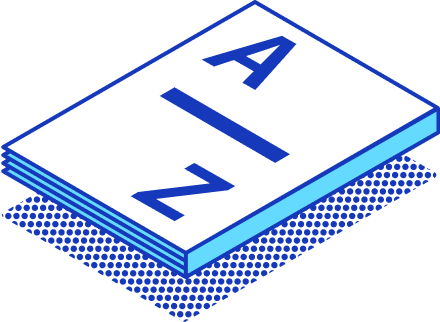
Consistent Hashing
What is Consistent Hashing?
Consistent Hashing is a technique used in distributed systems to distribute keys uniformly across a cluster of nodes. This method ensures minimal data movement when nodes are added or removed. The primary goal of Consistent Hashing is to maintain a balanced load across server nodes. This technique uses a virtual ring structure known as a hash ring. Server nodes are placed at random locations on the ring, allowing for seamless load balancing.
The concept of Consistent Hashing originated in the late 1990s. Researchers aimed to address the inefficiencies of traditional hashing methods. Early implementations focused on web caching and distributed databases. Over time, Consistent Hashing became a crucial component in various distributed systems. Modern applications include Amazon's DynamoDB and Apache Cassandra.
The Problem of Data Distribution
Challenges in Distributed Systems
Distributed systems face significant challenges in data distribution. Ensuring even distribution of data across nodes is critical. Uneven distribution can lead to overloaded nodes and degraded performance. Traditional hashing methods often fail to handle dynamic environments effectively. Changes in the number of nodes require rehashing of all keys, causing significant data movement and system disruption.
Traditional Hashing vs. Consistent Hashing
Traditional hashing methods use a simple modulo operation to distribute keys. This approach works well in static environments but struggles with dynamic changes. Consistent Hashing offers a solution by minimizing key relocation when nodes change. Instead of rehashing all keys, only a portion of the keys need redistribution. This optimization reduces workload and maintains consistent performance.
How Consistent Hashing Works
Hash Functions and Hash Rings
Consistent Hashing relies on hash functions to map keys to positions on a hash ring. A hash ring is a circular space where both keys and nodes are assigned positions. Each node is responsible for the keys falling between its position and the position of the previous node. This structure allows for efficient data distribution and load balancing.
Virtual Nodes and Their Role
Virtual nodes play a crucial role in Consistent Hashing. Each physical node is represented by multiple virtual nodes on the hash ring. This approach enhances load balancing by distributing the load more evenly across physical nodes. Virtual nodes also improve fault tolerance by ensuring that the failure of a single physical node does not disrupt the entire system.
Data Distribution Mechanism
The data distribution mechanism in Consistent Hashing involves mapping keys to nodes based on their positions on the hash ring. When a key needs to be stored or retrieved, the system calculates its hash value and finds the corresponding position on the ring. The nearest node in a clockwise direction from this position is responsible for the key. This process ensures efficient and balanced data distribution.
Advantages and Disadvantages
Benefits of Consistent Hashing
Scalability
Consistent Hashing enhances scalability in distributed systems. The technique allows easy addition or removal of nodes without extensive reconfiguration. When a new node joins, only a fraction of the keys need remapping. This minimizes disruptions and maintains system stability. Traditional hashing methods lack this adaptability. Consistent Hashing ensures efficient scaling even in dynamic environments.
Load Balancing
Load balancing improves significantly with Consistent Hashing. The method distributes keys uniformly across nodes. This prevents any single node from becoming overloaded. Virtual nodes further enhance load balancing by distributing the load more evenly. Efficient load balancing results in better system performance and reliability. Traditional hashing methods often struggle to achieve this level of balance.
Fault Tolerance
Fault tolerance is a critical benefit of Consistent Hashing. The technique ensures minimal data movement when nodes fail. Virtual nodes play a crucial role in maintaining fault tolerance. Each physical node has multiple virtual representations on the hash ring. If a node fails, other nodes can quickly take over its responsibilities. This mechanism ensures continuous operation and data availability.
Limitations and Challenges
Complexity in Implementation
Implementing Consistent Hashing involves complexity. The technique requires careful design and configuration. Setting up the hash ring and managing virtual nodes demand expertise. Developers must ensure that hash functions distribute keys uniformly. Incorrect implementation can lead to imbalanced loads and degraded performance. Traditional hashing methods are simpler but less efficient.
Potential Performance Overheads
Consistent Hashing may introduce performance overheads. The system needs to calculate hash values for keys and nodes. Managing virtual nodes adds additional computational requirements. These overheads can impact system performance, especially in large-scale environments. Developers must optimize the implementation to minimize these effects. Traditional hashing methods have lower overheads but lack efficiency.
