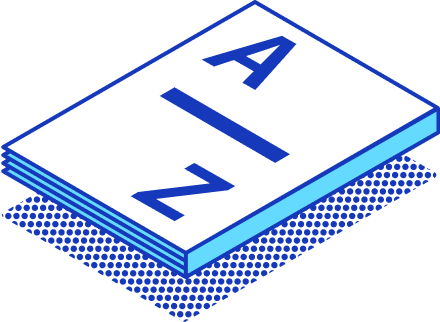
Data Serialization: What It Is and Why It’s Needed
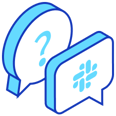
Join StarRocks Community on Slack
Connect on SlackWhat Is Data Serialization?
In the vast world of computing, data serialization is an essential process that enables data to move seamlessly between different systems, storage formats, and transmission protocols. This concept is not just a technical operation but a fundamental bridge connecting diverse components in modern software architectures.
Whether you are developing a distributed system, building APIs, or managing big data pipelines, serialization ensures that complex data structures remain accessible, consistent, and efficient. This guide takes an in-depth look at data serialization, from its core principles to its real-world applications and advanced techniques, all while providing practical examples and best practices.
Comparison: Serialization vs. Deserialization
Data serialization is the process of converting complex data structures or objects into a format that can be easily stored or transmitted. Conversely, deserialization involves reconstructing the original structure from this format.
For example, consider a nested object like a user profile containing strings, integers, and lists. Serialization converts this object into a JSON or binary format so it can be stored in a database or sent to a different system via a network.
Serialization vs. Deserialization
Aspect | Serialization | Deserialization |
---|---|---|
Definition | Converts data structures/objects into a storable or transmittable format. | Converts serialized data back into its original form. |
Direction | Original format → Encoded format | Encoded format → Original format |
Purpose | Prepares data for storage, transmission, or interoperability. | Reconstructs data for processing, use, or manipulation. |
Output/Input | Formats like JSON, XML, Protobuf, or binary streams. | Original data structure or object. |
Tools and Libraries | Libraries for JSON (json in Python, Gson in Java), Protobuf, Avro. |
Same libraries, used for decoding or parsing. |
Use Cases | Storing data in databases, transmitting over networks, logging. | Retrieving stored data, processing received data. |
Challenges | Efficient encoding, format selection, schema versioning, maintaining backward/forward compatibility. | Handling corrupted data, version mismatches, validating schema evolution across systems. |
Schema Evolution Challenge: Formats like Protobuf and Avro support backward and forward compatibility, but strict schema enforcement can sometimes limit flexibility in highly dynamic systems. Developers need to ensure schema changes (like adding or removing fields) are well-documented and versioned.
Use Cases for Serialization
Serialization is critical in scenarios where data needs to be stored or transmitted efficiently. Examples include:
Network Communication
Serialized data can be sent over a network as a compact, universal format, enabling communication between distributed systems or microservices.
Example: Sending Data in JSON
import json
data = {"name": "Alice", "age": 30}
serialized_data = json.dumps(data)
# Send serialized_data over the network
Data Storage
Data is serialized before being saved in databases or files. This ensures that the structure is preserved and easily retrievable.
Example: Storing Data in Protobuf
import example_pb2 # Generated Protobuf classes
user = example_pb2.User(name="Alice", age=30)
serialized_data = user.SerializeToString()
# Save serialized_data to a file
Logging and Auditing
Serialized data is often used in logs or audit trails to record system states or events.
Use Cases for Deserialization
Deserialization is necessary when data stored or received in serialized form needs to be processed or displayed.
Reconstructing Stored Data
When data is retrieved from storage (e.g., a database or file), deserialization is used to restore its original structure.
Example: Reading Protobuf Data
user = example_pb2.User()
with open("user_data.bin", "rb") as file:
user.ParseFromString(file.read())
print(user.name) # Alice
Processing API Responses
APIs often return serialized data, such as JSON, which the client deserializes to use programmatically.
Example: Parsing API Response
fetch("https://api.example.com/user")
.then(response => response.json())
.then(data => console.log(data.name)); // Alice
Inter-System Communication
Systems that exchange serialized data (e.g., IoT devices, message queues) must deserialize it for further processing.
