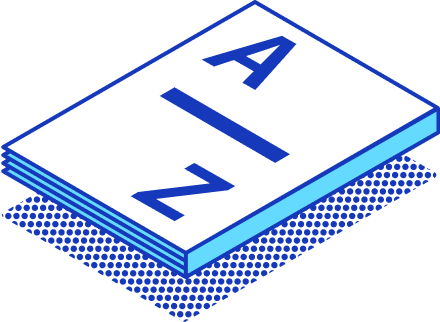
Foreign Keys Explained: Essential Concepts and Best Practices
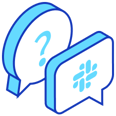
Join StarRocks Community on Slack
Connect on SlackWhat Are Foreign Keys?
Foreign keys are fundamental to relational database design, ensuring data consistency and enforcing relationships between tables. A foreign key is a column (or a set of columns) in one table that references the primary key of another table. This linkage enforces referential integrity, meaning every value in the foreign key column must correspond to an existing value in the referenced table.
Example:
Consider a students table and a classes table. The class_id
in the students table serves as a foreign key referencing the class_id
in the classes table:
-
Classes Table (Parent Table):
CREATE TABLE classes (
class_id INT PRIMARY KEY,
class_name VARCHAR(50) NOT NULL
); -
Students Table (Child Table):
CREATE TABLE students (
student_id INT PRIMARY KEY,
student_name VARCHAR(50) NOT NULL,
class_id INT,
FOREIGN KEY (class_id) REFERENCES classes(class_id)
);
This setup ensures that a student must belong to an existing class.
How Foreign Keys Differ from Primary Keys
Feature | Primary Key | Foreign Key |
---|---|---|
Purpose | Uniquely identifies each row | Establishes a link between tables |
Uniqueness | Must be unique | Can have duplicate values |
Nullability | Cannot contain NULL values | Can contain NULL values unless restricted |
Number per Table | One per table | Multiple foreign keys per table |
Purpose and Benefits of Foreign Keys
Foreign keys play a vital role in relational databases, ensuring data integrity, consistency, and efficient management. The following are key benefits of foreign keys:
1. Enforcing Data Consistency
Foreign keys prevent invalid relationships by ensuring that references between tables remain valid. This avoids the risk of orphaned records and incorrect dependencies.
-
Example: If a student’s
class_id
references a non-existent class, the database rejects the entry, ensuring consistency.
2. Preventing Data Anomalies
Data anomalies occur when records are improperly referenced or lost. Foreign keys eliminate insertion, deletion, and update anomalies by maintaining strict relationships between tables.
-
Insertion anomaly: Prevents adding a student with a non-existent
class_id
. -
Deletion anomaly: Ensures that deleting a class does not leave students assigned to an invalid class.
-
Update anomaly: Ensures changes to
class_id
propagate correctly.
3. Enabling Cascading Actions
Foreign keys support ON DELETE CASCADE and ON UPDATE CASCADE, automating actions that prevent inconsistent data.
-
Example: If
class_id
changes in the classes table, the update propagates to all students assigned to that class.
4. Improving Query Efficiency
Foreign keys, when properly indexed, speed up JOIN operations, reducing execution time when retrieving related data across multiple tables.
-
Example: Retrieving all students in a specific class using a JOIN query is optimized when
class_id
is indexed.
5. Supporting Database Normalization
Foreign keys enable database normalization by dividing data into logically organized tables, reducing redundancy and improving maintainability.
-
Example: Instead of storing class names in multiple places, they are stored once in classes, with students referencing the class via
class_id
.
Technical Aspects of Foreign Keys
Foreign keys are essential to maintaining relationships in a relational database, but their implementation has several technical considerations, including referential integrity, constraints, performance implications, and indexing.
1. Referential Integrity Enforcement
Foreign keys enforce referential integrity by ensuring that the values in a foreign key column must match an existing primary key in the referenced table. This prevents orphaned records.
-
Parent Table: Holds the primary or unique key being referenced.
-
Child Table: Holds the foreign key linking back to the parent table.
Example:
ALTER TABLE students
ADD CONSTRAINT fk_class_student
FOREIGN KEY (class_id) REFERENCES classes(class_id)
ON DELETE CASCADE
ON UPDATE CASCADE;
2. Foreign Key Constraints
Foreign keys include constraints that control what happens when referenced data changes:
-
ON DELETE CASCADE: Deletes child records when a parent record is deleted.