Implementation Steps and Examples
Basic Implementation
Setting Up the Hash Ring
Setting up the hash ring forms the foundation of Consistent Hashing. The process begins by selecting a suitable hash function. This function maps both nodes and keys to positions on the hash ring. Each node receives multiple virtual node representations to ensure even load distribution. The system places these virtual nodes at random positions on the ring. This setup allows for efficient data distribution and load balancing.
Adding and Removing Nodes
Adding and removing nodes in Consistent Hashing involves minimal disruption. When adding a node, the system assigns new virtual nodes to positions on the hash ring. Only a fraction of the keys need remapping to the new node. This minimizes data movement and maintains system stability. Removing a node follows a similar process. The system reassigns the keys of the removed node to the nearest nodes on the ring. This ensures continuous operation and data availability.
Advanced Techniques
Optimizing for Load Balancing
Optimizing for load balancing in Consistent Hashing involves fine-tuning the distribution of virtual nodes. The system can adjust the number of virtual nodes per physical node based on load requirements. More virtual nodes result in finer-grained load distribution. Monitoring and adjusting the hash function can also enhance load balancing. Ensuring uniform key distribution across the hash ring prevents any single node from becoming overloaded.
Handling Node Failures
Handling node failures in Consistent Hashing relies on the redundancy provided by virtual nodes. When a node fails, its virtual nodes become inactive. The system quickly reassigns the keys of the failed node to the nearest active nodes. This process ensures minimal data loss and continuous operation. Implementing replication strategies further enhances fault tolerance. Replicating data across multiple nodes ensures availability even during node failures.
Code Examples
Example in Python
import hashlib
class ConsistentHashing:
def __init__(self, num_replicas=3):
self.num_replicas = num_replicas
self.hash_ring = {}
def _hash(self, key):
return int(hashlib.md5(key.encode('utf-8')).hexdigest(), 16)
def add_node(self, node):
for i in range(self.num_replicas):
hash_key = self._hash(f"{node}-{i}")
self.hash_ring[hash_key] = node
def remove_node(self, node):
for i in range(self.num_replicas):
hash_key = self._hash(f"{node}-{i}")
del self.hash_ring[hash_key]
def get_node(self, key):
hash_key = self._hash(key)
sorted_keys = sorted(self.hash_ring.keys())
for k in sorted_keys:
if hash_key <= k:
return self.hash_ring[k]
return self.hash_ring[sorted_keys[0]]
# Usage example
ch = ConsistentHashing()
ch.add_node("Node1")
ch.add_node("Node2")
print(ch.get_node("my_key"))
Example in Java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.SortedMap;
import java.util.TreeMap;
public class ConsistentHashing {
private final int numReplicas;
private final SortedMap<Integer, String> hashRing = new TreeMap<>();
public ConsistentHashing(int numReplicas) {
this.numReplicas = numReplicas;
}
private int hash(String key) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] digest = md.digest(key.getBytes());
return ((digest[0] & 0xFF) << 24) | ((digest[1] & 0xFF) << 16) | ((digest[2] & 0xFF) << 8) | (digest[3] & 0xFF);
}
public void addNode(String node) throws NoSuchAlgorithmException {
for (int i = 0; i < numReplicas; i++) {
int hashKey = hash(node + "-" + i);
hashRing.put(hashKey, node);
}
}
public void removeNode(String node) throws NoSuchAlgorithmException {
for (int i = 0; i < numReplicas; i++) {
int hashKey = hash(node + "-" + i);
hashRing.remove(hashKey);
}
}
public String getNode(String key) throws NoSuchAlgorithmException {
if (hashRing.isEmpty()) {
return null;
}
int hashKey = hash(key);
if (!hashRing.containsKey(hashKey)) {
SortedMap<Integer, String> tailMap = hashRing.tailMap(hashKey);
hashKey = tailMap.isEmpty() ? hashRing.firstKey() : tailMap.firstKey();
}
return hashRing.get(hashKey);
}
public static void main(String[] args) throws NoSuchAlgorithmException {
ConsistentHashing ch = new ConsistentHashing(3);
ch.addNode("Node1");
ch.addNode("Node2");
System.out.println(ch.getNode("my_key"));
}
}
Practical Considerations
Handling Node Additions and Removals
Minimizing Data Movement
Consistent Hashing minimizes data movement when adding or removing nodes. Traditional hashing methods require rehashing all keys, causing significant disruptions. Consistent Hashing only redistributes a small portion of the keys. This approach ensures efficient scaling and maintains system stability. The hash ring structure allows seamless integration of new nodes. Existing nodes continue to function without extensive reconfiguration.