Serialization and Deserialization in Action
Let’s examine a complete workflow where serialization and deserialization work together:
1. Serialization:
-
- A client application serializes a user object into JSON to send it to the server.
const user = { name: "Alice", age: 30 };
const serializedData = JSON.stringify(user);
console.log(serializedData); // '{"name":"Alice","age":30}'
2. Transmission:
- The serialized JSON is transmitted over HTTP or saved to a database.
3. Deserialization:
- The server deserializes the received JSON to reconstruct the original object.
import json
serialized_data = '{"name": "Alice", "age": 30}'
user = json.loads(serialized_data)
print(user["name"]) # Alice
Data serialization and deserialization are indispensable tools in modern computing, facilitating seamless data storage, transmission, and interoperability. By encoding complex data structures into portable formats, serialization ensures that systems can communicate effectively across diverse platforms and architectures. Deserialization complements this process by reconstructing data into usable forms, enabling applications to process, display, or store information efficiently.
Why Data Serialization Is Crucial
1. Bridging the Gap Between Systems
Modern applications often need to interact with other systems written in different programming languages, running on diverse architectures, and adhering to unique protocols. Serialization enables these systems to exchange data seamlessly.
1.1 Cross-Platform Compatibility
Serialization ensures data can be shared across platforms without being tied to a specific system or language. It converts data into a universal format that different systems can understand.
- Example 1: A Python server serializes data into JSON, which a JavaScript client can easily deserialize.
- Example 2: Protobuf provides efficient communication between applications written in languages like Java, C++, or Go.
Code Example: Python Server
import json
data = {"name": "Alice", "age": 30}
serialized = json.dumps(data)
# Transmit serialized data to client
Code Example: JavaScript Client
const serializedData = '{"name": "Alice", "age": 30}';
const data = JSON.parse(serializedData);
console.log(data.name); // Alice
Why it Matters: JSON and Protobuf bridge language and platform barriers, making them ideal for APIs, distributed systems, and microservices.
2. Enhancing Data Storage
Serialization transforms complex in-memory data structures into compact formats optimized for storage, ensuring data integrity and efficient retrieval.
2.1 Efficient Storage Solutions
Serialized data is often smaller than its in-memory representation, especially when using compact formats like Protobuf or Avro. These formats remove redundant metadata, significantly reducing storage requirements.
Example: Storing user profiles in a database:
{"users": [{"name": "Alice", "age": 30}, {"name": "Bob", "age": 25}]}
- Raw Memory: Includes additional overhead like pointers and data alignment.
- Serialized Format: Removes unnecessary overhead, optimizing storage.
2.2 Data Persistence
Serialization is critical for persisting data over time. It ensures that stored data can be retrieved and reused later without loss of structure or meaning.
Example: Saving Application State in Python
import pickle
state = {"session": "active", "last_page": "home"}
with open("app_state.pkl", "wb") as file:
pickle.dump(state, file)
# Deserialize the state
with open("app_state.pkl", "rb") as file:
restored_state = pickle.load(file)
print(restored_state) # Output: {'session': 'active', 'last_page': 'home'}
Why it Matters: Persistent storage through serialization is crucial for applications like session management, logs, and configuration files.
3. Facilitating Data Transmission
Serialization ensures that data can be transmitted efficiently and reliably between networked components.
3.1 Network Communication
Serialized data is often transmitted as a compact, streamable format like JSON, XML, or Protobuf, maintaining its structure and integrity during transmission.
Example: Real-Time Messaging
{"sender": "Alice", "message": "Hello, World!"}
The server deserializes this JSON to process the message and forward it to the recipient.
3.2 Real-Time Data Sharing
Serialization is essential for real-time applications like gaming, IoT, and live dashboards, where low latency is critical. Compact and fast serialization formats like Protobuf and MessagePack reduce latency by minimizing payload size.
Why it Matters: Serialization enables seamless data sharing, ensuring reliability in high-performance systems.
4. Optimizing Application Performance
Efficient serialization significantly impacts performance, especially in systems handling large data volumes or requiring high throughput.
4.1 Reducing Latency
Binary formats like Protobuf and MessagePack reduce the size of serialized data, speeding up transmission and parsing.
Example: JSON vs. Protobuf for the Same Data
- JSON:
{"name": "Alice", "age": 30}
→ 36 bytes - Protobuf: Encoded binary → ~15 bytes
Smaller payloads result in faster data transfer, especially over bandwidth-limited networks.
