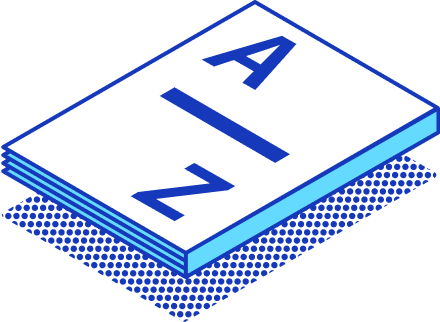
The ABCs of JSON: A Starter’s Guide
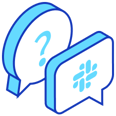
Join StarRocks Community on Slack
Connect on SlackJSON, short for JavaScript Object Notation, is a lightweight format used to store and exchange data. Its simple structure makes it easy for you to read and write. Machines can also parse and generate JSON data without difficulty. This format is inspired by JavaScript object literal syntax, which contributes to its human-readable nature.
Understanding JSON is essential because it works seamlessly across different programming languages. Developers favor it for its ability to simplify data exchange. Whether you're building a website or an app, JSON ensures smooth communication between systems.
Key Takeaways
-
JSON means JavaScript Object Notation. It is simple and easy to read for saving and sharing data.
-
JSON is popular in web development, especially in APIs, because it is easy to use and works with many coding languages.
-
Learning JSON rules is important. Using key-value pairs, arrays, and correct formatting makes good JSON data.
-
JSON is better than XML because it is smaller and quicker to process, making it great for sharing data.
-
Try making and reading JSON data to get better at using it in real-life projects.
What is JSON?
Definition and significance of JSON
JSON, or JavaScript Object Notation, is a lightweight and human-readable data format. It allows you to store and exchange data efficiently between systems. JSON originated in 2001 when Douglas Crockford and his team sought a better way to enable real-time communication between servers and browsers. They wanted to avoid relying on plugins like Flash or Java applets. The first JSON message was sent in April 2001, marking a pivotal moment in computing history.
JSON’s simplicity and flexibility make it a cornerstone of modern programming. Developers worldwide prefer it for its ability to handle data transfer seamlessly. It plays a crucial role in web development, especially in AJAX applications, where it enables dynamic updates without reloading the entire page. JSON’s straightforward structure ensures that you can easily read, write, and parse it, even if you’re new to programming.
Why JSON is a universal data format
JSON has become a universal data format because of its adaptability and efficiency. It is based on a subset of JavaScript, but you can use it with almost any programming language, including Python, Java, and Ruby. This cross-language compatibility makes JSON an ideal choice for developers working on diverse platforms.
The transition from XML to JSON marked a significant shift in data interchange practices. JSON surpassed XML in popularity due to its lightweight nature and ease of use. Unlike XML, JSON eliminates the need for verbose tags, reducing file size and improving readability. Its flexibility allows you to represent complex data structures like objects and arrays effortlessly.
JSON’s role as the backbone of efficient data transfer mechanisms, such as RESTful APIs, further highlights its importance. Whether you’re building a web application or integrating third-party services, JSON ensures smooth communication between systems. Its widespread adoption across industries underscores its status as a universal standard for data exchange.
Understanding JSON Syntax
Key components of JSON
Objects and key-value pairs
JSON objects are the foundation of this data interchange format. They represent structured data using key-value pairs. A key is always a string, while the value can be a string, number, boolean, array, object, or null. For example, in the JSON object {"name": "Alice", "age": 30}
, "name" is the key, and "Alice" is the value. You can use JSON objects to organize data logically, making it easier to access and manipulate.
Arrays and their structure
Arrays in JSON allow you to store multiple values in an ordered list. These values can include strings, numbers, objects, or even other arrays. For instance, ["apple", "banana", "cherry"]
is a JSON array containing strings. Arrays are useful when you need to represent a collection of related items, such as a list of names or phone numbers.
Data types in JSON
JSON supports several data types, including:
-
Strings: Represented in double quotes, like
"hello"
. -
Numbers: Can be integers or decimals, such as
42
or3.14
. -
Booleans: Represent true or false values.
-
Null: Represents an empty or undefined value.
-
Arrays: Ordered lists of values.
-
Objects: Collections of key:value pairs.
JSON formatting rules
Proper use of commas, colons, and brackets
To create valid JSON, you must follow specific JSON syntax rules. Use colons to separate keys and values, commas to separate items in arrays or objects, and curly braces {}
to define objects. Square brackets []
are used for arrays. For example:
{
"name": "Alice",
"age": 30,
"hobbies": ["reading", "cycling"]
}
Common syntax errors to avoid
Avoid these common mistakes when writing JSON:
-
Forgetting to enclose keys or strings in double quotes.