-
ON DELETE SET NULL: Sets child foreign key values to NULL when the parent is deleted.
-
ON DELETE RESTRICT: Prevents deletion of a parent record if it has existing child records.
-
ON UPDATE CASCADE: Automatically updates foreign key values when the referenced key changes.
3. Indexing Foreign Keys
Foreign keys are not automatically indexed, but indexing them is crucial for optimizing performance in JOIN operations.
CREATE INDEX idx_students_class_id
ON students(class_id);
Without indexing, queries on large datasets can result in full table scans, impacting performance.
4. Self-Referencing Foreign Keys
A table can reference itself to represent hierarchical relationships, such as employee-supervisor structures.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(50),
manager_id INT,
FOREIGN KEY (manager_id) REFERENCES employees(employee_id)
);
5. Performance Considerations
Foreign keys provide integrity but can introduce performance overhead:
-
Insert/update overhead: The database must check foreign key constraints before inserting or updating records.
-
JOIN complexity: Queries involving multiple foreign key joins require optimization through indexing.
6. Foreign Keys in Distributed Databases
Many distributed databases (e.g., MongoDB, Cassandra) do not support foreign keys because enforcing referential integrity across distributed nodes is complex. Instead, referential integrity is managed at the application level.
Best Practices for Using Foreign Keys
Foreign keys are essential for maintaining data integrity but should be used carefully to avoid performance issues and complex dependencies. Here are key best practices:
1. Define Foreign Keys to Enforce Referential Integrity
Always define foreign keys to maintain valid relationships between tables. Without foreign keys, orphaned rows may occur, leading to data inconsistency.
2. Index Foreign Keys for Faster Queries
Foreign keys are not automatically indexed. Indexing foreign keys improves query performance, especially for JOIN operations.
CREATE INDEX idx_students_class_id ON students(class_id);
3. Choose the Right ON DELETE/ON UPDATE Strategy
-
ON DELETE CASCADE: Automatically delete child records when a parent record is deleted.
-
ON DELETE SET NULL: Removes the reference but keeps child records.
-
ON DELETE RESTRICT: Prevents deletion if child records exist.
4. Avoid Circular References
Ensure foreign keys do not create circular dependencies between tables, which can lead to data integrity issues and operational deadlocks.
5. Use Foreign Keys in Moderation for Performance
While essential for data consistency, excessive foreign keys can slow down transactions due to additional checks on inserts, updates, and deletes.
6. Consider Foreign Keys in Distributed Databases
Many NoSQL and distributed databases (e.g., MongoDB, Cassandra) do not support foreign keys natively. In such cases, enforce relationships at the application level.
7. Document Foreign Key Relationships
Maintaining clear documentation of table relationships helps developers understand database constraints and cascading behaviors.
Foreign Key FAQs
1. What happens if I try to delete a parent record with existing child records?
Without cascading actions, the database will throw an error, preventing deletion. ON DELETE CASCADE can be used to delete child records automatically.
2. Can a table have more than one foreign key?
Yes, a table can have multiple foreign keys, each referencing different parent tables.
3. Can foreign keys contain NULL values?
Yes, foreign keys can contain NULL values, meaning a child record may not have a corresponding parent record.
4. What are cascading actions?
Cascading actions propagate changes from the parent table to related child records, such as updating or deleting related rows automatically.
5. Can a foreign key reference a non-primary key column?
Yes, a foreign key can reference a column with a unique constraint, not necessarily a primary key.
6. What is a parent and child table in terms of foreign keys?
-
Parent Table: The table containing the referenced primary key.
-
Child Table: The table containing the foreign key that references the parent.
7. Can I remove a foreign key constraint?
Yes, you can remove a foreign key using:
ALTER TABLE students DROP FOREIGN KEY fk_students_class;
8. What is the impact of foreign keys on performance?
Foreign keys ensure data integrity but can add overhead on inserts, updates, and deletes due to constraint checks. Proper indexing can mitigate performance issues.
Conclusion
Foreign keys are essential in relational databases for maintaining data integrity, consistency, and structured relationships between tables. By implementing best practices such as indexing, cascading actions, and careful foreign key selection, databases can remain scalable, efficient, and well-structured.