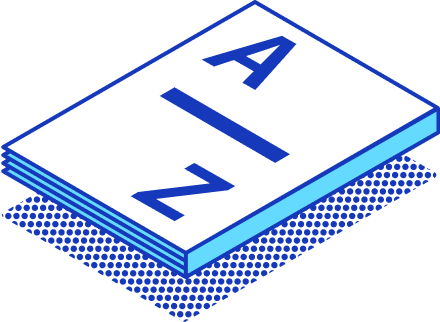
Why Data Structures Matter in Efficient Programming
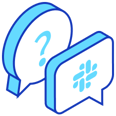
Join StarRocks Community on Slack
Connect on SlackUnderstanding Data Structures
Data structures are the foundation of efficient programming. They allow you to organize and manage data effectively, ensuring that your programs run smoothly and efficiently. By understanding data structures, you can optimize performance and enhance your problem-solving skills.
Definition and Purpose
Data structures are specialized formats for organizing and storing data. They enable you to perform operations like searching, sorting, and modifying data efficiently. The choice of data structure can significantly impact the performance of your algorithms. For instance, using a stack for managing function calls in a program can simplify the process and reduce errors. Data structures are crucial in designing efficient software and play a critical role in algorithm design.
Common Data Structures
Understanding common data structures is essential for any programmer. These structures provide the building blocks for more complex systems and applications.
Arrays and Lists
Arrays and lists are primitive data structures that store collections of elements. Arrays have a fixed size, while lists can grow dynamically. You can use arrays for tasks that require fast access to elements by index. Lists are more flexible and allow for easy insertion and deletion of elements.
Stacks and Queues
Stacks and queues are linear data structures that follow specific order principles. A stack operates on a last-in, first-out (LIFO) basis, making it ideal for tasks like undo operations in software. A queue follows a first-in, first-out (FIFO) order, which is useful for managing tasks in a scheduling system.
Trees and Graphs
Trees and graphs are non-linear data structures that represent hierarchical and interconnected data. Trees, such as binary trees, are used in databases and file systems to organize data efficiently. Graphs model complex relationships and networks, making them suitable for social networks and transportation systems.
The Role of Data Structures in Efficiency
Organizing Data for Optimal Performance
Data structures play a pivotal role in organizing data for optimal performance. They provide a systematic way to manage and access data, ensuring that your programs run efficiently. By choosing the right data structure, you can minimize resource utilization and reduce execution time. This optimization is crucial when dealing with large datasets or complex systems.
-
Speed and Memory Usage: Data structures optimize performance by facilitating fast and accurate data access. They help improve memory and network management, allowing your programs to run faster and use less memory. For instance, using a hash table can significantly speed up data retrieval compared to a simple list.
-
Efficient Storage: Data structures enable efficient storage of large amounts of data. They are essential for managing databases and internet indexing services. By organizing data effectively, you can make informed decisions in software design and development.
-
Swift Retrieval and Modification: Data structures are architected to facilitate swift retrieval and modification of data elements. This leads to optimized performance, directly translating to faster program execution and enhanced responsiveness.
Enhancing Problem-Solving Skills
Leveraging data structures enhances your problem-solving skills. They provide the tools necessary to tackle complex challenges and develop efficient algorithms. Understanding how to organize and manipulate data effectively is key to becoming a proficient programmer.
-
Logical Reasoning: Data structures improve your logical reasoning abilities. They allow you to break down complex problems into manageable parts, making it easier to devise solutions. For example, using a tree structure can help you navigate hierarchical data efficiently.
-
Algorithm Design: Data structures are integral to designing efficient algorithms. They provide the foundation for developing solutions that handle vast amounts of data efficiently. By mastering data structures, you can create algorithms that are both effective and optimized for performance.
-
Real-World Applications: Data structures have practical applications in various fields. They are used in everything from cooking recipes to transportation systems. Understanding how to apply data structures in real-world scenarios enhances your ability to solve problems creatively and effectively.
Data Structures and Algorithms
Data structures and algorithms form the core of efficient software development. They work together to solve complex problems and optimize performance. Understanding their synergy is crucial for any programmer aiming to excel in software development.
How They Work Together
Data structures and algorithms complement each other in programming. Data structures organize and store data, while algorithms process this data to solve problems. You can think of data structures as the framework and algorithms as the tools that operate within this framework.
