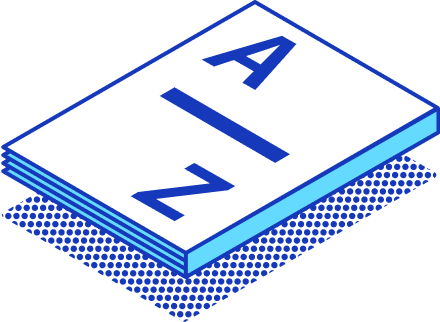
Cassandra Query Language (CQL)
What is Cassandra Query Language (CQL)
Cassandra Query Language (CQL) serves as the primary language for communicating with Apache Cassandra. CQL offers a model similar to SQL, making it familiar to those who have experience with relational databases. However, CQL is specifically designed to work with Cassandra's distributed, NoSQL architecture. The purpose of CQL is to provide a high-level language that abstracts away some of the low-level details of the Apache Cassandra database. This abstraction simplifies database interactions and optimizes data retrieval processes.
Comparison with SQL
CQL shares many similarities with SQL, such as the use of tables, rows, and columns. However, there are notable differences. CQL does not support JOIN operations, which are common in SQL. Instead, CQL relies on denormalization and the use of multiple tables to achieve similar results. CQL also lacks the GROUP BY clause, which means aggregation must be handled differently. Despite these differences, CQL retains a syntax that is easy to understand for those familiar with SQL.
Basic Syntax and Structure
Key Components of CQL Statements
CQL statements consist of several key components. These include:
-
Keywords: Words that define the action to be performed, such as
SELECT
,INSERT
,UPDATE
, andDELETE
. -
Identifiers: Names of keyspaces, tables, and columns.
-
Literals: Fixed values used in queries, such as numbers or strings.
-
Operators: Symbols that specify operations, such as
=
,<
,>
, andAND
.
Each CQL statement follows a specific structure, starting with a keyword and followed by other components in a defined order.
Commonly Used Commands
Several commands are frequently used in CQL. These include:
-
SELECT: Retrieves data from one or more tables.
SELECT * FROM users WHERE user_id = 1;
-
INSERT: Adds new data to a table.
INSERT INTO users (user_id, name, email) VALUES (1, 'John Doe', 'john.doe@example.com');
-
UPDATE: Modifies existing data in a table.
UPDATE users SET email = 'new.email@example.com' WHERE user_id = 1;
-
DELETE: Removes data from a table.
DELETE FROM users WHERE user_id = 1;
These commands form the foundation of most interactions with a Cassandra database using CQL.
Data Definition Language (DDL) in CQL
Creating Keyspaces
Syntax and Examples
Creating a keyspace in Cassandra involves defining the replication strategy and replication factor. The syntax for creating a keyspace is straightforward:
CREATE KEYSPACE keyspace_name
WITH REPLICATION = { 'class' : 'SimpleStrategy', 'replication_factor' : 3 };
In this example, keyspace_name
represents the name of the keyspace. The SimpleStrategy
class specifies that data will be replicated across multiple nodes. The replication_factor
determines the number of replicas for each piece of data.
Best Practices
-
Choose an Appropriate Replication Strategy: Use
SimpleStrategy
for single data centers. UseNetworkTopologyStrategy
for multiple data centers. -
Set a Proper Replication Factor: Ensure the replication factor matches the number of nodes in the cluster to avoid data loss.
-
Name Keyspaces Meaningfully: Use descriptive names for keyspaces to reflect their purpose.
Creating Tables
Syntax and Examples
Creating tables in Cassandra involves specifying column definitions, primary keys, and optional clustering columns. The basic syntax for creating a table is:
CREATE TABLE table_name (
column1 data_type,
column2 data_type,
PRIMARY KEY (column1)
);
For example, to create a table named users
:
CREATE TABLE users (
user_id UUID,
name TEXT,
email TEXT,
PRIMARY KEY (user_id)
);
Primary Keys and Clustering Columns
Primary keys in Cassandra ensure unique row identification. Clustering columns define the order of data storage within a partition. For example:
CREATE TABLE orders (
order_id UUID,
user_id UUID,
product_id UUID,
order_date TIMESTAMP,
PRIMARY KEY (user_id, order_date)
) WITH CLUSTERING ORDER BY (order_date DESC);
In this example, user_id
serves as the partition key, while order_date
acts as the clustering column. The CLUSTERING ORDER BY
clause specifies descending order for order_date
.