4.2 Enabling Scalability
Serialization techniques like batch processing and parallel serialization allow distributed systems to handle large datasets efficiently.
Why it Matters: Optimized serialization ensures applications remain scalable and responsive under heavy loads.
5. Supporting Data Integration
Serialization simplifies integration between heterogeneous systems, making it a backbone for modern software ecosystems.
5.1 API Communication
Serialization formats like JSON and XML provide standardized ways to structure data in REST and SOAP APIs, ensuring smooth interoperability between services.
Example: Fetching Data from an API
fetch("https://api.example.com/user/1")
.then((response) => response.json())
.then((data) => console.log(data.name)); // Alice
5.2 Big Data Pipelines
Serialization is critical for processing and transferring large datasets in big data ecosystems like Kafka, Spark, and Hadoop. Formats like Avro and Protobuf ensure high performance and schema consistency.
Why it Matters: Serialization enables seamless integration, supporting diverse tools and platforms in complex data workflows.
6. Enabling Data Recovery and Migration
Serialization is essential for disaster recovery, backups, and data migration, ensuring data consistency and compatibility across versions.
6.1 Data Recovery
Serialized data allows backups to be restored accurately. For example, serialized user sessions can be reloaded to resume operations after a system failure.
6.2 Schema Evolution
Formats like Avro and Protobuf support schema evolution, allowing backward-compatible updates to data structures.
Example: Adding a New Field in Protobuf
message User {
string name = 1;
optional int32 age = 2; // New field with backward compatibility
}
Why it Matters: Schema evolution prevents compatibility issues, enabling smooth updates and migrations.
How Serialization Empowers Developers
Serialization provides developers with powerful tools to handle data efficiently:
- Interoperability: Ensures smooth communication between systems in different languages.
- Efficiency: Reduces data size for storage and transmission.
- Consistency: Maintains structure and integrity across systems.
Real-World Scenario: Microservices Architecture In microservices, serialization ensures data flows seamlessly between services, regardless of their internal implementations.
Example: A microservice serializes user data in Protobuf for transmission to another service, which deserializes it to process requests efficiently.
Best Practices for Data Serialization
When working with data serialization, following best practices ensures that your processes are efficient, secure, and maintainable. Let’s break down these practices into actionable, detailed steps tailored to real-world scenarios.
Choose the Right Format
The choice of serialization format depends heavily on your application's requirements. Each format has trade-offs in terms of readability, efficiency, and flexibility.
-
JSON: Use for APIs, front-end interactions, and when human-readability is critical.
- Example Use Case: A REST API returning a user profile in JSON ensures easy debugging and integration with JavaScript clients.
{
"name": "Alice",
"age": 30
}
Protocol Buffers (Protobuf): Best for high-performance systems where efficiency and speed are priorities.
- Example Use Case: A microservices-based architecture where services need to exchange large amounts of structured data with minimal overhead.
message User {
string name = 1;
int32 age = 2;
}
XML: Ideal for scenarios requiring metadata, schemas, or hierarchical data.
- Example Use Case: Configuration files for enterprise systems where structure and metadata must be explicitly defined.
<User>
<Name>Alice</Name>
<Age>30</Age>
</User>
Pro Tip: When working with complex systems, consider the need for schema evolution. Formats like Protobuf and Avro support versioning, which ensures compatibility as data structures change.
Handle Errors Gracefully
Data corruption or invalid input can disrupt serialization and deserialization. Implement robust error handling to mitigate these risks.
- Deserialization Error Handling:
- Catch exceptions during deserialization and log detailed error messages.
- Provide fallbacks for partial or corrupted data.
Example in Python:
import json
serialized_data = '{"name": "Alice", "age": "thirty"}' # Invalid age value
try:
user = json.loads(serialized_data)
age = int(user["age"]) # This will raise a ValueError
except (json.JSONDecodeError, ValueError) as e:
print(f"Error during deserialization: {e}")
user = None
- Schema Validation: Use libraries or schema definitions (e.g., JSON Schema, Protobuf schema) to validate serialized data before deserialization.
Pro Tip: Always test deserialization against various edge cases, including missing fields, unexpected data types, and malformed payloads.
