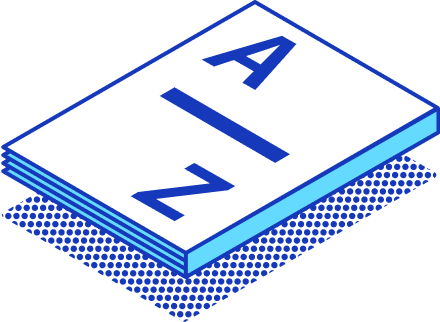
Data Manipulation Language (DML): A Guide to Database Data Management
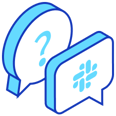
Join StarRocks Community on Slack
Connect on SlackIn the world of databases, Data Manipulation Language (DML) is the set of commands that empowers you to work directly with the data inside your database tables. Think of DML as the toolbox that allows you to add, view, update, and delete data stored in databases, such as MySQL, SQL Server, and PostgreSQL. Using DML effectively not only makes data management easier but also helps ensure data accuracy, integrity, and security.
Whether you're new to DML or looking to refine your knowledge, let’s explore the core DML commands and some best practices for efficient data manipulation.
Core DML Commands: The Basics
Understanding DML commands is essential for interacting with data, as they let you handle everything from adding new entries to keeping data up-to-date.
INSERT Command: Adding New Data
The INSERT
command lets you add new records to a table, which is often the starting point in a database. Think of it like filling out a new row in an Excel sheet, where each column represents a specific type of information.
Basic Syntax and Usage
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
Here, you specify the table name and the columns you want to populate, followed by the values that match each column.
Example: Adding a New Student
Suppose you have a Students
table with columns ID
, Name
, and Age
. To add a new student named John Doe, who is 20 years old, you would use:
INSERT INTO Students (ID, Name, Age)
VALUES (1, 'John Doe', 20);
This command adds a new row with the specified values, like filling out a new row on a spreadsheet but with the added security and scalability of a database.
SELECT Command: Retrieving Data
The SELECT
command is used to view data in your database. With this command, you can specify which columns you’d like to retrieve and add conditions to narrow down results, similar to filtering data in a spreadsheet.
Basic Syntax and Usage
SELECT column1, column2, ...
FROM table_name
WHERE condition;
This format allows you to see the data you need, rather than pulling in everything from the table.
Example: Finding Students Over 18
Imagine you want to find all students older than 18. Here’s how you could use SELECT
:
SELECT Name, Age
FROM Students
WHERE Age > 18;
This command returns the names and ages of all students who are over 18, showing only the information you care about.
UPDATE Command: Modifying Existing Data
The UPDATE
command is for modifying existing records. It’s like editing a row in a table, where you select specific columns to update with new values.
Basic Syntax and Usage
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
This lets you change data for specific rows while leaving the rest untouched.
Example: Updating a Student's Age
Say John Doe just had a birthday, and you need to update his age to 21:
UPDATE Students
SET Age = 21
WHERE Name = 'John Doe';
This command will only affect the row where the name matches 'John Doe', ensuring accuracy without impacting other records.
DELETE Command: Removing Data
The DELETE
command is for removing records from a table. It’s useful for keeping your database clean and ensuring outdated or incorrect data doesn’t linger.
Basic Syntax and Usage
DELETE FROM table_name
WHERE condition;
Be cautious with DELETE
—without a WHERE
clause, it will delete all rows in the table!
Example: Removing a Student Record
Suppose John Doe has graduated, and you need to delete his record:
DELETE FROM Students
WHERE Name = 'John Doe';
This command removes only the row where the student’s name is 'John Doe', leaving the rest of the data intact.
Advanced DML Techniques and Best Practices
Once you’ve mastered the basics, advanced DML techniques can help you take data management to the next level, making your operations more secure, efficient, and resilient.
