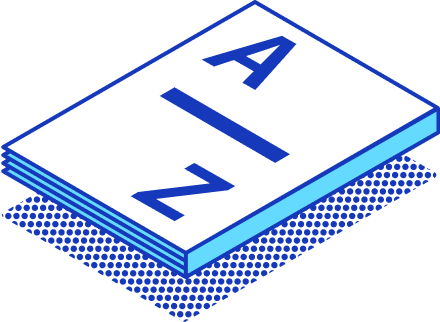
SQLite
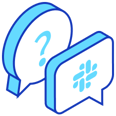
Join StarRocks Community on Slack
Connect on SlackWhat Is SQLite
Definition and Overview
SQLite serves as a compact, efficient database engine. The SQLite library operates without a server, making it ideal for many applications. Developers use SQLite to manage data in mobile apps, web browsers, and embedded systems. The database stores information in a single file, which simplifies data management. SQLite supports SQL, allowing users to perform complex queries easily. The SQLite Tutorial provides guidance for beginners to master the basics.
Key Features of SQLite
SQLite offers several key features that make it popular. The database is self-contained, requiring no additional software. Users can control data with SQL commands directly within their applications. SQLite supports multiple data types, including BLOBs, integers, and text. The engine ensures data integrity through ACID compliance. Changes in version updates often enhance performance and reliability. The SQLite Download page provides easy access to the latest version.
History and Development
The development of SQLite began in the year 2000. Richard Hipp created SQLite to offer a lightweight alternative to traditional databases. The SQLite library has evolved over time, with many changes in version releases. Developers worldwide contribute to its ongoing improvement. SQLite remains open-source, allowing anyone to use and modify the code. The database has become one of the most widely used RDBMS solutions globally.
How SQLite Differs from Other Databases
Comparison with MySQL
SQLite and MySQL both serve as relational database management systems. However, a significant difference lies in their architecture. SQLite operates without a server, while MySQL requires a server setup. SQLite is ideal for small to medium-sized applications. MySQL suits larger, enterprise-level projects with multiple users. SQLite uses a single file for storage, whereas MySQL employs a more complex structure. The default settings in SQLite prioritize simplicity and ease of use.
Comparison with PostgreSQL
PostgreSQL offers advanced features compared to SQLite. However, SQLite excels in scenarios requiring minimal configuration. PostgreSQL supports more complex data types and functions. SQLite focuses on simplicity and portability. The difference in language support also sets them apart. SQLite uses a straightforward SQL syntax, while PostgreSQL offers more extensive options. Both databases have their strengths, depending on the project's needs.
How Does SQLite Work
Architecture of SQLite
File-based Database System
SQLite uses a file-based database system. The entire database resides in a single file. This structure provides simplicity and portability. You can easily copy and share the database file. The file-based approach eliminates the need for a server. This makes SQLite ideal for applications with limited resources.
SQLite Engine Components
The SQLite engine consists of several key components. The SQL parser interprets SQL commands. The virtual machine executes these commands. The backend handles data storage and retrieval. Each component plays a role in managing data efficiently. The default settings in SQLite prioritize ease of use. Developers can control configurations using DBCONFIG options.
SQLite Data Types and Storage Classes
Supported Data Types
SQLite supports various data types. These include integers, text, and BLOBs. BLOB stands for Binary Large Object. You can store images and files as BLOBs. SQLite also supports real numbers and null values. The flexibility of data types enhances data management.
Storage Classes Explained
SQLite uses storage classes to define data storage. Five storage classes exist: NULL, INTEGER, REAL, TEXT, and BLOB. The storage class determines how data is stored. SQLite assigns storage classes based on the data type. Changes in version updates may affect storage behavior. Understanding storage classes helps you manage data effectively.
Advantages of Using SQLite
Lightweight and Portable
No Server Requirement
SQLite offers significant pros in terms of simplicity. The database does not require a server to operate. This feature allows you to control data easily without complex setups. Developers can integrate SQLite into applications with minimal effort. The default configuration suits many projects, reducing the need for extensive adjustments.
Cross-Platform Compatibility
SQLite provides excellent cross-platform compatibility. You can use it on various operating systems like Windows, macOS, and Linux. This flexibility allows developers to join different environments seamlessly. The database file remains consistent across platforms. This consistency ensures that data management stays efficient and straightforward.
Reliability and Performance
ACID Compliance
SQLite ensures data integrity through ACID compliance. ACID stands for Atomicity, Consistency, Isolation, and Durability. These properties guarantee reliable transactions. You can trust SQLite to maintain data accuracy even during unexpected events. The database engine handles transactions efficiently, providing robust control over data operations.
Efficient Memory Usage
SQLite excels in performance due to efficient memory usage. The engine is optimized to run smoothly on devices with limited resources. You will find SQLite faster than many alternatives like PostgreSQL and nearly as fast as MySQL. This speed makes SQLite ideal for applications where performance matters. Developers appreciate the ability to manage data swiftly without sacrificing reliability.
Common Use Cases for SQLite
Mobile Applications
Android and iOS Integration
Developers often choose SQLite for mobile applications. The database integrates seamlessly with both Android and iOS platforms. You can manage data directly on the device without needing a server. This integration allows developers to maintain control over data storage and retrieval. SQLite provides a reliable solution for handling local data in mobile apps. The default settings in SQLite simplify the process of embedding databases within applications. Developers can use SQL commands to interact with the database efficiently.
Offline Data Storage
SQLite excels in offline data storage for mobile applications. Users can access data without an internet connection. This feature enhances user experience by ensuring data availability at all times. Developers can store user preferences, app settings, and other data locally. SQLite supports various data types, including BLOBs, which allow for storing multimedia files. The database engine ensures data integrity even when the device is offline. You can trust SQLite to provide consistent performance in offline scenarios.
