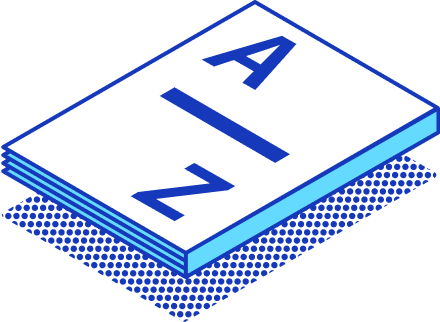
Apache Kafka
What is Apache Kafka?
Apache Kafka is an open-source distributed event streaming platform. It was developed by LinkedIn and later open-sourced in 2011. The platform handles real-time data feeds with high throughput, low latency, and fault tolerance. Kafka's architecture comprises two main layers: the storage layer and the compute layer. These layers ensure seamless data flow and processing.
Kafka uses a Publish and Subscribe model to read and write streams of records. The system is built with partitioning and replication features, enhancing its scalability and reliability. Kafka has become the de facto standard for data streaming, used by thousands of organizations worldwide.
Core concepts (topics, producers, consumers, brokers)
Kafka's core concepts include topics, producers, consumers, and brokers.
-
Topics: Topics are categories where records are stored and published. Each topic is divided into partitions, which helps in parallel processing and scalability.
-
Producers: Producers are applications that publish data to topics. They send records to specific topics based on the application's requirements.
-
Consumers: Consumers are applications that subscribe to topics to read and process records. Consumers can read data from multiple topics and partitions.
-
Brokers: Brokers are servers that store data and serve client requests. A Kafka cluster consists of multiple brokers, ensuring data distribution and fault tolerance.
Key Features
Scalability
Kafka's architecture supports horizontal scaling. Users can add more brokers to a Kafka cluster to handle increased data loads. Partitioning allows data to be distributed across multiple brokers. This ensures efficient data processing and storage.
Fault tolerance
Kafka provides fault tolerance through data replication. Each partition has multiple replicas stored on different brokers. If a broker fails, another broker with a replica takes over. This ensures data availability and reliability.
High throughput
Kafka is optimized for high throughput. The platform can handle trillions of events per day. Kafka's design minimizes latency, enabling real-time data processing. The combination of partitioning, replication, and efficient storage mechanisms contributes to its high performance.
Kafka Architecture
Components
Producers
Producers are the applications responsible for publishing data to Apache Kafka topics. Each producer sends records to specific topics based on the application's requirements. Producers can handle high volumes of data and ensure efficient data transmission. The Producer API allows developers to integrate their applications seamlessly with Kafka's storage layer.
Consumers
Consumers are applications that subscribe to Kafka topics to read and process records. Each consumer reads data from multiple topics and partitions. The Consumer API facilitates this process, enabling applications to act on new events as they occur. Consumers play a crucial role in real-time data processing and analytics.
Brokers
Brokers are the servers that store data and serve client requests. A Kafka cluster consists of multiple brokers, ensuring data distribution and fault tolerance. Each broker manages one or more partitions of a topic. Brokers handle data replication to ensure reliability and availability. The distributed nature of brokers allows Kafka to scale horizontally.
Zookeeper
Zookeeper is a centralized service for maintaining configuration information and providing distributed synchronization. Apache Kafka uses Zookeeper to manage and coordinate brokers. Zookeeper ensures that brokers are aware of each other and maintain the overall health of the Kafka cluster. Zookeeper also handles leader election for partitions, ensuring data consistency.
Data Flow
How data moves through Apache Kafka
Data movement in Apache Kafka follows a structured path. Producers publish records to topics, which are divided into partitions. Each partition stores records in an ordered sequence. Brokers manage these partitions and ensure data distribution across the cluster. Consumers subscribe to topics and read records from partitions. This flow enables real-time data processing and analytics.
Partitioning and replication
Partitioning is a key feature of Apache Kafka that enhances scalability and parallel processing. Each topic is divided into partitions, allowing data to be distributed across multiple brokers. This distribution ensures efficient data handling and storage. Replication provides fault tolerance by creating multiple copies of each partition. If a broker fails, another broker with a replica takes over, ensuring data availability and reliability.
