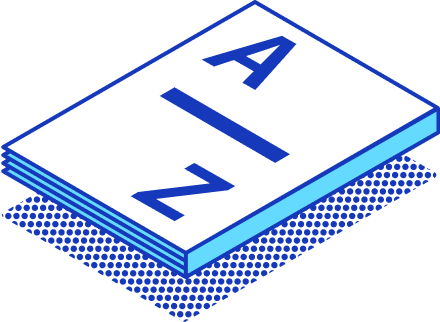
Lambda
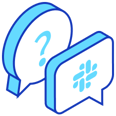
Join StarRocks Community on Slack
Connect on SlackWhat Is Lambda?
The term Lambda originates from the Greek letter λ. In mathematics and computer science, Lambda represents anonymous functions. These functions do not have a name. The concept of Lambda emerged in the 1930s through Alonzo Church's work on Lambda calculus. This mathematical framework laid the foundation for functional programming.
In programming, Lambda refers to small, anonymous functions. These functions can take any number of arguments but contain only one expression. Lambda functions allow developers to write concise code. The use of Lambda functions simplifies certain tasks and enhances code readability. Lambda expressions often appear in functional programming languages.
Lambda in Different Programming Languages
Lambda in Python
Python supports Lambda functions with a simple syntax. The lambda
keyword defines these functions. Python Lambda functions execute single expressions and return the result. Developers use Python Lambda functions for short, throwaway functions. Python's popularity in AWS Lambda stems from its simplicity and versatility.
Lambda in JavaScript
JavaScript uses Lambda functions, often called arrow functions. The =>
syntax defines these functions. JavaScript Lambda functions provide a more concise way to write function expressions. JavaScript developers use Lambda functions for callbacks and event handling. Lambda functions enhance code readability and reduce boilerplate in JavaScript.
Lambda in Java
Java introduced Lambda expressions in Java 8. These expressions enable functional programming features in Java. Java Lambda expressions simplify the implementation of single-method interfaces. Java developers use Lambda expressions to write cleaner and more efficient code. Lambda expressions improve Java's ability to handle concurrent programming tasks.
AWS Lambda: A Serverless Revolution
Understanding AWS Lambda
How AWS Lambda Works
AWS Lambda operates as a serverless compute service. Developers run code without managing servers. AWS handles the infrastructure, including scaling and maintenance. Lambda functions execute in response to events. These events trigger code execution automatically. AWS Lambda supports various programming languages like Python, JavaScript, and Java. Developers write code in these languages and deploy it on AWS Lambda. The platform manages the computing resources needed for execution.
Key Features of AWS Lambda
AWS Lambda offers several key features. The service provides automatic scaling. Code execution scales based on workload demand. AWS Lambda supports multiple languages, enhancing flexibility for developers. The platform integrates with other AWS services. Developers use Lambda with Amazon S3, DynamoDB, and more. AWS Lambda also includes monitoring and logging capabilities. These features help developers track performance and troubleshoot issues.
Benefits of Using AWS Lambda
Cost-Effectiveness
AWS Lambda provides cost-effective computing. Users pay only for the compute time consumed. No charges apply when the code is not running. This pricing model reduces operational costs. Businesses save money by avoiding server management expenses. AWS Lambda's cost-effectiveness appeals to startups and large enterprises alike.
Scalability and Flexibility
AWS Lambda excels in scalability and flexibility. The platform automatically adjusts to varying workloads. Developers deploy applications that handle sudden traffic spikes. AWS Lambda supports real-time data processing. Businesses process and analyze data efficiently. The platform's flexibility extends to diverse use cases. Developers build web applications, mobile backends, and IoT solutions. AWS Lambda empowers innovation across industries.
Key Concepts of Lambda
Anonymous Functions
Anonymous functions, often referred to as Lambda functions, play a crucial role in programming. These functions do not have a name, which makes them different from regular functions. Developers use anonymous functions to write concise code. The primary advantage lies in the ability to create small, single-use functions without the need for a formal definition.
In Python, you can define an anonymous function using the lambda
keyword. The syntax is straightforward and allows for quick implementation. For example, consider a function that adds two numbers:
add = lambda x, y: x + y
print(add(3, 5)) # Output: 8
JavaScript uses arrow functions to achieve similar functionality. The syntax involves the =>
operator, making it easy to understand and implement:
const add = (x, y) => x + y;
console.log(add(3, 5)); // Output: 8
Java introduced Lambda expressions in Java 8, enabling developers to write anonymous functions efficiently. This feature enhances Java's functional programming capabilities:
BinaryOperator<Integer> add = (x, y) -> x + y;
System.out.println(add.apply(3, 5)); // Output: 8
Anonymous functions simplify code by reducing the need for verbose function definitions. Developers often use these functions in scenarios where a simple operation is required, such as sorting or filtering data.