Altering and Dropping Tables
Syntax and Examples
Altering tables in Cassandra allows adding or modifying columns. The syntax for altering a table is:
ALTER TABLE table_name
ADD new_column data_type;
For example, to add a phone_number
column to the users
table:
ALTER TABLE users
ADD phone_number TEXT;
Dropping tables involves removing the entire table and its data. The syntax for dropping a table is:
DROP TABLE table_name;
For example, to drop the users
table:
DROP TABLE users;
Use Cases
-
Adding Columns: Use altering to accommodate new data requirements without disrupting existing data.
-
Dropping Tables: Remove obsolete tables to maintain database cleanliness and efficiency.
Data Manipulation Language (DML) in CQL
Data Manipulation Language (DML) in Cassandra Query Language (CQL) enables users to perform essential operations such as inserting, updating, and deleting data. Mastery of DML commands is crucial for effective database management.
Inserting Data
Syntax and Examples
The INSERT
command in CQL allows users to add new rows to a table. The basic syntax for an INSERT
statement is:
INSERT INTO table_name (column1, column2, column3) VALUES (value1, value2, value3);
For example, to insert a new user into the users
table:
INSERT INTO users (user_id, name, email) VALUES (uuid(), 'Jane Doe', 'jane.doe@example.com');
This command inserts a new row with a unique user ID, name, and email address.
Batch Inserts
Batch inserts enable multiple INSERT
statements to execute as a single operation. This approach improves performance by reducing the number of network round trips. The syntax for batch inserts is:
BEGIN BATCH
INSERT INTO users (user_id, name, email) VALUES (uuid(), 'John Smith', 'john.smith@example.com');
INSERT INTO users (user_id, name, email) VALUES (uuid(), 'Alice Johnson', 'alice.johnson@example.com');
APPLY BATCH;
This command inserts two new rows into the users
table within a single batch operation.
Updating Data
Syntax and Examples
The UPDATE
command in CQL modifies existing rows in a table. The basic syntax for an UPDATE
statement is:
UPDATE table_name SET column1 = value1, column2 = value2 WHERE primary_key_column = key_value;
For example, to update the email address of a user in the users
table:
UPDATE users SET email = 'new.email@example.com' WHERE user_id = some_uuid;
This command updates the email address of the specified user.
Conditional Updates
Conditional updates allow modifications only if specific conditions are met. The IF
clause specifies these conditions. The syntax for conditional updates is:
UPDATE table_name SET column1 = value1 WHERE primary_key_column = key_value IF condition;
For example, to update a user's email address only if the current email matches a specified value:
UPDATE users SET email = 'new.email@example.com' WHERE user_id = some_uuid IF email = 'old.email@example.com';
This command updates the email address only if the current email matches 'old.email@example.com'.
Deleting Data
Syntax and Examples
The DELETE
command in CQL removes rows from a table. The basic syntax for a DELETE
statement is:
DELETE FROM table_name WHERE primary_key_column = key_value;
For example, to delete a user from the users
table:
DELETE FROM users WHERE user_id = some_uuid;
This command removes the specified user from the table.
Use Cases
-
Removing Obsolete Data: Use the
DELETE
command to remove outdated or irrelevant data. -
Maintaining Data Integrity: Delete rows that no longer meet specific criteria to ensure data integrity.
-
Optimizing Storage: Regularly delete unnecessary data to optimize storage and improve database performance.
Querying Data with CQL
Basic Select Statements
Syntax and Examples
The SELECT
statement in CQL retrieves data from tables. The basic syntax includes the SELECT
keyword, followed by column names, the FROM
clause, and the table name.
SELECT column1, column2 FROM table_name;
For example, to retrieve user names and emails from the users
table:
SELECT name, email FROM users;
This command fetches the name
and email
columns from the users
table.
