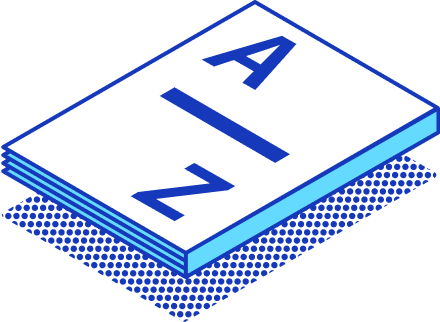
Create, Read, Update, and Delete (CRUD)
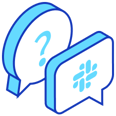
Join StarRocks Community on Slack
Connect on SlackWhat Is CRUD (Create, Read, Update, and Delete)?
The "Create" operation in CRUD (Create, Read, Update, and Delete) refers to adding new records to a database. This operation allows users to insert new data entries, ensuring the database grows and evolves with new information. The primary purpose of the Create operation is to enable the addition of unique data elements, which can be anything from user profiles to transaction records.
How Create Works in Databases
In databases, the Create operation involves inserting a new record into a table. This process typically requires specifying values for each field in the table. For instance, in a relational database, a SQL INSERT
statement accomplishes this task. The database management system (DBMS) ensures that the new record adheres to the defined schema and constraints, such as primary keys and foreign keys.
Examples of Create Operations
Examples of Create operations include:
-
Adding a new user to a web application's database.
-
Inserting a new product into an inventory management system.
-
Creating a new blog post in a content management system.
Read
Definition and Purpose
The "Read" operation in CRUD (Create, Read, Update, and Delete) involves retrieving existing data from a database. This operation allows users to access and view stored information without modifying it. The primary purpose of the Read operation is to provide users with the ability to query and display data as needed.
How Read Works in Databases
In databases, the Read operation involves executing a query to fetch data from one or more tables. For relational databases, a SQL SELECT
statement performs this function. The DBMS processes the query and returns the requested data, ensuring that it meets any specified criteria or filters.
Examples of Read Operations
Examples of Read operations include:
-
Retrieving a user's profile information from a social media platform.
-
Displaying a list of products in an online store.
-
Fetching transaction history for a bank account.
Update
Definition and Purpose
The "Update" operation in CRUD (Create, Read, Update, and Delete) refers to modifying existing records in a database. This operation allows users to change data entries, ensuring that the database remains accurate and up-to-date. The primary purpose of the Update operation is to enable the modification of data elements to reflect changes or corrections.
How Update Works in Databases
In databases, the Update operation involves altering one or more fields of an existing record. For relational databases, a SQL UPDATE
statement performs this task. The DBMS ensures that the updated record adheres to the defined schema and constraints, maintaining data integrity.
Examples of Update Operations
Examples of Update operations include:
-
Changing a user's email address in a web application's database.
-
Updating the price of a product in an inventory management system.
-
Modifying the content of a blog post in a content management system.
Delete
Definition and Purpose
The "Delete" operation in CRUD (Create, Read, Update, and Delete) involves removing existing records from a database. This operation allows users to eliminate outdated or unnecessary data. The primary purpose of the Delete operation is to maintain the database's relevance and accuracy by purging obsolete information.
How Delete Works in Databases
In databases, the Delete operation involves executing a command to remove one or more records from a table. For relational databases, a SQL DELETE
statement performs this task. The database management system (DBMS) ensures that the deleted record no longer exists in the table while maintaining the integrity of the remaining data.
Examples of Delete Operations
Examples of Delete operations include:
-
Removing a user's account from a web application's database.
-
Deleting an out-of-stock product from an inventory management system.
-
Erasing an outdated blog post from a content management system.
Practical Applications of CRUD
CRUD in Web Development
Using CRUD in RESTful APIs
RESTful APIs utilize CRUD (Create, Read, Update, and Delete) operations to manage resources efficiently. The "Create" operation corresponds to the HTTP POST
method, which allows the addition of new resources. The "Read" operation aligns with the HTTP GET
method, enabling data retrieval without modification. The "Update" operation uses the HTTP PUT
or PATCH
methods to alter existing resources. The "Delete" operation employs the HTTP DELETE
method to remove resources.
RESTful APIs provide a standardized way to interact with web services. Developers can perform CRUD operations using these HTTP methods. For instance, adding a new user to a database involves sending a POST
request with the user's details. Retrieving user information requires a GET
request. Modifying user data involves a PUT
or PATCH
request. Removing a user account necessitates a DELETE
request.
