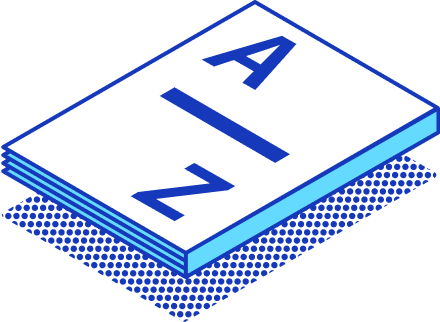
What is a Query Execution Plan and How Does it Work?
A query execution plan acts as a roadmap created by database management systems to show how your query will run. It helps you understand the steps the system takes to retrieve or modify data. By analyzing the execution plan, you can identify inefficiencies like missing indexes or costly join operations. For example, an e-commerce platform improved query performance by creating composite indexes and partitioning tables, reducing response times by 80%. Faster queries not only enhance database performance but also improve user experience, making applications smoother and more reliable.
Key Takeaways
-
A query execution plan is like a map. It shows how a database handles your question. Studying it helps find problems and make it faster.
-
Checking execution plans often can make databases work better. It helps find things like missing shortcuts or costly steps.
-
Tools like SQL Server Management Studio or MySQL Workbench show execution plans. These tools make it simple to see and fix your queries.
-
Adding the right shortcuts, called indexes, makes queries faster. Indexes help the database find data quickly without searching everything.
-
Breaking down hard queries and fixing the order can help. Try to filter data early to make later steps easier.
How Query Execution Plans Work
Purpose of a Query Execution Plan
A query execution plan serves as a blueprint for how a database processes your query. It breaks down the steps required to retrieve or modify data. By analyzing this plan, you can understand how the database accesses tables, applies filters, and joins data. This insight helps you identify inefficiencies and optimize query performance. For example, execution plans improve query performance by highlighting areas where indexes are missing or where operations are unnecessarily complex. Without this roadmap, you might struggle to pinpoint why a query runs slowly or consumes excessive resources.
How Databases Generate Query Execution Plans
Databases follow a structured process to create an execution plan. First, the database parses your SQL query into a parse tree, which represents its structure. Then, the query optimizer generates multiple candidate plans based on this tree. Each plan undergoes cost estimation, where the optimizer evaluates resource demands like CPU and disk usage. Finally, the optimizer selects the plan with the lowest cost and executes it.
To visualize an execution plan, you can use tools like SQL Server Management Studio. For an actual execution plan, write your query and press Ctrl+M. For an estimated plan, press Ctrl+L. These tools provide a graphical representation of the plan, making it easier to interpret.
Why Query Execution Plans Are Crucial for Performance
The impact of query execution plans on database performance is significant. They allow the optimizer to choose the most efficient way to execute a query, reducing execution time and resource usage. For instance, addressing inefficiencies in execution plans can cut query processing time by up to 75%. Execution plans improve query performance by minimizing disk accesses and ensuring the database uses the best strategy for data retrieval. Tools like MySQL EXPLAIN and SQL Server Management Studio help you analyze these plans and make informed decisions to enhance performance.
Components of a Query Execution Plan
Operators and Steps in Query Execution
Operators in an execution plan represent the actions the database performs to execute your query. Each operator corresponds to a specific task, such as retrieving data, applying filters, or joining tables. Understanding these operators helps you analyze how efficiently your query runs. Common operators include:
-
Result operator: Displays the final results of your query.
-
Nested loop operator: Handles different types of joins, such as inner and left outer joins.
-
Non-clustered Index Seek operator: Searches for specific data using an index, avoiding a full table scan.
-
Non-clustered Index Scan operator: Reads all rows from a non-clustered index.
-
Non-clustered Index Insert/Delete operators: Add or remove rows from a non-clustered index.
Each operator plays a role in breaking down your query into manageable steps. By reviewing these steps, you can identify areas where the database might be performing unnecessary work, such as scanning an entire table instead of using an index.
Cost Estimates and Their Significance
Cost estimates in an execution plan provide a way to measure the resource demands of each operation. These estimates help you prioritize optimizations by showing which parts of the query consume the most resources. For example, analyzing CPU costs can reveal performance bottlenecks, guiding you to focus on specific areas like index tuning.
Different databases calculate cost estimates in unique ways. In SQL Server, the estimated CPU cost is a relative measure rather than an exact time. Factors like data distribution, the presence of indices, and the complexity of joins influence these estimates. By understanding these costs, you can choose less resource-intensive query paths and improve overall performance.
Execution Order of Operations
The execution order in a query execution plan determines how the database processes your query. This order ensures that efficient operations, such as filtering and grouping, occur before more resource-intensive tasks like sorting. Early filtering reduces the size of the data set, making subsequent steps faster.
For example, if the database applies a filter after sorting, it wastes resources sorting unnecessary data. Reviewing the execution order helps you spot inefficiencies and ensure the database processes your query in the most logical sequence. A well-optimized execution order not only improves performance but also ensures accurate results.