Filtering and Sorting Data
Filtering data involves using the WHERE
clause to specify conditions. Sorting data uses the ORDER BY
clause. For example, to filter users by a specific email domain and sort by name:
SELECT name, email FROM users WHERE email LIKE '%@example.com' ORDER BY name ASC;
This command retrieves users with emails ending in @example.com
and sorts them alphabetically by name.
Advanced Query Techniques
Using Indexes
Indexes in CQL enhance query performance by allowing faster data retrieval. Creating an index involves specifying the column to be indexed.
CREATE INDEX ON table_name (column_name);
For example, to create an index on the email
column in the users
table:
CREATE INDEX ON users (email);
This index speeds up queries filtering by the email
column.
Materialized Views
Materialized views provide a way to precompute and store query results. Creating a materialized view involves defining the view's structure and query.
CREATE MATERIALIZED VIEW view_name AS
SELECT column1, column2 FROM table_name
WHERE primary_key_column IS NOT NULL
PRIMARY KEY (primary_key_column, clustering_column);
For example, to create a view of active users sorted by registration date:
CREATE MATERIALIZED VIEW active_users AS
SELECT user_id, name, registration_date FROM users
WHERE user_id IS NOT NULL AND status = 'active'
PRIMARY KEY (user_id, registration_date);
This view precomputes active users and sorts them by registration date.
Aggregation Functions
Aggregation functions in CQL perform calculations on data sets. Common functions include COUNT
, SUM
, AVG
, MIN
, and MAX
.
SELECT COUNT(column_name) FROM table_name;
For example, to count the number of users:
SELECT COUNT(user_id) FROM users;
This command returns the total number of users in the users
table.
Best Practices and Tips for Using CQL
Performance Optimization
Indexing Strategies
Effective indexing strategies can significantly enhance query performance in Cassandra. Indexes allow faster data retrieval by providing a mechanism to quickly locate rows based on column values. However, improper use of indexes can lead to performance degradation.
-
Create Indexes on Frequently Queried Columns: Identify columns frequently used in
WHERE
clauses and create indexes on those columns. -
Avoid Over-Indexing: Excessive indexing can increase write latency. Limit the number of indexes to essential columns.
-
Use Composite Indexes: For queries involving multiple columns, consider creating composite indexes to improve query efficiency.
Query Tuning
Query tuning involves optimizing CQL queries to reduce execution time and resource consumption. Properly tuned queries can handle large datasets more efficiently.
-
Use Prepared Statements: Prepared statements reduce parsing overhead by pre-compiling queries. This approach improves performance for repetitive queries.
-
Limit Result Sets: Use the
LIMIT
clause to restrict the number of rows returned. This practice reduces the amount of data transferred and processed. -
Avoid Full Table Scans: Design queries to use partition keys and clustering columns. Full table scans can be resource-intensive and slow.
Security Considerations
Authentication and Authorization
Authentication and authorization are critical for securing Cassandra databases. Proper implementation ensures that only authorized users can access and modify data.
-
Enable Authentication: Configure Cassandra to require authentication for all connections. Use secure credentials for user accounts.
-
Implement Role-Based Access Control (RBAC): Assign roles with specific permissions to users. This approach limits access based on job functions.
-
Audit User Activity: Regularly review logs to monitor user activity. Auditing helps detect unauthorized access and potential security breaches.
Data Encryption
Data encryption protects sensitive information from unauthorized access. Implementing encryption ensures data confidentiality both at rest and in transit.
-
Encrypt Data at Rest: Use encryption mechanisms to protect data stored on disk. Enable Transparent Data Encryption (TDE) for keyspaces and tables.
-
Encrypt Data in Transit: Use SSL/TLS to encrypt data transmitted between clients and Cassandra nodes. Configure SSL certificates for secure communication.
-
Manage Encryption Keys Securely: Store encryption keys in a secure key management system. Regularly rotate keys to enhance security.
Conclusion
Cassandra Query Language (CQL) plays a vital role in managing Apache Cassandra databases. Mastery of CQL enables efficient data operations and enhances database performance. Practicing and exploring CQL further will solidify understanding and improve skills. Additional resources, such as official documentation and community forums, provide valuable insights for continuous learning.
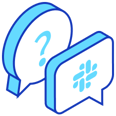
Join StarRocks Community on Slack
Connect on Slack