Desktop Applications
Embedded Systems
Embedded systems benefit from SQLite's lightweight nature. The database operates without a server, making it ideal for resource-constrained environments. Developers can embed SQLite into applications to manage data efficiently. The database's small footprint allows it to run smoothly on devices with limited memory. SQLite's default configuration suits many embedded systems without requiring extensive adjustments. You can control data operations using SQL commands within the application code.
Local Data Management
SQLite offers an excellent solution for local data management in desktop applications. The database stores all data in a single file, simplifying data handling. Developers can easily copy and share the database file across different systems. SQLite supports various data types, including integers, text, and BLOBs. This flexibility allows developers to manage diverse data sets effectively. The database engine provides robust control over data operations through ACID compliance. You can rely on SQLite to maintain data accuracy and reliability in local applications.
Getting Started with SQLite
Installation and Setup
Installing SQLite on Different Platforms
You can install SQLite on various platforms like Windows, macOS, and Linux. Each platform provides a straightforward installation process. On Windows, download the SQLite zip file from the official website. Extract the files to a directory of your choice. Add the directory to your system's PATH variable for easy access. On macOS, use the Homebrew package manager. Run the command brew install sqlite
in the terminal. For Linux, use the package manager specific to your distribution. For example, on Ubuntu, run sudo apt-get install sqlite3
. The installation process ensures that you have the necessary tools to interact with SQLite databases.
Basic Configuration
After installation, configure SQLite for your needs. The default settings work well for most applications. You can customize configurations using the command line interface. Open the terminal or command prompt and type sqlite3
to start the SQLite shell. Use SQL commands to set up your database environment. The .help
command lists available options and commands. You can create new databases and tables directly from the shell. The configuration process allows you to control how SQLite operates within your projects.
Basic SQLite Commands
Creating and Managing Tables
Creating tables in SQLite involves using SQL commands. Start by opening the SQLite shell. Use the CREATE TABLE
statement to define a new table. Specify the table name and columns with their data types. For example, CREATE TABLE students (id INTEGER, name TEXT, grade REAL)
. This command creates a table with three columns. Manage tables using additional SQL commands. The ALTER TABLE
statement modifies existing tables. The DROP TABLE
command deletes tables. These commands provide control over database structure and organization.
Performing CRUD Operations
CRUD operations form the basis of database interactions. CRUD stands for Create, Read, Update, and Delete. Use SQL commands to perform these operations in SQLite. To insert data, use the INSERT INTO
statement. For example, INSERT INTO students (id, name, grade) VALUES (1, 'John Doe', 85.5)
. Retrieve data with the SELECT
statement. For example, SELECT * FROM students
fetches all records. Update records using the UPDATE
statement. For instance, UPDATE students SET grade = 90 WHERE id = 1
. Delete records with the DELETE FROM
statement. For example, DELETE FROM students WHERE id = 1
. These operations allow you to manage data effectively within your SQLite database.
Exploring SQLite with Python
Python SQLite Module
Establishing a Connection
Python offers a built-in module to interact with SQLite databases. You can establish a connection using the sqlite3
module. This module allows you to connect to an existing database or create a new one. To start, import the sqlite3
module in your Python script. Use the connect()
function to open a connection to the database file. For example:
import sqlite3
connection = sqlite3.connect('example.db')
The connect()
function creates a new database file if it does not exist. The connection object provides access to execute SQL commands and manage data. Always close the connection after completing operations to free up resources.
Executing SQL Commands
Once connected, you can execute SQL commands using the connection object. The cursor()
method creates a cursor object to interact with the database. Use the execute()
method of the cursor to run SQL queries. For example, to create a table:
cursor = connection.cursor()
cursor.execute('CREATE TABLE students (id INTEGER, name TEXT, grade REAL)')
The execute()
method allows you to perform various SQL operations like inserting, updating, or deleting records. After executing commands, commit changes using the commit()
method of the connection object. This step ensures that all modifications are saved to the database file.
Handling Data with Python
Using Cursors and Transactions
Cursors play a crucial role in managing data within SQLite databases. The cursor object enables you to fetch data from query results. Use the fetchall()
method to retrieve all rows from a query result. For example:
cursor.execute('SELECT * FROM students')
rows = cursor.fetchall()
for row in rows:
print(row)
Transactions help maintain data integrity during multiple operations. Use the begin
statement to start a transaction. Commit the transaction with the commit()
method or roll back changes with the rollback()
method if errors occur.
Adapting Custom Python Types
SQLite supports various data types, including text, integers, and BLOBs. However, you might need to store custom Python types in the database. The sqlite3
module allows you to adapt these types for storage. Use the register_adapter()
function to define how Python types convert to SQLite-compatible formats. For example, to store a custom object:
class Student:
def __init__(self, name, grade):
self.name = name
self.grade = grade
def adapt_student(student):
return f"{student.name},{student.grade}"
sqlite3.register_adapter(Student, adapt_student)
This approach ensures that custom types integrate seamlessly with SQLite databases. The flexibility of adapting Python types enhances data management capabilities.
Conclusion
SQLite offers a robust solution for managing data efficiently. The database engine provides a lightweight and portable option for various applications. You can use SQLite to CREATE tables and manage data with ease. The Default settings ensure simplicity, while ACID compliance guarantees data integrity. Python types SQLite natively supports enhance data handling capabilities. Developers can adapt Python types to suit specific needs. The BLOB storage class allows for versatile data management. The added in version updates continue to improve performance. Control over SQL commands empowers users to customize their databases effectively.