Data Access Methods (e.g., Index Scans, Table Scans)
When a database processes your query, it uses specific methods to access the data. These methods, such as index scans and table scans, play a critical role in determining query performance. Understanding how these methods work helps you identify inefficiencies and optimize your queries.
Index Scans vs. Table Scans: Key Differences
Databases use index scans and table scans to retrieve data, but their performance impact varies:
-
Index Scans: These focus on reading fewer pages by leveraging an index. They work well when filtering conditions are applied, as they can eliminate unnecessary rows before accessing the data pages. However, if the index does not cover all columns in the query, additional lookups may be required.
-
Table Scans: These read all rows in a table, regardless of filtering conditions. While this method ensures complete data retrieval, it can be slower because it processes more data than necessary.
When an index includes all the columns needed for the query, the performance difference between an index scan and a table scan becomes negligible.
Common Data Access Methods in Query Execution Plans
Query execution plans rely on various data access methods to retrieve and process data efficiently. Here’s a breakdown of the most common methods and their impact:
Component |
Description |
---|---|
The order in which source tables are accessed can vary, affecting performance. |
|
Methods to Extract Data |
Different methods like index seeks or table scans are used based on the query requirements. |
Calculation and Filtering Methods |
Various methods are employed to compute, filter, aggregate, and sort data from the tables. |
SQL Server, for example, uses logical and physical execution plans to determine the best way to retrieve data. The logical plan outlines the sequence of operations, while the physical plan shows how these operations are implemented.
How Access Methods Affect Performance
Access methods like full table scans and index seeks directly influence query speed. Index utilization often leads to faster queries by narrowing down the data set early. Filtering and predicate evaluation further refine the results, reducing the workload for subsequent operations like sorting or joining.
By analyzing the data access methods in your query execution plan, you can pinpoint inefficiencies and make informed decisions to improve performance.
How to Generate and Interpret a Query Execution Plan
Generating SQL Execution Plans in Popular Databases
SQL Server
Creating an SQL execution plan in SQL Server is straightforward. To generate an actual execution plan:
-
Write your query and press
Ctrl+M
. -
Right-click in the query window and select "Display Actual Execution Plan".
-
Alternatively, click the "Display Actual Execution Plan" icon on the toolbar.
For an estimated execution plan:
-
Press
Ctrl+L
after writing your query. -
Right-click in the query window and select "Display Estimated Execution Plan".
-
Use the "Display Estimated Execution Plan" icon on the toolbar.
These steps allow you to visualize how SQL Server processes your query and identify potential bottlenecks.
MySQL
In MySQL, use the EXPLAIN
command to generate an SQL execution plan. For example:
EXPLAIN SELECT * FROM orders WHERE order_date > '2023-01-01';
This command provides insights into how the database retrieves data, including details about table scans, index usage, and join strategies.
PostgreSQL
PostgreSQL offers the EXPLAIN
command with the ANALYZE
option for detailed execution statistics. For example:
EXPLAIN ANALYZE SELECT * FROM customers WHERE city = 'New York';
This command not only shows the execution plan but also provides actual runtime metrics, helping you analyze query performance more effectively.
Interpreting Key Metrics in Execution Plans
Execution Time and Resource Usage
Execution plans reveal how much time and resources your query consumes. Look for metrics like CPU usage, memory allocation, and disk I/O. High resource usage often indicates inefficiencies. For instance, a full table scan may consume more resources than an index seek. By analyzing the generated plan, you can pinpoint areas where the database spends the most time and optimize accordingly.
Identifying Bottlenecks and Inefficiencies
Execution plans help you identify potential bottlenecks that slow down query performance. Common issues include:
-
Scans: Full table scans consume significant I/O and CPU resources. Use indexes to reduce their impact.
-
Sorts: Sorting operations can be resource-intensive. Matching indexes to the
ORDER BY
clause minimizes sorting costs. -
Joins: Hash joins and nested loops often require high memory and CPU. Optimize by indexing join columns and reducing table sizes.
Understanding these bottlenecks allows you to refine your queries and improve database efficiency.
Using Query Execution Plans to Optimize Performance
Identifying Common Issues in Execution Plans
Missing Indexes and Their Impact
Missing indexes often lead to slower query performance by increasing query cost. Without proper indexing, the database may resort to full table scans, which consume more resources. You can identify missing indexes in an execution plan through the 'MissingIndexes' element. This feature suggests potential indexes that could improve efficiency. Additionally, dynamic management views (DMVs) provide insights into missing indexes, helping you address this issue effectively.
Expensive Joins and How to Address Them
Execution plans reveal the types of joins used in your queries, such as nested loops or hash joins. These details help you identify expensive joins that consume excessive resources. For example, the plan shows whether tables are fully scanned or if indexes are utilized. It also highlights the cost of each join type. To optimize these joins, ensure that join columns are indexed and reduce the size of the data being joined. This approach minimizes resource usage and improves query performance.