Optimize Performance
Efficient serialization minimizes latency and maximizes throughput, especially in large-scale systems.
-
Compact Binary Formats: Use binary formats like Protobuf, Avro, or MessagePack for reduced payload size and faster transmission. For example, a JSON object might occupy 100 bytes, while its Protobuf equivalent could be under 20 bytes.
-
Batch Processing: For high-throughput systems (e.g., data pipelines), serialize multiple records in a single operation to reduce overhead.
Example with Batch Serialization:
import json
data_batch = [{"name": "Alice", "age": 30}, {"name": "Bob", "age": 25}]
serialized_batch = json.dumps(data_batch)
print(serialized_batch)
Pro Tip: Measure serialization and deserialization performance using profiling tools to identify bottlenecks. Optimize by adjusting data formats or algorithms as needed.
Ensure Security
Serialized data can be a vector for attacks if not handled securely. Implement safeguards to protect against vulnerabilities.
-
Encrypt Sensitive Data: Use encryption protocols (e.g., AES, RSA) to secure serialized data before transmission or storage.
- Example: Encrypt a JSON payload before sending it over a network.
from cryptography.fernet import Fernet
key = Fernet.generate_key()
cipher = Fernet(key)
data = '{"name": "Alice", "age": 30}'
encrypted_data = cipher.encrypt(data.encode())
print(encrypted_data) # Encrypted byte string
-
Validate Input Data: Use schema validation to prevent malicious payloads from being injected during serialization.
Pro Tip: Avoid deserializing untrusted data unless you have implemented strict validation and sanitization measures.
5. Document Schema Changes
In schema-based serialization formats (e.g., Protobuf, Avro), documenting schema changes is critical for maintaining compatibility over time.
- Versioning: Introduce new fields as optional with default values to ensure older systems can still process updated schemas.
Example of Schema Evolution in Protobuf:
// Version 1
message User {
string name = 1;
}
// Version 2
message User {
string name = 1;
optional int32 age = 2; // New field with default compatibility
}
- Documentation: Maintain a changelog for schema updates to help developers understand the evolution and potential compatibility issues.
Pro Tip: Use tools like Avro’s Schema Registry to manage schema versions across distributed systems.
Advanced Topics in Data Serialization
As you delve deeper into data serialization, advanced topics like security and performance optimization become critical for effective data handling. These considerations ensure serialized data remains secure, reliable, and efficient for use in complex systems.
Security Concerns
-
Data Integrity
- Correct explanation. Using hash functions (e.g., SHA-256) or checksums is standard practice to verify data integrity.
- Suggestion: Mention that checksums (e.g., CRC32) are faster but less secure compared to cryptographic hashes (e.g., SHA-256).
-
Encryption Techniques
- The examples and encryption choices (AES for symmetric, RSA for asymmetric) are accurate.
- Suggestion: Highlight the use of TLS for transport-level encryption, as mentioned under "MITM Attacks." TLS already secures serialized data during transmission.
-
Mitigating MITM Attacks
- Correct and relevant. Emphasizing TLS and strong encryption aligns with best practices.
- Suggestion: Add a note on certificate pinning as an additional defense against MITM attacks.
Updated Best Practices:
- Always validate deserialized data to avoid injection attacks.
- Use encryption and secure transport layers to prevent unauthorized access.
- Employ schema validation to reject malformed or unexpected payloads.
Performance Optimization
-
Reducing Latency
- The focus on compact formats like Protobuf and Avro is accurate.
- Suggestion: Add a note on the impact of serialization libraries and compression techniques (e.g., GZIP) on reducing latency.
-
Improving Throughput
- Accurate and comprehensive. Batch serialization and parallel processing are well-known optimizations.
- Suggestion: Mention lazy deserialization, which avoids unnecessary parsing of unused data fields, as another way to optimize throughput in large datasets.
Emerging Concerns in Serialization
-
Deserialization Vulnerabilities
- Correct and complete. The guidance on validating data, sanitizing inputs, and restricting sources is solid.
- Suggestion: Mention specific examples of real-world deserialization attacks (e.g., Java's deserialization vulnerabilities) to highlight the severity of this issue.
-
Schema Evolution
- Accurate and well-explained. Adding optional fields and schema registries are key techniques.