Benefits of Using Apache Kafka
Real-time Data Processing
Stream processing capabilities
Apache Kafka excels in real-time data processing. The platform's architecture supports continuous data ingestion and analysis. Financial institutions use Kafka to process market data feeds instantly. Retailers analyze customer behavior in real-time to optimize marketing strategies. Online retailers deploy machine learning models for personalized recommendations. Kafka's stream processing capabilities enable businesses to react to events as they occur, enhancing decision-making and operational efficiency.
Reliability and Durability
Data retention policies
Kafka ensures data reliability and durability through robust data retention policies. Each record in Kafka can be stored for a configurable period, allowing for historical data analysis. Companies like Walmart and Lowe’s leverage Kafka to manage inventory and supply-chain data. Kafka's ability to retain data over time provides a reliable source for auditing and compliance. The platform's fault-tolerant design ensures data availability even during system failures.
Scalability
Horizontal scaling
Kafka's architecture supports horizontal scaling, making it ideal for handling large volumes of data. Users can add more brokers to a Kafka cluster to accommodate growing data needs. Partitioning allows data to be distributed across multiple brokers, ensuring efficient processing. Ecommerce giants like Domino’s and Bosch use Kafka for real-time analytics and fraud protection. Kafka's scalability enables businesses to expand their data infrastructure seamlessly, supporting growth and innovation.
Common Use Cases
Event Sourcing
Explanation and examples
Event sourcing involves storing the state of a system as a sequence of events. Each event represents a change in the system's state. Apache Kafka excels in this domain due to its ability to handle high-throughput data streams. Kafka records each event in an immutable log, ensuring data integrity.
For example, financial institutions use Kafka for transaction processing. Each transaction gets stored as an event, allowing for accurate auditing and reconciliation. Retail companies implement event sourcing to track inventory changes. Each sale or restock event updates the inventory state, providing real-time visibility.
Log Aggregation
Explanation and examples
Log aggregation consolidates logs from various services and systems into a central repository. Kafka serves as an efficient solution for this purpose. Companies like LinkedIn and Twitter use Kafka to aggregate logs, enabling real-time analysis and monitoring.
Kafka collects logs from multiple sources and publishes them to a central topic. Consumers then read these logs for further processing. This setup allows for quick identification of issues and performance bottlenecks. For instance, a microservices architecture benefits greatly from log aggregation. Each microservice sends logs to Kafka, where they get analyzed for errors and performance metrics.
Real-time Analytics
Explanation and examples
Real-time analytics involves processing data as it arrives to generate immediate insights. Kafka's stream processing capabilities make it ideal for this use case. Businesses leverage Kafka to gain actionable insights without delay.
E-commerce platforms use Kafka for real-time customer behavior analysis. Data from user interactions gets processed instantly to optimize marketing strategies. Financial firms employ Kafka to monitor market trends and execute trades based on real-time data. This capability enhances decision-making and operational efficiency.
Getting Started with Apache Kafka
Installation
System requirements
To install Apache Kafka, ensure the system meets the following requirements:
-
Java: Install Java 8 or later. Kafka relies on the Java Runtime Environment (JRE) for execution.
-
Zookeeper: Kafka uses Zookeeper for distributed coordination. Ensure Zookeeper is installed and running.
-
Operating System: Kafka supports various operating systems, including Linux, macOS, and Windows.
-
Memory and Storage: Allocate sufficient memory and storage based on the expected data volume and workload.
Step-by-step installation guide
Follow these steps to install Apache Kafka on a local system:
-
Download Kafka: Obtain the latest Kafka release from the official Apache Kafka website.
-
Extract the tar file: Use the terminal to extract the downloaded tar file.
tar -xzf kafka_2.13-2.8.0.tgz
cd kafka_2.13-2.8.0 -
Start Zookeeper: Run the following command to start Zookeeper.
bin/zookeeper-server-start.sh config/zookeeper.properties
-
Start Kafka server: Launch the Kafka server using the command below.
bin/kafka-server-start.sh config/server.properties
-
Verify installation: Check the Kafka logs to ensure the server started successfully.
Basic Operations
Creating topics
Creating topics in Apache Kafka involves the following steps:
-
Open terminal: Navigate to the Kafka installation directory.
