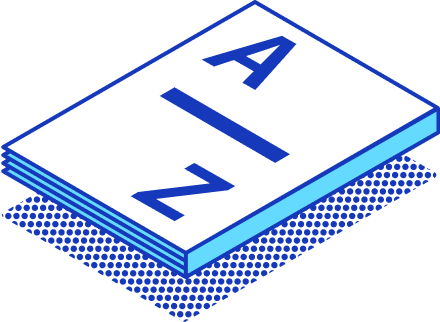
Composite Keys
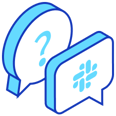
Join StarRocks Community on Slack
Connect on SlackWhat is a Composite Key?
A composite key in SQL combines two or more columns to uniquely identify each record in a table. Database designers use composite keys when a single column cannot ensure uniqueness. The combination of multiple columns provides a unique identifier for each row, enhancing data integrity and retrieval efficiency.
Examples of Composite Keys
Consider a table named Orders
. This table contains columns like OrderID
, ProductID
, and CustomerID
. Neither OrderID
nor ProductID
alone can uniquely identify a row. Combining OrderID
and ProductID
forms a composite key that uniquely identifies each order-product pair. Another example involves a Student
table with columns StudentID
, CourseID
, and Semester
. Combining StudentID
and CourseID
creates a composite key that uniquely identifies each student's course enrollment.
How Composite Keys Differ from Primary Keys
Primary Key Overview
A primary key uniquely identifies each record in a table. It consists of a single column or a combination of columns. Primary keys enforce uniqueness and prevent null values. Database designers often use primary keys to establish relationships between tables.
Differences and Similarities
Composite keys and primary keys both serve to uniquely identify records. However, a primary key can consist of a single column, while a composite key always involves multiple columns. Composite keys are particularly useful in complex database designs where no single column can ensure uniqueness. For example, in a many-to-many relationship, composite keys provide a robust solution for uniquely identifying records.
Practical Examples and Syntax
Creating Composite Keys in SQL
SQL Syntax for Composite Keys
Creating a composite key in SQL involves combining two or more columns to form a unique identifier. The syntax for defining a composite key varies slightly between different SQL databases, but the general approach remains consistent.
To create a composite key in SQL, use the PRIMARY KEY
constraint followed by the columns that will form the composite key. Here is an example in MySQL:
CREATE TABLE Orders (
OrderID INT,
ProductID INT,
CustomerID INT,
PRIMARY KEY (OrderID, ProductID)
);
In this example, the combination of OrderID
and ProductID
forms the composite key for the Orders
table. This ensures that each order-product pair is unique within the table.
Example Queries
Once a composite key is defined, various SQL operations can be performed using this key. Here are some example queries:
-
Inserting Data:
INSERT INTO Orders (OrderID, ProductID, CustomerID) VALUES (1, 101, 1001);
INSERT INTO Orders (OrderID, ProductID, CustomerID) VALUES (1, 102, 1002);These queries insert new rows into the
Orders
table. The composite key ensures that each combination ofOrderID
andProductID
remains unique. -
Selecting Data:
SELECT * FROM Orders WHERE OrderID = 1 AND ProductID = 101;
This query retrieves the row where
OrderID
is 1 andProductID
is 101. The composite key facilitates efficient data retrieval. -
Updating Data:
UPDATE Orders SET CustomerID = 1003 WHERE OrderID = 1 AND ProductID = 101;
This query updates the
CustomerID
for the specified composite key. -
Deleting Data:
DELETE FROM Orders WHERE OrderID = 1 AND ProductID = 101;
This query deletes the row with the specified composite key.
Using Composite Keys in Database Operations
Inserting Data with Composite Keys
Inserting data into a table with a composite key requires specifying values for all columns that form the key. This ensures that each combination remains unique. For example:
INSERT INTO Student (StudentID, CourseID, Semester) VALUES (123, 'CS101', 'Fall2023');
INSERT INTO Student (StudentID, CourseID, Semester) VALUES (123, 'CS102', 'Fall2023');
These queries insert records into the Student
table. The composite key (StudentID
, CourseID
) ensures that each student's course enrollment remains unique.
Querying Data with Composite Keys
Querying data from a table with a composite key involves specifying values for all columns that form the key. This allows precise data retrieval. For example:
SELECT * FROM Student WHERE StudentID = 123 AND CourseID = 'CS101';
This query retrieves the record where StudentID
is 123 and CourseID
is 'CS101'. The composite key ensures efficient and accurate data retrieval.
Composite keys enhance data integrity and retrieval efficiency. Database designers must understand the intricacies of composite keys to make informed decisions on their usage. Proper implementation of composite keys leads to robust and flexible database designs.
Advantages and Disadvantages
Advantages of Using Composite Keys
Enhanced Uniqueness
A composite key enhances the uniqueness of records in a database table. By combining multiple columns, a composite key ensures that each row remains distinct. This approach proves beneficial when no single column can guarantee uniqueness. For instance, in a table with employee names and IDs, combining these columns creates a unique identifier for each employee. This method prevents duplicate entries and maintains data integrity.
Improved Data Integrity
Composite keys improve data integrity by ensuring that each record remains unique. This uniqueness helps in maintaining consistency across tables. Composite keys manage complex relationships within a database. For example, in a student enrollment system, combining StudentID
and CourseID
ensures that each student's course registration remains unique. This combination prevents duplicate enrollments and maintains accurate records.
