters include uppercase letters (A-Z), lowercase letters (a-z), digits (0-9), and two special characters (+ and /). This set of characters ensures that the encoded data remains within the printable ASCII range. The use of these characters allows Base64 Encoding to represent binary data in a text format.
Padding and Special Characters
Padding plays a crucial role in Base64 Encoding. The algorithm adds padding characters (=) to the encoded string to ensure that the length of the string is a multiple of four. Padding helps maintain the integrity of the encoded data during transmission. Special characters like + and / are used to represent specific binary values. These characters help encode a wide range of binary data into a compact text format.
Use Cases of Base64 Encoding
Data Transmission
Email Attachments
Base64 Encoding plays a vital role in email communication. Email protocols like SMTP can only handle text data. Binary files, such as images or documents, need conversion into a text format. Base64 Encoding converts these binary files into ASCII strings. This process ensures that email attachments remain intact during transmission. The encoded data can be decoded back into its original form by the recipient's email client.
Embedding Images in HTML/CSS
Web developers often embed images directly into HTML or CSS files. Base64 Encoding allows this by converting image files into ASCII strings. These strings can be included within HTML or CSS code. This method eliminates the need for separate image files. Embedding images using Base64 Encoding simplifies the deployment process. It also reduces the number of HTTP requests, improving page load times.
Data Storage
Storing Binary Data in Text Files
Storing binary data in text files presents challenges. Many text editors and systems cannot handle raw binary data. Base64 Encoding offers a solution by converting binary data into a text format. This encoded text can be stored in standard text files. Developers can easily read and write these files using common text processing tools. Base64 Encoding ensures compatibility across different platforms and applications.
Database Storage
Databases often store binary data, such as images or documents. Directly storing binary data in databases can lead to issues with data integrity and compatibility. Base64 Encoding converts binary data into ASCII strings. These strings can be safely stored in database fields designed for text. This approach ensures that binary data remains intact and accessible. Base64 Encoding also simplifies data retrieval and manipulation within database systems.
Security Applications
Encoding Sensitive Data
Security applications frequently use Base64 Encoding to protect sensitive data. Encoding data before transmission adds an extra layer of security. Base64 Encoding converts sensitive information into a non-human-readable format. This makes it harder for unauthorized parties to interpret the data. While not a substitute for encryption, Base64 Encoding provides basic obfuscation. It enhances the overall security of data transmission.
Basic Obfuscation
Base64 Encoding serves as a simple method for obfuscating data. Obfuscation involves making data less understandable to unauthorized users. Base64 Encoding converts readable data into a seemingly random string of characters. This process helps protect data from casual inspection. Developers use Base64 Encoding to obscure API keys, configuration files, and other sensitive information. While not foolproof, it adds a layer of protection against unauthorized access.
Advantages and Disadvantages
Advantages
Universality and Compatibility
Base64 Encoding offers universal compatibility across various platforms and applications. The method converts binary data into ASCII text, ensuring that the data can be transmitted through text-based protocols like HTTP and SMTP. This encoding scheme allows for seamless integration with different systems, making it a versatile tool in modern computing. The use of printable characters ensures that the encoded data remains readable and easily transferable.
Simplicity and Ease of Use
Base64 Encoding provides a straightforward and easy-to-implement solution for encoding binary data. The process involves simple steps that can be easily understood and executed by developers. Base64 Encoding does not require complex algorithms or extensive computational resources. The method's simplicity makes it accessible to developers with varying levels of expertise. This ease of use contributes to its widespread adoption in various applications.
Disadvantages
Increased Data Size
One significant drawback of Base64 Encoding is the increase in data size. The encoding process converts binary data into a text format, resulting in a 33% increase in the size of the original data. This increase can lead to higher storage requirements and longer transmission times. While Base64 Encoding ensures compatibility and readability, the larger data size can impact performance, especially in bandwidth-constrained environments.
Performance Overhead
Base64 Encoding introduces a performance overhead due to the additional processing required for encoding and decoding data. The method involves converting binary data into ASCII text and vice versa, which can consume computational resources. This performance overhead may not be significant for small data sets but can become a concern for larger volumes of data. Developers need to consider this overhead when implementing Base64 Encoding in performance-sensitive applications.
Practical Examples
Encoding and Decoding in Various Languages
Python
Python offers built-in support for Base64 encoding and decoding through the base64
module. Developers can easily encode binary data into a Base64 string using the b64encode
function. For decoding, the b64decode
function converts a Base64 string back to its original binary form. Here is an example:
import base64
# Encoding
data = b'Hello, World!'
encoded_data = base64.b64encode(data)
print(encoded_data) # Output: b'SGVsbG8sIFdvcmxkIQ=='
# Decoding
decoded_data = base64.b64decode(encoded_data)
print(decoded_data) # Output: b'Hello, World!'
Python's straightforward approach makes it a popular choice for handling Base64 encoding and decoding tasks.
JavaScript
JavaScript provides methods for Base64 encoding and decoding within the window
object. The btoa
function encodes a string to Base64, while the atob
function decodes a Base64 string back to its original form. Here is an example:
// Encoding
let data = 'Hello, World!';
let encodedData = btoa(data);
console.log(encodedData); // Output: SGVsbG8sIFdvcmxkIQ==
// Decoding
let decodedData = atob(encodedData);
console.log(decodedData); // Output: Hello, World!
JavaScript's native functions provide a simple way to perform Base64 operations in web applications.
Java
Java includes the java.util.Base64
class for encoding and decoding Base64 strings. The getEncoder
method returns a Base64 encoder, and the getDecoder
method returns a Base64 decoder. Here is an example:
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
// Encoding
String data = "Hello, World!";
String encodedData = Base64.getEncoder().encodeToString(data.getBytes());
System.out.println(encodedData); // Output: SGVsbG8sIFdvcmxkIQ==
// Decoding
byte[] decodedBytes = Base64.getDecoder().decode(encodedData);
String decodedData = new String(decodedBytes);
System.out.println(decodedData); // Output: Hello, World!
}
}
Java's robust library support ensures efficient Base64 encoding and decoding for enterprise-level applications.
Real-World Applications
Web Development
Web developers frequently use Base64 encoding to embed images directly into HTML or CSS files. This technique eliminates the need for separate image files, reducing the number of HTTP requests. Embedding images as Base64 strings can improve page load times and simplify the deployment process.
For example, an image encoded in Base64 can be included in an HTML img
tag:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUA...">
Base64 encoding streamlines the integration of media assets into web pages, enhancing user experience.
API Communication
APIs often require the transmission of binary data, such as files or images. Base64 encoding ensures that this binary data remains intact during transport over text-based protocols like HTTP. Many APIs accept Base64-encoded strings as input for file uploads or data submissions.
For instance, a JSON payload containing a Base64-encoded image might look like this:
{
"image": "iVBORw0KGgoAAAANSUhEUgAAAAUA..."
}
Base64 encoding facilitates secure and reliable data exchange between client applications and servers, making it indispensable in modern API communication.
Conclusion
Base64 encoding plays a crucial role in modern computing. This method ensures the safe transmission of binary data over text-based protocols. The encoding process converts binary data into a sequence of printable characters. Base64 encoding finds applications in email attachments, web development, and database storage. The method offers universality and simplicity but increases data size and introduces performance overhead.
Base64 encoding remains indispensable for data representation and transmission. The technique enhances compatibility and readability across various platforms. Future trends may see further optimizations to reduce data size and improve performance. Understanding Base64 encoding is essential for developers working with data transmission and security protocols.
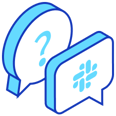
Join StarRocks Community on Slack
Connect on Slack