-
Using trailing commas after the last item in an object or array.
-
Mixing single and double quotes.
-
Leaving out colons between keys and values.
Example of JSON syntax
Here’s an example of a JSON object with annotations:
{
"first_name": "John", // A string representing the first name
"last_name": "Smith", // A string representing the last name
"is_alive": true, // A boolean indicating if the person is alive
"age": 27, // A number representing the age
"address": { // A nested object for the address
"street_address": "21 2nd Street",
"city": "New York",
"state": "NY",
"postal_code": "10021-3100"
},
"phone_numbers": [ // An array of phone number objects
{
"type": "home",
"number": "212 555-1234"
},
{
"type": "office",
"number": "646 555-4567"
}
],
"children": ["Catherine", "Thomas", "Trevor"], // An array of strings
"spouse": null // A null value indicating no spouse
}
This example demonstrates how JSON organizes structured data in a clear and readable way.
Why JSON is Widely Used
Advantages of JSON
Lightweight and easy to read
JSON stands out for its simplicity and lightweight nature. Its syntax is concise, which reduces the size of data files. This makes it ideal for transmitting data over networks. You can easily read and write JSON because of its straightforward structure. This readability makes it a popular choice for configuration files and data exchange.
Language-independent data format
JSON is not tied to any specific programming language. You can use it with Python, JavaScript, Java, and many others. This versatility allows developers from different backgrounds to work with JSON seamlessly. Its language-agnostic nature makes it a universal tool for data exchange.
Compatibility with modern programming languages
Modern programming languages offer built-in support for JSON. This compatibility simplifies the process of parsing and generating JSON data. You can also represent complex data structures, such as arrays and nested objects, without difficulty. JSON’s widespread adoption in web APIs further highlights its importance in modern development.
JSON vs other data formats
JSON vs XML
JSON offers several advantages over XML:
-
JSON is easier to read due to its simple syntax.
-
JSON files are smaller because they avoid verbose tags.
-
JSON parsing is faster, which improves performance in applications.
XML, however, supports features like comments and attributes, which JSON lacks. Despite this, JSON’s efficiency and readability make it the preferred choice for most developers.
JSON vs YAML
When comparing JSON and YAML, consider their use cases:
-
Use JSON for APIs. Its compact format ensures efficient data interchange.
-
Use YAML for configuration files. Its human-readable structure makes it easier to edit manually.
-
Choose JSON if your project relies on programming languages with native JSON support.
JSON uses a straightforward syntax with key-value pairs. YAML, on the other hand, relies on indentation and whitespace to define structure. While YAML is more flexible, JSON’s well-defined specifications make it a reliable choice for data exchange.
A Beginner's Guide to Working with JSON
Creating JSON data
Writing JSON manually
When you create JSON data manually, you should follow best practices to ensure clarity and consistency. Start by maintaining a clean and organized structure. Use consistent name-value pairs and avoid unnecessary nesting. This makes your JSON easier to read and debug. For example:
{
"name": "John Doe",
"age": 25,
"skills": ["JavaScript", "Python", "HTML"]
}
Use JSON schema validation to detect inconsistencies early. Arrays work well for large strings of data, as they are intuitive and manageable. Keep your schemas simple to avoid overcomplicating data representation.
Generating JSON programmatically
You can generate JSON programmatically using libraries and tools available in various programming languages. Some popular options include:
-
Python:
simplejson
,jsonpickle
-
JavaScript:
JSON-js
,JSON 3
-
Java:
Gson
,Jackson
-
C++:
RapidJSON
,Nlohmann JSON
These tools simplify the process of creating JSON data, especially for complex or dynamic datasets.
Parsing JSON
Parsing JSON in JavaScript
Parsing JSON in JavaScript involves a few straightforward steps:
-
Use
JSON.parse()
to convert a JSON string into a JavaScript object. -
Handle JSON strings that represent arrays by iterating through them.
-
Access nested properties using chained dot notation.
-
Use a
try...catch
block to handle errors from invalid JSON inputs. -
Transform values during parsing with a reviver function.