-
Efficient Data Handling: Algorithms rely on data structures to access and manipulate data efficiently. For example, sorting algorithms like quicksort use arrays to organize data quickly. This combination ensures that your programs run smoothly and efficiently.
-
Problem Solving: Data structures and algorithms provide a systematic approach to problem-solving. They allow you to break down complex problems into smaller, manageable tasks. By understanding how they work together, you can develop solutions that are both effective and optimized for performance.
-
Software Development: In software development, data structures and algorithms play a vital role. They help developers create scalable and maintainable software. By mastering these concepts, you can improve your software development skills and create high-quality applications.
Examples of Advanced Data Structures
Advanced data structures offer powerful tools for developers. They provide efficient solutions for complex problems in software development.
-
Hash Tables: Hash tables store data in key-value pairs, allowing for fast data retrieval. They are widely used in software development for tasks like caching and indexing.
-
Heaps: Heaps are specialized tree-based data structures that support priority queue operations. They are essential in algorithms like Dijkstra's shortest path and are used in software development for efficient task scheduling.
-
Graphs: Graphs model complex relationships and networks. They are used in algorithms for finding the shortest path, network flow, and more. Understanding graphs is crucial for developers working on social networks, transportation systems, and other interconnected applications.
-
Tries: Tries are tree-like data structures used for efficient string searching. They are commonly used in software development for tasks like autocomplete and spell-checking.
By exploring these advanced data structures, you can enhance your problem-solving skills and become a more proficient developer. They provide the foundation for developing efficient algorithms and creating high-performance software.
Impact on User Experience
Data structures significantly impact user experience by enhancing application performance. When you choose the right data structure, you ensure that your applications run smoothly and respond quickly to user interactions. This efficiency is crucial in today's fast-paced digital world, where users expect instant results and seamless experiences.
Improving Application Performance
-
Swift Data Access: Data structures like arrays and hash tables allow for rapid data retrieval. Imagine a scenario where you store a grocery list in an array. You can instantly access any item, leading to faster program execution and improved responsiveness. This speed directly translates to a better user experience.
-
Efficient Memory Usage: Selecting appropriate data structures helps optimize memory usage. For instance, using a B-tree can balance memory utilization, ensuring consistent performance regardless of data size. This optimization is vital for applications handling large datasets, as it prevents slowdowns and enhances scalability.
-
Algorithm Integration: Algorithms work hand-in-hand with data structures to process data efficiently. By integrating efficient algorithms, you can solve complex problems quickly, reducing computational costs. This synergy between data structures and algorithms ensures that your applications perform optimally, even under heavy loads.
-
Scalability and Flexibility: As your data grows, the choice of data structures becomes critical. Efficient data structures and algorithms provide the scalability needed to handle increasing data volumes without sacrificing performance. They offer the flexibility to adapt to changing requirements, ensuring your applications remain responsive and reliable.
Real-World Examples
-
Social Media Platforms: Social networks rely on graphs to model relationships between users. These data structures enable efficient data organization and retrieval, allowing platforms to deliver personalized content swiftly. Algorithms process this data to recommend friends or suggest content, enhancing user engagement.
-
Search Engines: Search engines use advanced data structures like tries and hash tables to index vast amounts of information. These structures facilitate quick data retrieval, enabling users to find relevant results in milliseconds. The integration of efficient algorithms further refines search results, improving user satisfaction.
-
E-commerce Websites: E-commerce platforms utilize data structures to manage product catalogs and user data. By employing efficient algorithms, they can offer personalized recommendations and streamline the checkout process. This optimization enhances the overall shopping experience, encouraging repeat visits.
-
Transportation Systems: Transportation networks use graphs to model routes and connections. Algorithms like Dijkstra's shortest path leverage these structures to calculate optimal routes, ensuring timely arrivals. This efficiency improves user satisfaction and trust in the system.
Incorporating the right data structures and algorithms into your applications is essential for delivering a superior user experience. By understanding their importance, you can create responsive, efficient, and scalable software that meets the demands of modern users.
Conclusion
Data structures are the backbone of efficient programming. They organize, store, and access data in ways that enhance performance and problem-solving skills. By mastering data structures, you empower yourself to create well-organized and responsive programs. Their role extends beyond traditional arguments, serving as essential tools in software development. Familiarity with these concepts is crucial for coding interviews and real-world applications. As you explore further, you'll find that understanding data structures not only improves your programming skills but also fosters critical thinking. Embrace this knowledge to excel in the ever-evolving tech landscape.