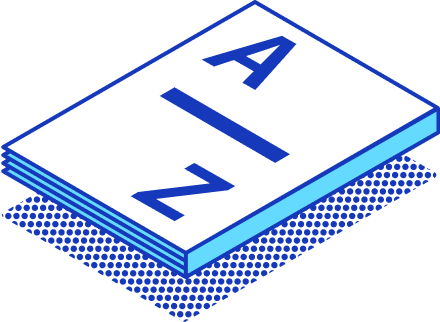
A Closer Look at Data Retrieval in Databases
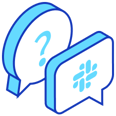
Join StarRocks Community on Slack
Connect on SlackWhat is Data Retrieval?
Data retrieval refers to the process of accessing information stored in databases to meet specific needs or answer specific queries. It involves submitting a query—a structured request—that is processed by the database management system (DBMS) to return precise results.
Why is Data Retrieval Important?
Modern organizations generate and rely on vast amounts of data for both operational and strategic purposes. Efficient data retrieval is essential for:
- Decision-Making: Quickly accessing relevant data to make informed choices.
- Analysis and Reporting: Producing dashboards, visualizations, and performance metrics.
- Customer Insights: Identifying behavioral patterns and preferences through trends.
Key Components of Data Retrieval
Queries
- Structured requests formulated using query languages such as SQL (Structured Query Language) or APIs.
- Queries specify criteria for data selection, filtering, aggregation, and formatting.
Database Management Systems (DBMS)
- Software responsible for managing, organizing, and retrieving data based on user queries.
- Examples: MySQL, PostgreSQL, MongoDB, and Redis.
Results
- Data returned by the DBMS in formats like tables, JSON, or XML, tailored to the query requirements.
Databases and Storage Structures
Databases form the backbone of data retrieval, storing vast amounts of information in an organized manner. Efficient storage structures facilitate quicker and more accurate access.
Storage Components
- Tables: Foundational structure in relational databases, organizing data into rows (records) and columns (attributes).
- Indexes: Specialized data structures that improve search efficiency by providing direct paths to data.
- Example: A B-tree index accelerates queries on a column like
OrderDate
.
- Example: A B-tree index accelerates queries on a column like
- Schemas: Define the logical organization, relationships, and constraints within a database.
Best Practices:
- Employ indexing for frequently queried columns.
- Normalize data to reduce redundancy and enhance consistency.
Query Languages and Interfaces
Query languages and user interfaces make data retrieval accessible for developers, analysts, and even non-technical users.
SQL (Structured Query Language)
- Widely used in relational databases for operations like filtering (
SELECT
), joining tables (JOIN
), and aggregating data (SUM
,AVG
). - Example:
SELECT ProductName, SUM(Sales)
FROM SalesData
WHERE Region = 'North'
GROUP BY ProductName;
NoSQL Interfaces
- Designed for flexible, schema-less environments such as key-value, document, or graph databases.
- Example (MongoDB Query)
db.products.find({ "category": "electronics" })
User-Friendly Interfaces
- Graphical User Interfaces (GUIs): Tools like Tableau and Microsoft Access simplify querying and visualization.
- APIs: Allow programmatic retrieval of data using HTTP requests.
- Example:
curl -X GET "https://api.example.com/users"
Best Practices:
- Use parameterized queries to avoid SQL injection.
- Cache frequent API responses to minimize database load.
Types of Databases and Their Roles
Databases are the backbone of data storage and retrieval, designed to manage vast amounts of structured and unstructured information.
Types of Databases
Relational Databases (SQL):
- Structure: Data is stored in tables with predefined schemas, where rows represent records and columns represent attributes.
- Language: SQL (Structured Query Language) is used for data retrieval.
- Examples: MySQL, PostgreSQL, Oracle DB, Microsoft SQL Server.
- Use Case: Transactional systems like e-commerce platforms and banking.
Example SQL Query:
SELECT ProductName, Sales
FROM Products
WHERE Sales > 1000
ORDER BY Sales DESC;
NoSQL Databases:
- Structure: Flexible, handling unstructured or semi-structured data such as JSON or BSON documents.
- Examples: MongoDB, Cassandra, DynamoDB, Couchbase.