For example:
const jsonString = '{"name": "Alice", "age": 30}';
try {
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Outputs: Alice
} catch (error) {
console.error("Invalid JSON string");
}
Parsing JSON in Python
In Python, you can parse JSON data using the built-in json
module. Use the json.loads()
method to convert a JSON string into a Python dictionary. For example:
import json
json_string = '{"name": "Bob", "age": 40}'
data = json.loads(json_string)
print(data["name"]) # Outputs: Bob
You can also handle nested JSON objects and arrays by accessing their keys or iterating through them.
Accessing and manipulating JSON data
Accessing JSON objects and arrays
Accessing JSON data involves retrieving values from objects or arrays. Use dot notation or bracket notation for objects. For arrays, loop through the elements. For example:
const data = {
"name": "Charlie",
"hobbies": ["reading", "gaming", "coding"]
};
console.log(data.name); // Outputs: Charlie
console.log(data.hobbies[1]); // Outputs: gaming
Modifying JSON data
You can modify JSON data by updating values or adding new key-value pairs. For example:
data.name = "Charlie Brown";
data.age = 35;
console.log(data);
This flexibility makes JSON ideal for dynamic applications.
Tools for working with JSON
Popular JSON libraries
When working with JSON, libraries can simplify your tasks by offering powerful features. Here are some popular options:
-
lodash: This library provides reliable deep equality checks for JSON objects. It ensures accuracy when comparing complex data structures.
-
deep-diff and jsondiffpatch: These JavaScript libraries help you detect differences between JSON objects. They also generate detailed reports and patches, making them useful for debugging or version control.
-
Jackson: Known for its speed, Jackson is one of the fastest libraries for parsing large JSON files. It consistently performs well, even with smaller files.
-
JSON.simple: This lightweight library is ideal for basic JSON parsing and generation. It works efficiently for small-scale projects.
-
GSON: While slower than Jackson, GSON offers flexibility. It allows you to convert Java objects to JSON and vice versa.
Choosing the right library depends on your project’s needs. For example, Jackson is better for performance-critical tasks, while lodash is perfect for comparing JSON objects.
Online JSON validators and formatters
Online tools make it easy to validate and format JSON data. These tools help you identify errors and ensure proper formatting. Some popular options include:
-
JSONLint: This tool checks your JSON for syntax errors. It highlights mistakes and provides suggestions for corrections.
-
JSON Formatter & Validator: This tool formats your JSON data for better readability. It also validates the syntax to ensure compliance with JSON standards.
-
JSON Diff: This tool compares two JSON files side by side. It highlights differences visually, making it easier to spot changes.
Using these tools saves time and reduces errors. You can quickly validate your JSON or compare files without writing additional code.
Practical Applications of JSON
JSON in APIs
How JSON is used in RESTful APIs
APIs often rely on JSON to exchange data between systems. RESTful APIs, in particular, use JSON as their standard format for requests and responses. When you send a request to an API, it typically responds with JSON data. This format ensures that the information is lightweight and easy to parse. JSON's compatibility with most programming languages makes it an ideal choice for web APIs. You can use it to retrieve, update, or delete data from a server without reloading a webpage.
Example of a JSON API response
Here’s an example of a JSON response from an API that provides video details:
{
"videos": [
{
"id": "12345",
"title": "Introduction to JSON",
"description": "A beginner's guide to JSON syntax and usage.",
"channel": {
"name": "Tech Tutorials",
"subscribers": 50000
}
},
{
"id": "67890",
"title": "Advanced JSON Techniques",
"description": "Learn how to work with complex JSON data.",
"channel": {
"name": "Code Academy",
"subscribers": 120000
}
}
]
}
Each video object includes details like its unique ID, title, description, and channel information. This structure makes it easy for you to extract and use specific data.
JSON in configuration files
Why JSON is ideal for configuration
JSON is a popular choice for configuration files because of its simplicity and readability. You can easily define settings using key-value pairs, which makes it straightforward to modify configurations. Its lightweight syntax ensures that configuration files remain compact. JSON's compatibility with various programming languages allows you to use the same configuration file across different systems.
Example of a JSON configuration file
Here’s an example of a JSON configuration file for a web application:
{
"app_name": "MyWebApp",
"version": "1.0.0",
"settings": {
"theme": "dark",
"language": "en-US",
"notifications": true
},
"database": {
"host": "localhost",
"port": 5432,
"username": "admin",
"password": "password123"
}
}
This file defines application settings, such as theme and language, along with database connection details. You can quickly update these values to customize the application.
JSON for data storage and transfer
JSON in databases
Modern databases often use JSON to store and manage data. JSON databases are flexible and allow you to store data without the constraints of structured tables. This flexibility is useful for applications like web development, session management, and gaming leaderboards.