Redundant Operations in Queries
Execution plans help you spot redundant operations, such as unnecessary joins or full table scans. Each node in the plan represents an operation with a cost estimate, showing how much it impacts performance. By analyzing these nodes, you can identify inefficiencies and eliminate redundant steps. For instance, removing unnecessary joins or simplifying filters can significantly enhance query execution.
Strategies for Query Optimization
Adding Indexes to Improve Query Speed
Indexes play a crucial role in optimizing queries. They allow the database to locate data quickly, reducing the need for full table scans. Use the suggestions in the execution plan to create indexes that align with your query's filtering and sorting requirements. For example, adding a composite index on frequently queried columns can dramatically improve query speed.
Rewriting Queries for Efficiency
Simplifying your queries can make them easier for the database to process. Break complex queries into smaller parts. Limit result sets by selecting only the necessary columns and rows. Optimize WHERE clauses by writing precise conditions and ordering filters logically. For instance, refactoring subqueries or restructuring joins can reduce processing time and improve overall efficiency.
Partitioning Data for Better Performance
Partitioning divides large tables into smaller, manageable segments. This approach improves resource utilization by distributing data across partitions. To benefit from partitioning, include the partition key in your query. For example, querying a specific partition reduces the data scanned, speeding up execution. However, ensure partitions are neither too large nor too small to maintain optimal performance.
Tools for Analyzing Query Execution Plans
Built-in Database Tools for Query Execution Analysis
SQL Server Management Studio
SQL Server Management Studio (SSMS) provides a powerful way to analyze an execution plan. After running your query, SSMS displays a visual representation of the operations involved. You can see details like costs, statistics, and the sequence of steps. This tool helps you identify inefficiencies, such as missing indexes or expensive joins, and optimize your queries effectively.
MySQL Workbench
MySQL Workbench uses the EXPLAIN
command to help you understand how your query executes. It shows table access types, possible indexes, and estimated row counts. This information allows you to pinpoint performance issues, such as unnecessary table scans, and make adjustments to improve query speed.
pgAdmin
PostgreSQL’s pgAdmin offers the EXPLAIN
command with an ANALYZE
option. This feature provides actual execution statistics alongside the execution plan. By using this tool, you can evaluate runtime performance and identify bottlenecks in your queries. It’s especially useful for fine-tuning complex queries.
Third-Party Tools for Advanced Analysis
Third-party tools provide advanced features for analyzing query execution plans. These tools often include graphical interfaces and optimization recommendations, making them ideal for in-depth performance tuning.
Feature |
Description |
---|---|
Query builder and profiler |
Helps you tune queries and investigate performance issues. |
Offers a visual representation of execution plans for easier understanding. |
|
Recommendations for optimization |
Provides insights and suggestions for improving query performance. |
Index manager |
Resolves index fragmentation to enhance database efficiency. |
SQL snippets |
Allows you to reuse code snippets for faster query writing. |
dbForge Studio for SQL Server
dbForge Studio simplifies query optimization with tools like a query builder, profiler, and index manager. Its graphical interface makes analyzing query execution plans intuitive. You can also use its SQL formatting and code navigation features to streamline your workflow.
SolarWinds Database Performance Analyzer
SolarWinds Database Performance Analyzer focuses on performance monitoring and optimization. It provides detailed execution plan analysis, highlighting inefficiencies and offering actionable recommendations. Its visual tools make it easier to understand complex query operations.
Query execution plans are vital for understanding and improving database performance. They help you identify inefficiencies like missing indexes or poor joins, enabling faster query response times. Regularly analyzing these plans ensures you can troubleshoot bottlenecks, optimize queries, and plan for scalability. To maintain efficient systems, focus on strategies like indexing, query rewriting, and reducing subqueries. Explore tools like SQL Server Management Studio or SolarWinds Database Performance Analyzer to simplify analysis. By adopting these practices, you can enhance database efficiency and ensure long-term reliability.
FAQ
What is the difference between an estimated and an actual execution plan?
An estimated execution plan predicts how the database will execute your query. It does not run the query. An actual execution plan shows the steps the database took after running the query. Use both to identify inefficiencies and optimize performance.
How can you identify missing indexes in an execution plan?
Execution plans often highlight missing indexes in a "MissingIndexes" section. This feature suggests indexes that could improve query performance. You can also use dynamic management views (DMVs) in databases like SQL Server to find missing indexes.
Why do some queries use table scans instead of index scans?
Table scans occur when no suitable index exists for the query. They also happen if the query retrieves most rows in the table. Adding indexes or rewriting the query can reduce table scans and improve performance.
How often should you review query execution plans?
You should review execution plans regularly, especially after schema changes, query updates, or performance issues. Frequent reviews help you catch inefficiencies early and maintain optimal database performance.
Can third-party tools replace built-in database tools for execution plan analysis?
Third-party tools complement built-in tools by offering advanced features like optimization recommendations and visual interfaces. They simplify complex analysis but do not replace the need to understand built-in tools for basic query tuning.