- Use Case: Real-time data handling for applications like social media, IoT, or recommendation engines.
Example MongoDB Query:
db.products.find({ "category": "electronics" })
Cloud Databases:
-
- Features: Scalability, availability, and cost-effectiveness.
- Examples: AWS RDS, Google Cloud Firestore, Azure Cosmos DB.
- Use Case: SaaS applications requiring scalable infrastructure.
Role of Database Systems in Data Retrieval
Each type of database has unique features that influence retrieval processes. For example:
- Relational Databases: Use optimized SQL queries with indexing for precise, structured data retrieval.
- NoSQL Databases: Handle large volumes of diverse data efficiently, using document or key-value lookups.
- Cloud Databases: Provide elastic scaling for unpredictable workloads, ensuring consistent performance.
Methods of Data Retrieval
Data retrieval methods depend on the database structure, query language, and application requirements. Let’s explore the most common approaches:
SQL Queries
Overview: SQL (Structured Query Language) is the industry standard for interacting with relational databases.
SQL Commands:
- SELECT: Extract specific columns or rows. Example:
SELECT name, age FROM Employees WHERE department = 'Sales';
- JOIN: Combine data from multiple tables. Example:
SELECT Orders.OrderID, Customers.Name
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
- Aggregate Functions: Perform calculations like
SUM
,AVG
,COUNT
.
SELECT department, AVG(salary) AS AvgSalary
FROM Employees
GROUP BY department;
Best Practices for SQL Retrieval:
- Use indexes for faster lookups.
- Avoid
SELECT *
in production environments to reduce unnecessary data retrieval. - Use parameterized queries to mitigate SQL injection risks.
APIs for Data Retrieval
Overview: APIs (Application Programming Interfaces) provide a standardized way to access external or remote data sources.
Example API Workflow:
- Send a GET request:
curl -X GET "https://api.example.com/v1/users"
- Receive structured data (e.g., JSON):
{
"users": [
{ "id": 1, "name": "John Doe", "age": 30 },
{ "id": 2, "name": "Jane Smith", "age": 25 }
]
}
Best Practices:
- Handle rate limits to avoid API blocking.
- Use authentication tokens for secure data access.
- Implement caching to reduce redundant API calls.
NoSQL Queries
NoSQL databases offer diverse retrieval methods based on their design:
- Document-based: Query documents using flexible attributes.
- Key-value: Access data using unique keys.
- Graph-based: Traverse nodes and edges for complex relationships.
Example Use Case:
- Querying a graph database like Neo4j to find connected users:
MATCH (user1:User)-[:FRIENDS_WITH]->(user2:User)
WHERE user1.name = 'Alice'
RETURN user2.name;
Optimizing Database Performance for Faster Data Retrieval
Efficient data retrieval is critical for ensuring fast and accurate access to information, particularly in complex systems with large datasets. Multiple factors affect retrieval efficiency, including data size, query design, and hardware infrastructure. Here’s a breakdown of key elements and strategies to optimize performance:
Dataset Size: Challenges and Solutions
Challenges
As datasets grow in size, retrieval operations face scalability challenges. Large datasets can lead to:
- Increased Query Processing Time: More rows to scan and process.
- Higher Resource Consumption: Increased strain on CPU, memory, and storage systems.
- Reduced Performance: Without optimization, larger datasets can slow down response times.
Solutions
Indexing:
-
- Indexes act as shortcuts to locate data efficiently.
- Example: Creating a B-tree index on frequently queried columns.
- Use Case: Querying orders by
OrderDate
in an e-commerce database.
CREATE INDEX idx_order_date ON Orders (OrderDate);
Partitioning:
- Divides a large dataset into smaller, manageable chunks based on criteria such as date ranges or regions.
- Example: Partitioning sales data by year.
CREATE TABLE Sales (
SaleID INT,
SaleDate DATE,
Amount DECIMAL
) PARTITION BY RANGE (SaleDate);
Sharding:
-
- Distributes data across multiple servers or nodes to handle high query loads.
- Example: Using sharding in MongoDB to distribute customer data geographically.