Parameterized Queries: Safeguarding Your Database
Parameterized queries allow you to insert or update data using placeholders, which adds a layer of security and efficiency. One major advantage of parameterized queries is protection against SQL injection attacks, a common security vulnerability where attackers try to execute harmful SQL code through user input.
Example: Preventing SQL Injection with a Parameterized Query
Consider this query, where user input is used as a parameter:
SELECT * FROM Students WHERE Name = ?;
Here, ?
acts as a placeholder for a name input. This setup prevents any code within the input from being executed, so only data is processed, not potential harmful commands.
Transactions: Ensuring Data Integrity
A transaction is a set of DML operations that are executed as a unit. If all parts of the transaction succeed, you can commit the changes. If any part fails, you can roll back the entire transaction to its original state, avoiding incomplete updates that could compromise data integrity.
Example: Using Transactions with COMMIT and ROLLBACK
Let’s say you’re updating multiple tables for a new semester and need to ensure that all changes are successful before saving:
BEGIN TRANSACTION;
UPDATE Students SET Age = 21 WHERE Name = 'John Doe';
UPDATE Courses SET Credits = 4 WHERE CourseID = 101;
COMMIT;
If something goes wrong, you can cancel the transaction using ROLLBACK
:BEGIN TRANSACTION;
UPDATE Students SET Age = 21 WHERE Name = 'John Doe';
-- An error occurs here
ROLLBACK;
This way, you avoid partial updates and maintain data consistency.
Optimizing Queries: Improving Performance
Optimization is crucial when working with large databases. For example, indexes are special database structures that make searching faster by allowing you to jump directly to the data you need instead of scanning every row.
-
Example: Creating an Index for Faster Searches
If you often search by student name, creating an index on the
Name
column can speed up these queries:CREATE INDEX idx_name ON Students(Name);
Additionally, using
EXPLAIN
in SQL can help you analyze the execution plan of a query to identify potential bottlenecks and optimize them.
DML in Different Database Systems
While SQL-based DML commands are standardized, each database system has its unique implementation quirks. Knowing these variations helps you adapt your skills to different environments.
SQL Databases: Structured and Relational
SQL databases like MySQL and PostgreSQL are relational and schema-based, meaning they store data in well-defined tables with structured relationships.
Example: DML in MySQL or PostgreSQL
To add a record in MySQL or PostgreSQL:
INSERT INTO Employees (ID, Name, Position)
VALUES (101, 'Alice Smith', 'Manager');
And to retrieve specific data:
SELECT Name, Position
FROM Employees
WHERE Position = 'Manager';
NoSQL Databases: Flexibility and Scalability
NoSQL databases like MongoDB and Cassandra store data in more flexible, non-relational formats, making them ideal for unstructured data or applications needing rapid scaling.
Example of DML in MongoDB or Cassandra
In MongoDB, a document-based NoSQL database, you manipulate data using JSON-like documents. To insert a new document, you use the insertOne
method:
db.Employees.insertOne({ ID: 101, Name: 'Alice Smith', Position: 'Manager' });
To query data, you use the find
method:
db.Employees.find({ Position: 'Manager' });
Cassandra, a column-family NoSQL database, uses CQL (Cassandra Query Language) for data manipulation. To insert data, you use the INSERT
command:
INSERT INTO Employees (ID, Name, Position)
VALUES (101, 'Alice Smith', 'Manager');
To retrieve data, you use the SELECT
command:
SELECT Name, Position
FROM Employees
WHERE Position = 'Manager';
Key Takeaways: Mastering DML for Effective Database Management
Mastering DML techniques allows you to efficiently manipulate data, keep your database accurate, and enhance performance. Advanced techniques like parameterized queries and transactions safeguard against security risks and inconsistencies, while query optimization ensures your system runs smoothly as your data grows.
Remember, the world of databases is ever-evolving, so continuous learning is key. By staying current with database advancements, you'll ensure your DML skills remain robust, relevant, and effective.