Ensuring Consistency
Ensuring consistency in Consistent Hashing involves maintaining accurate key-to-node mappings. The system must update the hash ring promptly when nodes change. Virtual nodes play a crucial role in this process. Each physical node has multiple virtual representations on the hash ring. This redundancy ensures that key assignments remain consistent. The system can quickly adapt to changes, maintaining data integrity and availability.
Load Balancing Strategies
Dynamic Load Adjustment
Dynamic load adjustment optimizes performance in Consistent Hashing. The system monitors node loads and adjusts virtual node distributions accordingly. Adding more virtual nodes to heavily loaded physical nodes can balance the load. This fine-tuning process prevents any single node from becoming overloaded. Consistent Hashing's flexibility allows for real-time adjustments, enhancing overall system efficiency.
Monitoring and Metrics
Monitoring and metrics are essential for effective load balancing. The system should track key distribution and node performance continuously. Metrics such as load averages and response times provide valuable insights. These metrics help identify imbalances and potential bottlenecks. Regular monitoring ensures that the system can make informed adjustments. This proactive approach maintains optimal load distribution and system reliability.
Fault Tolerance Mechanisms
Replication Strategies
Replication strategies enhance fault tolerance in Consistent Hashing. The system replicates data across multiple nodes to ensure availability. If a node fails, other nodes can quickly take over its responsibilities. This redundancy minimizes data loss and downtime. Virtual nodes further improve fault tolerance by distributing replicas evenly. Consistent Hashing's structure allows for efficient replication, maintaining continuous operation.
Recovery Procedures
Recovery procedures are critical for handling node failures. The system must quickly detect and respond to node failures. Reassigning keys from failed nodes to active ones ensures data availability. Consistent Hashing's virtual node mechanism facilitates this process. Implementing automated recovery procedures can further enhance fault tolerance. These procedures ensure that the system remains resilient and reliable, even during failures.
Real-World Applications
Use Cases in Industry
Distributed Databases
Distributed databases rely heavily on Consistent Hashing to manage data distribution across multiple nodes. Amazon's DynamoDB and Apache Cassandra are prime examples. These databases use Consistent Hashing to ensure that data remains evenly distributed, even as nodes join or leave the system. This technique minimizes data movement, which enhances performance and stability. Consistent Hashing also helps maintain fault tolerance by redistributing data efficiently when node failures occur.
Content Delivery Networks (CDNs)
Content Delivery Networks (CDNs) benefit significantly from Consistent Hashing. CDNs like Akamai use this technique to distribute content across various servers globally. Consistent Hashing ensures that requests for content are routed to the nearest server, reducing latency and improving load times. This method also helps balance the load among servers, preventing any single server from becoming a bottleneck. By minimizing data movement during server additions or removals, CDNs maintain high availability and reliability.
Specific Programming Languages
Implementations in Python
Python offers robust libraries for implementing Consistent Hashing. Developers can use libraries like hash_ring
to create hash rings and manage virtual nodes. Python's flexibility allows for easy integration of Consistent Hashing into various applications. The language's simplicity makes it an excellent choice for prototyping and testing Consistent Hashing algorithms. Python's extensive community support ensures that developers can find resources and examples to guide their implementations.
Implementations in Java
Java provides powerful tools for implementing Consistent Hashing in enterprise applications. Libraries such as Guava
offer built-in support for hash functions and data structures needed for Consistent Hashing. Java's performance and scalability make it suitable for large-scale distributed systems. The language's strong typing and robust error handling enhance the reliability of Consistent Hashing implementations. Java's widespread use in industry ensures that developers can leverage existing knowledge and frameworks to build efficient distributed systems.
Conclusion
Consistent Hashing offers significant benefits for distributed systems. The technique ensures efficient data distribution and load balancing. Consistent Hashing minimizes data movement during node additions or removals. This method enhances scalability and fault tolerance.
Consistent Hashing plays a crucial role in modern distributed systems. The technique provides a scalable and flexible solution. Consistent Hashing maintains uniform data distribution and reduces the need for rehashing. This approach ensures system stability and performance.
Exploring Consistent Hashing further can lead to valuable implementations. Developers should consider integrating this technique into relevant projects. Consistent Hashing enhances the efficiency and reliability of distributed systems.
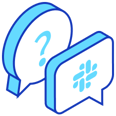
Join StarRocks Community on Slack
Connect on Slack