Lambda Expressions
Lambda expressions provide a compact way to represent anonymous functions. The syntax varies across programming languages but maintains the core principle of simplicity. In Python, the lambda
keyword precedes the argument list, followed by a colon and the expression:
square = lambda x: x * x
print(square(4)) # Output: 16
JavaScript employs the arrow function syntax, which omits the function
keyword and uses the =>
operator:
const square = x => x * x;
console.log(square(4)); // Output: 16
Java's Lambda expressions allow for concise representation of single-method interfaces. The syntax includes the parameter list, the arrow token ->
, and the body of the expression:
Function<Integer, Integer> square = x -> x * x;
System.out.println(square.apply(4)); // Output: 16
Lambda expressions enhance code readability and efficiency. Developers can implement complex operations with minimal code. The use of Lambda expressions aligns with the principles of lambda calculus, which emphasizes the abstraction of functions.
Case Study: Netflix Gains New Efficiencies Using AWS Lambda
Netflix leverages AWS Lambda to automate critical processes. The company uses Lambda functions to manage infrastructure and replace inefficient workflows. Automation includes media file encoding, backup validation, and AWS resource monitoring. These improvements reduce errors and save time, showcasing the power of Lambda in real-world applications.
Characteristics of Lambda
Advantages of Using Lambda
Conciseness
Lambda functions offer a concise way to write code. Developers can create small, single-use functions without extensive setup. This approach reduces the amount of code needed for simple tasks. The use of Lambda functions aligns with the principles of lambda calculus usage, which emphasizes simplicity and abstraction. By focusing on the core logic, developers can enhance productivity. The compact nature of Lambda functions makes them a gold standard in modern programming.
Flexibility
Lambda functions provide flexibility in various programming scenarios. Developers can use these functions across different programming languages. This versatility allows for seamless integration into existing projects. AWS Lambda enhances this flexibility by supporting multiple languages. Businesses can adapt quickly to changing requirements with Lambda's capabilities. The ability to handle diverse tasks makes Lambda a silver bullet in software development.
Limitations of Lambda
Readability Issues
Lambda functions can pose readability challenges. The concise nature may lead to confusion for those unfamiliar with the syntax. Developers must ensure that the function's purpose is clear. Overuse of Lambda functions can result in code that is difficult to understand. Maintaining a balance between conciseness and clarity is crucial. Clear documentation can mitigate readability issues associated with Lambda functions.
Debugging Challenges
Debugging Lambda functions can present unique challenges. The anonymous nature of these functions complicates error tracking. Developers may struggle to identify the source of a problem. AWS Lambda's serverless environment adds another layer of complexity. Effective debugging requires a good understanding of the function's context. Utilizing logging and monitoring tools can aid in troubleshooting. Despite these challenges, Lambda functions remain a valuable tool in a developer's arsenal.
Lambda functions serve as Closures, capturing variables from their surrounding scope. This feature enhances the power of Lambda functions in programming. The ability to maintain state within a function is a silver lining for developers. Closures enable more sophisticated operations without additional complexity. The combination of gold standard features and silver lining benefits makes Lambda functions indispensable.
Practical Examples of Lambda
Use Cases in Data Processing
Filtering and Mapping
AWS Lambda excels in data processing tasks, especially filtering and mapping. You can use Lambda to process real-time data streams from sources like IoT devices or social media. This capability allows businesses to analyze, transform, and filter data efficiently. AWS Lambda helps you gain insights into operations and make informed decisions quickly.
Consider a scenario where you need to filter data from a large dataset. Lambda functions can Add efficiency by processing only the necessary information. This approach saves time and resources. Developers often use Lambda for mapping tasks, where data is transformed into a new format. The flexibility of Lambda functions ensures seamless integration into existing workflows.
AWS Lambda's serverless architecture eliminates the need to manage servers. This feature saves developers time and reduces operational overhead. A developer once commented on the significant time saved by not managing servers or related tasks. The ability to focus solely on code enhances productivity and innovation.
