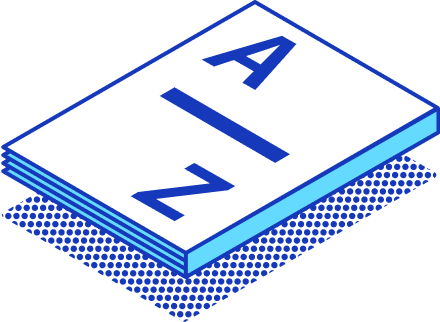
Depth-First Search (DFS)
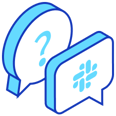
Join StarRocks Community on Slack
Connect on SlackWhat Is Depth-First Search (DFS)
Definition and Basic Concepts
Graph Theory Basics
Graphs are like maps. They show connections between things. Nodes represent points, and edges connect them. You can think of nodes as cities and edges as roads. Graphs can be directed or undirected. Directed graphs have one-way streets. Undirected graphs have two-way streets. Graphs can also have cycles. Cycles are paths that loop back to the starting point.
DFS Algorithm Overview
The DFS algorithm explores graphs by diving deep into each branch. The DFS algorithm starts at a node and travels along a path until it can't go further. Then, the DFS algorithm backtracks to explore other paths. The DFS algorithm uses a stack to keep track of nodes. The DFS algorithm can handle cycles by marking visited nodes. The DFS algorithm ensures each node gets visited once.
How the Depth-First Search Algorithm Works
Recursive Approach
The recursive approach uses function calls. The DFS algorithm calls itself for each unvisited neighbor. This method is like exploring a maze. You go down one path until you hit a dead end. Then, you backtrack and try another path. The DFS algorithm keeps track of visited nodes to avoid loops. The recursive approach is simple but can use a lot of memory.
Iterative Approach
The iterative approach uses a stack. The DFS algorithm pushes the starting node onto the stack. The DFS algorithm pops a node from the stack and visits it. The DFS algorithm pushes unvisited neighbors onto the stack. This process repeats until the stack is empty. The iterative approach is more memory-efficient than recursion. The DFS algorithm still explores all paths in the graph.
Depth-First Search (DFS) Pseudocode
Understanding the pseudocode of Depth-First Search (DFS) helps you grasp how this algorithm works. The pseudocode provides a step-by-step guide to implementing DFS in any programming language. Let's dive into both recursive and iterative approaches.
Recursive Pseudocode
Explanation of Steps
The recursive approach to Depth-First Search (DFS) involves calling a function repeatedly until all nodes are visited. Here's how it works:
-
Start at the initial node.
-
Mark the current node as visited.
-
For each unvisited neighbor, call the DFS function recursively.
-
Continue until all nodes connected to the starting node are visited.
This method uses the call stack to keep track of nodes. The recursion stops when there are no more unvisited neighbors.
Example Walkthrough
Imagine you have a graph with nodes A, B, C, and D. You start at node A:
-
Visit A and mark it as visited.
-
Move to an unvisited neighbor, say B.
-
Visit B and mark it as visited.
-
From B, move to another unvisited neighbor, C.
-
Visit C and mark it as visited.
-
If C has no unvisited neighbors, backtrack to B, then to A.
-
Finally, visit any remaining unvisited nodes like D.
This process ensures that you explore each path fully before backtracking.
Iterative Pseudocode
Explanation of Steps
The iterative approach to Depth-First Search (DFS) uses a stack data structure. Here's how it unfolds:
-
Push the starting node onto the stack.
-
While the stack is not empty, pop a node from the stack.
-
If the node is unvisited, mark it as visited.
-
Push all unvisited neighbors onto the stack.
-
Repeat until the stack is empty.
This method avoids the overhead of recursive calls by using an explicit stack.
Example Walkthrough
Consider the same graph with nodes A, B, C, and D:
-
Push A onto the stack.
-
Pop A and mark it as visited.
-
Push unvisited neighbors like B onto the stack.
-
Pop B, mark it as visited, and push its unvisited neighbors like C.
-
Continue this process until all nodes are visited.
-
The stack helps manage which nodes to visit next.
Both recursive and iterative methods achieve the same goal. They explore all paths in a graph using Depth-First Search (DFS). Each method has its own advantages. The recursive approach is simpler but can use more memory. The iterative approach is more memory-efficient.
Complexity Analysis of the Depth-First Search Algorithm
Understanding the complexity of Depth-First Search (DFS) helps you grasp its efficiency in various scenarios. Let's dive into the time and space complexity of this essential algorithm.
Time Complexity
The time complexity of DFS depends on the structure of the graph. In general, DFS has a time complexity of O(V + E)
. Here, V represents the number of vertices, and E represents the number of edges in the graph. This complexity arises because DFS visits each vertex and edge once.
-
Directed Graphs: In directed graphs, DFS maintains a time complexity of
O(V + |E|)
. Each edge gets visited once as DFS explores paths. -
Undirected Graphs: For undirected graphs, the time complexity remains
O(V + 2|E|)
. Each edge gets visited twice, once from each direction. -
Trees: When DFS operates on trees, the time complexity simplifies to
O(V)
. Trees have no cycles, so DFS visits each node once.