Disadvantages of Using Composite Keys
Complexity in Queries
Using composite keys can introduce complexity in queries. Queries involving composite keys require specifying values for all columns that form the key. This requirement can make SQL statements longer and harder to read. For example, a query to retrieve a specific order in an Orders
table with a composite key (OrderID
, ProductID
) needs both values:
SELECT * FROM Orders WHERE OrderID = 1 AND ProductID = 101;
This complexity can increase the likelihood of errors in query formulation.
Potential Performance Issues
Composite keys can lead to potential performance issues. The database must evaluate multiple columns to ensure uniqueness, which can slow down operations. Indexing composite keys can also consume more storage space and processing power. For large datasets, this overhead can impact the overall performance of the database. For example, inserting records into a table with a composite key requires checking the uniqueness of the combined columns, which can slow down the insertion process.
Best Practices
When to Use Composite Keys
Appropriate Scenarios
Composite keys prove essential in various database scenarios. Database designers should use composite keys when no single column can ensure uniqueness. For example, in a many-to-many relationship, composite keys provide a robust solution. Combining multiple columns creates a unique identifier for each row. This approach enhances data integrity and retrieval efficiency.
Composite keys also benefit complex database designs. In cases where multiple attributes together uniquely identify an entity, composite keys become necessary. For instance, in a student enrollment system, combining StudentID
and CourseID
ensures unique course registrations. This combination prevents duplicate entries and maintains accurate records.
Considerations for Design
Designing composite keys requires careful consideration. Database designers must ensure that the chosen columns together uniquely identify each record. The columns should have a logical relationship to each other. Avoid using columns that do not contribute to the uniqueness of the key.
Consider the impact on query performance. Composite keys can introduce complexity in SQL statements. Ensure that the database can handle the additional overhead. Indexing strategies play a crucial role in optimizing performance. Proper indexing can mitigate potential performance issues associated with composite keys.
Alternatives to Composite Keys
Single Column Primary Keys
Single column primary keys offer a simpler alternative to composite keys. A single column serves as the unique identifier for each record. This approach reduces query complexity. For example, using an auto-incrementing ID
column as the primary key simplifies data retrieval.
Single column primary keys work well in straightforward database designs. They provide a clear and concise way to ensure uniqueness. However, they may not suffice in complex scenarios where multiple attributes define uniqueness.
Unique Constraints
Unique constraints provide another alternative. These constraints enforce uniqueness on one or more columns without forming a composite key. For example, a unique constraint on Email
ensures that no two records share the same email address.
Unique constraints offer flexibility. They allow for enforcing uniqueness without the need for a composite key. This approach can simplify database design and improve query performance. However, unique constraints may not always replace the need for composite keys in complex relationships.
Comparisons with Related Concepts
Composite Keys vs. Foreign Keys
Definition of Foreign Keys
A foreign key in SQL establishes a link between two tables. The foreign key in one table points to the primary key in another table. This relationship enforces referential integrity within the database. For example, an Orders
table might have a foreign key that references the CustomerID
in a Customers
table.
Key Differences and Use Cases
Composite keys and foreign keys serve different purposes. Composite keys uniquely identify records within a single table. Foreign keys establish relationships between different tables. A composite key combines multiple columns to ensure uniqueness. A foreign key uses a single column or a combination of columns to reference another table's primary key.
Use Cases:
-
Composite Keys: Suitable for tables where no single column can ensure uniqueness. For example, a
StudentCourses
table might use a composite key ofStudentID
andCourseID
. -
Foreign Keys: Ideal for maintaining relationships between tables. For instance, an
Orders
table might use a foreign key to reference theCustomerID
in aCustomers
table.
Composite Keys vs. Unique Constraints
Definition of Unique Constraints
A unique constraint in SQL ensures that all values in a column or a set of columns are unique. Unlike composite keys, unique constraints do not serve as primary keys. Instead, they enforce uniqueness without forming a composite key. For example, a unique constraint on an Email
column ensures that no two records share the same email address.
Key Differences and Use Cases
Composite keys and unique constraints both enforce uniqueness. However, they differ in their application. Composite keys serve as primary keys, combining multiple columns to uniquely identify records. Unique constraints enforce uniqueness on one or more columns without forming a composite key.
Use Cases:
-
Composite Keys: Useful in complex database designs where multiple attributes together define uniqueness. For example, a
LibraryLoans
table might use a composite key ofBookID
andMemberID
. -
Unique Constraints: Suitable for enforcing uniqueness on individual columns or sets of columns. For instance, a
Users
table might have a unique constraint on theUsername
column to ensure no duplicate usernames exist.
In summary, understanding the differences and appropriate use cases for composite keys, foreign keys, and unique constraints enhances database design. Each concept plays a crucial role in maintaining data integrity and optimizing database performance.
Conclusion
Composite keys play a vital role in database schema design. They ensure data integrity and efficient retrieval, especially in complex scenarios. Database designers must understand the best practices for implementing composite keys. This knowledge leads to robust and efficient data models.
Experimenting with composite keys in SQL can enhance understanding and proficiency. This practice allows for more flexible and scalable database designs. Embrace the power of composite keys to create more effective data management solutions.