Query Optimization: Writing Efficient Queries
Poorly written queries can lead to slow performance and high resource usage. Query optimization techniques include:
-
Avoid Unnecessary Joins:
- Minimize joins by restructuring queries or denormalizing tables where appropriate.
-
- Pre-compute and store query results for frequently accessed data.
- Example: Creating a materialized view for monthly sales summaries.
-
Use Query Execution Plans:
- Tools like
EXPLAIN
in SQL help identify bottlenecks and suggest improvements.
- Tools like
Hardware and Infrastructure: The Impact of Technology Choices
In-Memory Databases:
- Databases like Redis and Memcached store data in RAM for real-time performance.
- Use Case: Real-time leaderboard updates in gaming applications.
Optimize Storage:
- Use SSDs for faster input/output (I/O) operations compared to traditional HDDs.
- Example: Migrating frequently accessed data to SSD storage to reduce latency.
Distributed Systems:
- Leverage distributed databases like Apache Cassandra for high availability and fault tolerance.
Practical Applications of Data Retrieval
Data retrieval enables actionable insights across diverse industries, from business decision-making to enhancing user experiences.
Business Use Cases
E-commerce:
- Application: Optimizing inventory by analyzing customer purchase trends.
- Example: Retrieve the top 10 best-selling products
SELECT ProductName, COUNT(*) AS PurchaseCount
FROM Orders
GROUP BY ProductName
ORDER BY PurchaseCount DESC
LIMIT 10;
Finance:
- Application: Fraud detection by monitoring high-value transactions.
- Example: Query transactions over $10,000 within the last week.
SELECT TransactionID, Amount, CustomerID
FROM Transactions
WHERE Amount > 10000 AND TransactionDate > CURRENT_DATE - INTERVAL 7 DAY;
Technology Use Cases
Search Engines:
- Application: Ranking web pages based on relevance using advanced retrieval algorithms.
- Example: Google uses PageRank to prioritize search results.
Streaming Services:
- Application: Real-time content recommendations based on user preferences.
- Example: Netflix uses collaborative filtering algorithms to suggest movies.
Challenges and Solutions in Data Retrieval
Efficient data retrieval often involves navigating technical challenges while maintaining performance and security.
Challenges
-
Data Integrity:
- Risk: Outdated or corrupted data can lead to incorrect insights.
- Solution: Implement regular data validation and deduplication processes.
-
Security Concerns:
- Risk: Data breaches and unauthorized access.
- Solution:
- Encrypt sensitive data both at rest and in transit.
- Implement access control mechanisms like role-based access control (RBAC).
Solutions and Best Practices
-
Index Maintenance:
- Regularly update and rebuild indexes to ensure consistent performance.
-
Query Caching:
- Store frequent query results to minimize database load.
- Example: Caching product details for an e-commerce application.
-
Data Masking:
- Hide sensitive information in query outputs to ensure compliance with privacy regulations.
- Example: Masking credit card numbers in query results.
SELECT CONCAT('****-****-****-', RIGHT(CreditCardNumber, 4)) AS MaskedCard
FROM Customers;
Future Trends in Data Retrieval
Emerging technologies and techniques are shaping the future of data retrieval, promising improved efficiency and capabilities.
Emerging Technologies
-
AI and Machine Learning:
- Intelligent query optimization and semantic search capabilities.
- Example: Elasticsearch uses machine learning to predict user intent and rank results.
-
- Provides flexibility by allowing clients to specify only the data they need.
- Example: Query nested objects in a single request
query {
user(id: "1") {
name
posts {
title
comments {
content
}
}
}
}
- Augmented Retrieval Techniques:
- Retrieval-Augmented Generation (RAG) combines search with generative AI for tasks like summarization.
- Example: Chatbots retrieving and summarizing documents for user queries.
Anticipated Challenges
-
Balancing Speed and Energy Efficiency:
- Optimization techniques must balance performance with sustainability goals.
-
Compliance with Privacy Regulations:
- Ensuring compliance with GDPR and similar laws as retrieval systems become more sophisticated.
Key Takeaways
Data retrieval is fundamental to modern data management, supporting decision-making, analysis, and innovation across industries. By adopting efficient practices, leveraging advanced tools, and embracing emerging trends, organizations can achieve faster, more secure, and scalable access to critical information.