- Suggestion: Include real-world tools like Confluent Schema Registry for managing Avro schemas, as they are widely used in modern data pipelines.
FAQs: Common Questions About Data Serialization
Q: What is the difference between serialization and marshaling?
Marshaling is a broader concept that encompasses preparing data for transport, which often includes serialization as one of its steps. While serialization focuses on encoding data into a storable or transmittable format (e.g., JSON or Protobuf), marshaling may also involve metadata management, protocol-specific preparation, or data alignment for transport layers.
- Example Contexts:
- In Remote Procedure Call (RPC) frameworks, marshaling involves both serializing the data and wrapping it with headers or additional information needed for communication.
- Marshaling might also include converting data into formats compatible with specific APIs or protocols.
Q: How do I handle schema evolution in serialization?
Schema evolution refers to maintaining compatibility between different versions of serialized data as data structures change. Formats like Avro and Protobuf are designed to support schema evolution, enabling systems to adapt over time without breaking existing functionality.
- Key Techniques:
- Optional Fields: New fields can be added without impacting older versions that do not use them.
- Default Values: Assigning default values ensures that missing fields are handled gracefully.
- Schema Registries: Tools like Avro’s Schema Registry help manage and validate schema changes dynamically.
- Backward and Forward Compatibility: Ensure that old versions of data can be read by new systems (backward compatibility) and vice versa (forward compatibility).
Q: Can serialization handle recursive structures like graphs?
Yes, some serialization formats and libraries can handle recursive structures such as graphs or trees. However, this often requires additional considerations to avoid issues like infinite loops or excessive memory usage.
-
Techniques for Handling Recursive Structures:
- Reference Preservation: Some libraries, like Python's
pickle
, support preserving references to the same object within a structure to avoid duplicating data. - Cycle Detection: Ensure the library or format can detect and handle cycles in graphs to prevent infinite loops during serialization.
- Flattening Structures: For formats like JSON, recursive structures may need to be flattened or converted into a hierarchical representation.
- Reference Preservation: Some libraries, like Python's
-
Limitations: Not all formats (e.g., Protobuf) inherently support recursive references, and additional logic may be required to handle complex relationships in data structures.
Q: What are the trade-offs between text-based and binary serialization formats?
Text-based formats like JSON and XML are human-readable, easy to debug, and widely supported, but they tend to be more verbose and slower to parse. Binary formats like Protobuf and Avro are more compact and faster but require specific tools for debugging and may lack readability.
- When to Choose Text-Based Formats: Use in scenarios where readability, ease of debugging, or widespread tool support is important (e.g., APIs, configuration files).
- When to Choose Binary Formats: Use in high-performance applications requiring minimal overhead and faster processing (e.g., IoT devices, large-scale data pipelines).
Q: How do I ensure security during serialization and deserialization?
Serialization can introduce security vulnerabilities, such as deserialization attacks or data leaks, if not handled carefully. Follow these best practices:
- Validate Input Data: Always validate incoming serialized data using schemas or strict type checks.
- Restrict Deserialization: Only deserialize data from trusted sources to prevent malicious payloads.
- Encrypt Sensitive Data: Use encryption protocols like AES or RSA to secure serialized data during storage or transmission.
- Sanitize Data: Ensure that deserialized data is free from injection attacks by implementing sanitization processes.
Q: What are the performance considerations in serialization?
Serialization performance depends on the format, data size, and system requirements. Key considerations include:
- Format Selection: Compact formats like Protobuf and Avro optimize for speed and storage, while JSON offers ease of use at the cost of verbosity.
- Batch Processing: Serialize multiple objects at once to reduce I/O overhead.
- Parallelization: Leverage parallel processing to handle large datasets more efficiently.
Latency vs. Throughput: Prioritize low-latency formats for real-time applications and high-throughput formats for bulk data processing.
Conclusion
Data serialization is a cornerstone of modern software development, enabling seamless data storage, transmission, and integration across diverse systems. By understanding advanced concepts like security measures and performance optimizations, developers can ensure their applications are robust, efficient, and secure. Best practices—such as validating input data, encrypting sensitive information, and optimizing throughput—help mitigate common challenges in serialization. As technologies like IoT and AI drive the need for scalable and interoperable systems, mastering serialization remains critical for building adaptable, high-performance applications.