Use Cases in Event Handling
Asynchronous Programming
AWS Lambda provides powerful solutions for event handling through asynchronous programming. Backend tasks should not delay responses to frontend requests. You can use Lambda to handle input-processing tasks efficiently. For example, Lambda can parse user input and send data to a database without affecting frontend performance.
Asynchronous programming with Lambda enables rapid response times. Developers can Add Lambda functions to clean up data and send it to applications that need it. This approach ensures smooth operation and enhances user experience. AWS Lambda supports real-time data processing, making it ideal for applications requiring immediate action.
The serverless nature of AWS Lambda allows for automatic scaling based on workload demand. Businesses can deploy applications that handle sudden traffic spikes without manual intervention. AWS Lambda's flexibility extends to diverse use cases, including web applications and mobile backends. The platform empowers developers to innovate and meet evolving demands.
Lambda vs Traditional Functions
Comparison of Syntax
Lambda functions and traditional functions differ significantly in syntax. Lambda functions offer a concise way to write code. Developers use Lambda functions for small, single-use tasks. The syntax for Lambda functions varies across programming languages.
In Python, you use the lambda
keyword to define a Lambda function. The syntax is simple and direct:
add = lambda x, y: x + y
print(add(3, 5)) # Output: 8
JavaScript employs arrow functions for Lambda expressions. The syntax uses the =>
operator:
const add = (x, y) => x + y;
console.log(add(3, 5)); // Output: 8
Java introduced Lambda expressions in Java 8. Java's syntax includes the parameter list, the arrow token ->
, and the expression body:
BinaryOperator<Integer> add = (x, y) -> x + y;
System.out.println(add.apply(3, 5)); // Output: 8
Traditional functions often require more verbose syntax. Developers must define a function name and specify parameters explicitly. This approach can lead to longer code blocks. Traditional functions may lack the flexibility of Lambda functions.
Performance Considerations
Lambda functions provide efficiency and speed advantages. Developers use Lambda functions to execute code without managing servers. AWS Lambda handles infrastructure tasks like scaling and maintenance. This serverless model reduces operational overhead.
Lambda functions excel in scenarios requiring rapid execution. Developers deploy Lambda functions for real-time data processing. The platform supports various programming languages, enhancing versatility. AWS Lambda's automatic scaling ensures applications handle sudden traffic spikes.
Traditional functions may not offer the same level of performance. Developers must manage server resources manually. This approach can lead to increased operational costs. Traditional functions may struggle with scalability and flexibility.
AWS Lambda empowers developers to innovate and grow. The platform provides tools for building scalable applications. Developers can focus on writing code without worrying about server management. AWS Lambda's flexibility and efficiency make it a valuable asset.
Future of Lambda in Programming
Trends and Developments
AWS Lambda continues to shape the future of programming with its serverless architecture. Developers increasingly adopt Lambda for its ability to streamline application deployment. The trend shows a shift toward cloud-native solutions. AWS Lambda reduces operational overhead and enhances scalability. Businesses can focus on innovation without server management concerns.
Lambda's adoption grows as more industries recognize its benefits. Real-time data processing and IoT device management are popular use cases. Organizations leverage Lambda to handle backend tasks efficiently. The serverless model provides predictable pricing and fast deployments. Developers find Lambda ideal for asynchronous processing.
Potential Innovations
Lambda's future includes exciting innovations in AI and machine learning. Developers explore ways to integrate Lambda with AI technologies. This integration promises to enhance data processing capabilities. Machine learning models can Improve decision-making processes. AWS Lambda supports these advancements with its flexible architecture.
Expert Testimony:
Developers can Improve applications by leveraging Lambda's capabilities. AI integration allows for smarter data analysis and automation. Machine learning models benefit from Lambda's scalability and efficiency. The combination of serverless computing and AI opens new possibilities. Businesses can Improve operations and gain competitive advantages.
AWS Lambda's role in programming will continue to evolve. Developers Follow trends and explore new use cases. The potential for innovation remains vast. Lambda empowers developers to Improve applications and meet future demands. The future of Lambda in programming looks promising and dynamic.
Conclusion
You explored Lambda's role in programming. Lambda functions offer concise solutions for developers. AWS Lambda enhances this with serverless computing. The platform provides cost-effective and scalable options. You learned about the importance of Lambda in modern software development. The future holds exciting possibilities for Lambda in cloud computing. Developers will continue to innovate with these tools. AWS Lambda empowers you to create efficient applications. The potential for growth remains vast. You can leverage Lambda's capabilities to meet evolving demands. The journey of Lambda in programming has only just begun.