-
JSON databases store data as individual objects, which eliminates row and table size limitations.
-
You can dynamically modify the schema by adding new attributes to documents.
-
JSON's simplicity makes it easy for both humans and machines to read and write data.
JSON for file-based data exchange
JSON is widely used for exchanging data between systems. Its lightweight and human-readable syntax makes it ideal for this purpose.
-
JSON supports complex data structures, such as nested objects and arrays, which makes it versatile.
-
It is language-agnostic, so you can use it with any programming language.
-
Machines can easily parse and generate JSON, simplifying data exchange processes.
For example, JSON is the standard format for web APIs, ensuring seamless communication between services and applications.
Limitations of JSON and Alternatives
Limitations of JSON
JSON is a powerful tool, but it has some drawbacks that you should consider:
-
No support for comments: JSON does not allow comments in its structure. This makes it harder to document or explain specific parts of your data, especially in configuration files.
-
Limited data types: JSON lacks native support for certain data types like dates. You must represent dates as strings or numbers, which can lead to inconsistencies.
-
Verbose for complex data: While JSON is lightweight, deeply nested or complex structures can become verbose. This can impact performance and bandwidth during data transfer.
-
No error handling: JSON does not include built-in mechanisms for error handling. Applications must manage errors, increasing development complexity.
-
Security risks: JSON can expose vulnerabilities when used with untrusted sources. Risks include insecure deserialization, injection attacks, and cross-site scripting (XSS).
To mitigate security risks, you should validate JSON data against a schema, use robust encoding practices, and implement a Content Security Policy (CSP).
Alternatives to JSON
XML: Pros and cons
XML is another widely used data format. It offers several advantages:
-
Pros:
-
XML supports schemas and namespaces, enabling robust validation.
-
It provides a consistent format for exchanging data across systems.
-
XML handles complex data structures effectively.
-
-
Cons:
-
XML files are more verbose than JSON, leading to larger file sizes.
-
Parsing XML is slower and more resource-intensive due to its extensive tagging.
-
XML is ideal for industries like healthcare and finance, where strict validation is essential.
YAML: Pros and cons
YAML is known for its readability and flexibility. Here’s how it compares to JSON:
-
Pros:
-
YAML is easier for humans to read and edit.
-
It supports comments, making it ideal for configuration files.
-
YAML represents complex data structures more naturally.
-
-
Cons:
-
YAML lacks native support in many programming languages.
-
Its complexity can make parsing slower compared to JSON.
-
YAML works well for infrastructure-as-code tools like Kubernetes and Docker.
When to choose JSON, XML, or YAML
Choosing the right format depends on your use case:
-
Use JSON for web APIs and applications requiring fast data parsing.
-
Choose XML for legacy systems or industries needing strict validation.
-
Opt for YAML when creating human-readable configuration files or managing cloud-based workflows.
Each format has strengths and weaknesses. Testing with real data can help you decide which one fits your project best.
JSON has revolutionized how data is exchanged and stored. Its simplicity and readability make it accessible to beginners, while its universality ensures compatibility with nearly all programming languages. You’ve learned that JSON uses objects and arrays as its core structures, with data represented in name-value pairs. Its lightweight format accelerates development and scales well for large applications.
Start practicing by creating and parsing JSON data. Experiment with APIs or configuration files to see its real-world applications. The more you work with JSON, the more confident you’ll become in leveraging its versatility.
FAQ
What does JSON stand for?
JSON stands for JavaScript Object Notation. It is a lightweight format used to store and exchange data. Despite its name, JSON works with many programming languages, not just JavaScript.
How is JSON different from XML?
JSON is simpler and easier to read than XML. It uses fewer characters, making it more lightweight. Unlike XML, JSON does not require opening and closing tags. This makes JSON faster to parse and more efficient for data exchange.
Can you use JSON with Python?
Yes, Python has built-in support for JSON through the json
module. You can use it to parse JSON strings into Python dictionaries or convert Python objects into JSON format. For example:
import json
data = json.loads('{"name": "Alice"}')
print(data["name"]) # Outputs: Alice
Is JSON case-sensitive?
Yes, JSON is case-sensitive. Keys and values must match exactly, including uppercase and lowercase letters. For example, "Name"
and "name"
are treated as two different keys.
How do you validate JSON?
You can validate JSON using online tools like JSONLint or programming libraries. These tools check for syntax errors and ensure your JSON follows proper formatting rules. Always validate JSON before using it in your application to avoid errors.