DFS efficiently handles different graph structures, making it a versatile tool in your Data Structure toolkit.
Space Complexity
Space complexity in DFS primarily relates to the storage of nodes in the stack data structure. The space complexity of DFS is O(V)
. This complexity arises because the stack stores nodes during traversal.
-
Memory Usage: DFS uses memory to keep track of visited nodes. The stack grows with the depth of the graph. In the worst case, the stack holds all vertices, leading to
O(V)
space usage.
DFS's space complexity makes it suitable for exploring large graphs without excessive memory consumption. The stack data structure efficiently manages nodes, ensuring DFS remains effective.
DFS's complexity analysis highlights its ability to navigate complex graph data structures efficiently. You can use DFS to explore paths, detect cycles, and perform topological sorting. Understanding these complexities equips you with the knowledge to apply DFS effectively in various scenarios. Whether you're tackling pathfinding problems or solving puzzles, DFS offers a reliable solution in your Guide to Understand Data Structure and Algorithm.
Practical Applications of Depth-First Search (DFS)
Depth-First Search (DFS) isn't just a theoretical concept. You can find DFS in many real-world applications. Let's explore some practical uses of this powerful Search Algorithm.
Pathfinding in Mazes
Mazes present a classic challenge. Depth-First Search shines in these scenarios. Imagine you're navigating a maze. You need to explore every possible path to find the exit. DFS helps you achieve that goal efficiently.
Example Scenarios
In video games, characters often navigate complex environments. Developers use DFS to ensure characters find their way through mazes or dungeons. For instance, a game might feature a labyrinth with multiple exits. DFS explores each path until it finds a solution. This ensures players experience a seamless adventure.
Robotics also benefits from DFS. Robots equipped with sensors use DFS to navigate unknown terrains. The algorithm guides robots through obstacles and dead ends. This allows them to reach their destination without getting stuck.
Solving Puzzles
Puzzles often require exploring various possibilities. Depth-First Search excels in solving these challenges. Whether it's a Sudoku puzzle or a jigsaw, DFS helps you find solutions by examining all potential moves.
Use Cases in Games
Puzzle games frequently rely on DFS. Consider a game where you must connect dots without crossing lines. DFS explores each connection possibility. This ensures you find a valid solution without missing any options.
In board games like chess, DFS aids in decision-making. The algorithm evaluates potential moves and outcomes. This allows players to strategize effectively and anticipate opponents' actions.
DFS proves invaluable in these scenarios. The algorithm's ability to explore all paths makes it a go-to tool for developers and engineers. Whether you're designing a game or programming a robot, DFS provides a reliable Guide to navigating complex challenges.
Implementing Depth-First Search (DFS) in Code
Implementing Depth-First Search (DFS) in code can feel like a rewarding challenge. You get to see the algorithm come to life on your screen. Let's dive into the Python code examples for both recursive and iterative implementations.
Code Examples in Python
Recursive Implementation
The recursive approach to DFS uses function calls to explore nodes. Here's a simple Python example:
def dfs_recursive(graph, node, visited):
if node not in visited:
print(node)
visited.add(node)
for neighbor in graph[node]:
dfs_recursive(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
visited = set()
dfs_recursive(graph, 'A', visited)
This code starts at node 'A' and explores each path deeply before backtracking. The visited
set keeps track of nodes to prevent revisiting.
Iterative Implementation
The iterative approach uses the Stack data structure to manage nodes. Here's how you can implement it in Python:
def dfs_iterative(graph, start):
visited = set()
stack = [start]
while stack:
node = stack.pop()
if node not in visited:
print(node)
visited.add(node)
stack.extend(reversed(graph[node]))
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
dfs_iterative(graph, 'A')
In this version, the stack manages which nodes to visit next. The algorithm pops nodes from the stack and visits them, adding unvisited neighbors back to the stack.
Common Pitfalls and Debugging Tips
Implementing DFS can sometimes lead to common pitfalls. Understanding these issues helps you debug effectively.
Avoiding Infinite Loops
Infinite loops occur when the algorithm revisits nodes endlessly. To avoid this, always mark nodes as visited. Use a set or list to track visited nodes. This ensures each node gets processed only once.
Handling Large Graphs
Large graphs can overwhelm memory resources. The recursive approach might hit a recursion limit. For large graphs, prefer the iterative method. The Stack data structure handles depth without excessive memory use. Optimize by limiting depth or using iterative deepening.
Implementing DFS in Python offers a hands-on way to grasp the algorithm's mechanics. Whether you choose recursion or iteration, understanding these methods equips you to tackle complex graph problems. Keep experimenting and refining your skills to become proficient in DFS.
Conclusion
Depth-First Search (DFS) offers a powerful tool for navigating complex data structures. You explored key concepts, techniques, and practical applications. DFS plays a crucial role in the toolkit of a Full Stack Java Developer. Keep diving deeper into DFS to enhance your skills as a Full Stack Developer. The journey to mastering DFS will enrich your path as a Full Stack professional. Your feedback and questions are welcome. Share your thoughts and experiences with DFS. Engage with the community of Full Stack Java Developers. Your insights can inspire others on their Full Stack journey.