-
Run topic creation command: Use the following command to create a new topic named
test-topic
.bin/kafka-topics.sh --create --topic test-topic --bootstrap-server localhost:9092 --partitions 1 --replication-factor 1
-
Verify topic creation: List all topics to confirm the creation of
test-topic
.bin/kafka-topics.sh --list --bootstrap-server localhost:9092
Producing and consuming messages
Producing and consuming messages in Apache Kafka involves the following steps:
-
Start a producer: Use the terminal to start a Kafka producer.
bin/kafka-console-producer.sh --topic test-topic --bootstrap-server localhost:9092
Type messages into the terminal to send them to the
test-topic
. -
Start a consumer: Open another terminal window and start a Kafka consumer.
bin/kafka-console-consumer.sh --topic test-topic --from-beginning --bootstrap-server localhost:9092
The consumer will display the messages sent by the producer.
By following these steps, users can set up Apache Kafka and perform basic operations like creating topics and producing/consuming messages. This foundational knowledge enables further exploration of Kafka's advanced features and capabilities.
Related Technologies
Kafka Connect
Overview and use cases
Kafka Connect serves as a robust tool for integrating Apache Kafka with various data sources and sinks. This framework simplifies the process of streaming data between Kafka and other systems. Kafka Connect supports both source connectors, which pull data from external systems into Kafka, and sink connectors, which push data from Kafka to external systems.
Use Cases:
-
Database Integration: Companies use Kafka Connect to stream data from databases like MySQL or PostgreSQL into Kafka topics. This enables real-time analytics and monitoring.
-
Data Warehousing: Organizations leverage Kafka Connect to load data from Kafka into data warehouses such as Amazon Redshift or Google BigQuery. This facilitates large-scale data analysis.
-
Log Aggregation: Kafka Connect aggregates logs from various services into a central Kafka topic. This setup allows for efficient log monitoring and troubleshooting.
Kafka Streams
Overview and use cases
Kafka Streams is a client library for building applications and microservices that process data stored in Kafka. This library provides high-level abstractions for stream processing, including transformations, aggregations, and joins.
Use Cases:
-
Real-time Analytics: Businesses use Kafka Streams to perform real-time data analysis. For example, e-commerce platforms analyze customer interactions to optimize marketing strategies.
-
Event-driven Applications: Kafka Streams powers event-driven architectures by processing streams of events in real-time. Financial institutions use this capability for fraud detection and transaction monitoring.
-
Data Enrichment: Companies enrich incoming data streams by joining them with reference data stored in Kafka. This process enhances the value of the data before it reaches downstream systems.
Comparison with Other Messaging Systems
RabbitMQ
RabbitMQ is a widely-used message broker known for its simplicity and flexibility. It excels in scenarios requiring complex routing and message delivery guarantees.
Key Differences:
-
Throughput: Kafka outperforms RabbitMQ in throughput, writing data up to 15 times faster.
-
Use Case: RabbitMQ suits applications needing advanced message routing and flexible configurations.
-
Scalability: Kafka offers better scalability due to its partitioned log model, making it ideal for large-scale data streaming.
Apache Pulsar
Apache Pulsar is a modern distributed messaging system designed for high performance and scalability. Pulsar combines features of both traditional message brokers and log-based systems.
Key Differences:
-
Latency: Kafka, in its default configuration, shows lower latency compared to Pulsar in most benchmarks.
-
Architecture: Pulsar uses a multi-layer architecture with separate serving and storage layers, providing flexibility in deployment.
-
Performance: Kafka generally provides higher throughput and faster message processing than Pulsar.
Choosing between Kafka, RabbitMQ, and Pulsar depends on specific requirements. Kafka excels in high-throughput data streaming. RabbitMQ offers ease of use and flexibility. Pulsar provides a blend of both worlds with a modern twist.
Conclusion
Apache Kafka offers a robust solution for real-time data processing. The platform's architecture ensures scalability, fault tolerance, and high throughput. Kafka's core components, such as producers, consumers, brokers, and Zookeeper, work together to manage data efficiently.
Exploring Kafka further can unlock new capabilities for handling streaming data. Beginners can start with resources like Tim Berglund's YouTube videos, which provide a comprehensive introduction to Kafka concepts and use cases.
For additional learning, consider the following resources:
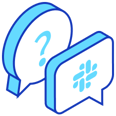
Join StarRocks Community on Slack
Connect on Slack