CRUD Operations in Frontend Frameworks
Frontend frameworks like React, Angular, and Vue.js implement CRUD operations to manage application state and user interactions. These frameworks use components and state management libraries to handle data changes. The "Create" operation involves adding new elements to the application's state. The "Read" operation retrieves and displays data to the user. The "Update" operation modifies existing elements in the state. The "Delete" operation removes elements from the state.
For example, a React application might use the useState
hook to manage a list of items. Adding a new item involves updating the state with a new entry. Displaying the list of items requires reading the state and rendering the components. Modifying an item involves updating the state with the new data. Removing an item requires filtering the state to exclude the deleted entry.
CRUD in Database Management Systems
SQL and CRUD
SQL databases rely on CRUD operations to manage data. The "Create" operation uses the INSERT
statement to add new records to a table. The "Read" operation employs the SELECT
statement to retrieve data. The "Update" operation utilizes the UPDATE
statement to modify existing records. The "Delete" operation uses the DELETE
statement to remove records.
Efficient database design is crucial for optimal CRUD operations. Poor design can negatively impact performance. For instance, performing deletions in batches for large datasets can avoid locking issues. Using transactions provides rollback capabilities, ensuring data integrity.
NoSQL and CRUD
NoSQL databases also implement CRUD operations but with different syntax and methodologies. The "Create" operation involves adding new documents or records. The "Read" operation retrieves documents based on specific queries. The "Update" operation modifies existing documents. The "Delete" operation removes documents from the database.
NoSQL databases like MongoDB use JSON-like documents to store data. Adding a new document involves inserting it into a collection. Retrieving documents requires querying the collection with specific criteria. Modifying a document involves updating its fields. Removing a document requires deleting it from the collection.
Testing and Performance Considerations
Testing CRUD Operations
Unit Testing
Unit testing involves verifying individual components of an application to ensure each part functions correctly. For CRUD (Create, Read, Update, and Delete) operations, unit tests focus on validating the correctness of each operation. Developers write test cases to check if the Create
function correctly adds new records to the database. The Read
function should accurately retrieve data without errors. The Update
function must modify existing records as intended. The Delete
function should remove records without leaving residual data.
Unit tests help identify bugs early in the development process. Automated testing tools like JUnit for Java or PHPUnit for PHP can streamline this process. These tools allow developers to run multiple tests quickly, ensuring that CRUD operations perform as expected.
Integration Testing
Integration testing examines how different components of an application work together. For CRUD operations, integration tests verify that the database interacts correctly with the application. These tests ensure that data flows seamlessly between the user interface and the database.
Integration tests might involve scenarios where a user creates a new account, updates profile information, retrieves transaction history, and deletes an outdated entry. Tools like Selenium or Postman can facilitate integration testing by simulating user actions and API requests. Successful integration tests confirm that CRUD operations function cohesively within the broader application.
Performance Optimization
Indexing Strategies
Indexing strategies play a crucial role in optimizing the performance of CRUD operations. Indexes improve the speed of data retrieval by allowing the database to locate records more efficiently. For example, creating an index on a user ID field can significantly reduce the time required for Read
operations.
However, indexes also impact Create
, Update
, and Delete
operations. Adding a new record requires updating the index, which can slow down the Create
process. Similarly, modifying or deleting a record necessitates index adjustments. Therefore, developers must balance the benefits of faster queries with the potential overhead on other CRUD operations.
Query Optimization
Query optimization enhances the performance of Read
operations by refining how the database processes queries. Efficient queries minimize the load on the database, reducing response times and improving overall performance. Developers can use techniques like selecting only necessary fields, avoiding complex joins, and using query caching.
For instance, instead of retrieving all columns from a table, a query can specify only the required fields. This approach reduces the amount of data transferred and speeds up the Read
operation. Query optimization also involves analyzing execution plans to identify bottlenecks and adjust queries accordingly.
Conclusion
CRUD (Create, Read, Update, and Delete) operations form the foundation of database management. These operations enable efficient data manipulation and retrieval. Implementing CRUD effectively requires understanding indexing strategies and query optimization techniques. Exploring CRUD in various applications can enhance data management skills. Developers should consider performance factors when designing systems that rely on CRUD operations.