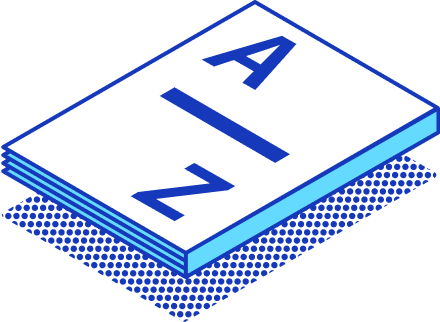
Data Access Object (DAO)
What is a DAO?
A Data Access Object (DAO) provides an abstract interface to a database or other persistence mechanisms. DAO isolates the data access logic from the business logic. This separation enhances code maintainability and reusability. Developers use DAOs to interact with databases without exposing the underlying database details.
Why use a DAO?
Using a Data Access Object (DAO) simplifies data operations. DAO allows developers to focus on business logic without worrying about database interactions. This approach improves productivity and reduces implementation time. The market capitalization of DAO tokens has exceeded $20 billion, highlighting the significant growth and adoption of DAOs in various projects.
Core Components of DAO
Interface
The interface defines the methods that the Data Access Object (DAO) will implement. The interface ensures consistency across different DAO implementations. Developers can easily switch between different databases or persistence mechanisms by changing the implementation of the interface.
Concrete Class
The concrete class implements the methods defined in the interface. The concrete class contains the actual code to interact with the database. Developers write the database-specific code in this class. This approach keeps the data access logic separate from the business logic.
Data Source
The data source represents the database or other persistence mechanisms. The data source can be a relational database, a NoSQL database, or even a file system. The Data Access Object (DAO) interacts with the data source to perform CRUD (Create, Read, Update, Delete) operations.
Benefits of Using DAO
Separation of Concerns
A Data Access Object (DAO) separates the data access logic from the business logic. This separation makes the code easier to understand and maintain. Developers can change the data access logic without affecting the business logic.
Code Reusability
Using a Data Access Object (DAO) promotes code reusability. Developers can reuse the same DAO across different projects. This approach saves time and effort. Developers do not need to write the same data access code multiple times.
Ease of Maintenance
A Data Access Object (DAO) makes the code easier to maintain. Developers can update the data access logic without changing the business logic. This approach reduces the risk of introducing bugs. The abstraction provided by the DAO simplifies unit testing.
Implementing Data Access Object (DAO) in Your Project
Setting Up the Environment
Required Tools and Libraries
To start, gather the necessary tools and libraries. For Java projects, use JDBC for database connectivity. Include JUnit for testing purposes. Use Maven or Gradle for dependency management. Ensure the availability of a database like MySQL or PostgreSQL.
Initial Configuration
Configure the project by setting up the database connection. Create a database.properties
file to store the database URL, username, and password. Load these properties in the application using a configuration class. This setup ensures secure and flexible database connections.
Creating the DAO Interface
Defining Methods
Define the methods required for data operations in the DAO interface. Common methods include create
, read
, update
, and delete
. These methods provide a standardized way to interact with the database.
public interface UserDao {
void createUser(User user);
User getUserById(int id);
void updateUser(User user);
void deleteUser(int id);
}
Example Code
Implement the UserDao
interface with the above methods. This example shows how to define methods for user-related data operations.
public class UserDaoImpl implements UserDao {
@Override
public void createUser(User user) {
// Implementation code
}
@Override
public User getUserById(int id) {
// Implementation code
return null;
}
@Override
public void updateUser(User user) {
// Implementation code
}
@Override
public void deleteUser(int id) {
// Implementation code
}
}
Implementing the DAO Class
Connecting to the Data Source
Establish a connection to the data source within the DAO class. Use JDBC for database connections. Load the database properties and create a connection object.
public class UserDaoImpl implements UserDao {
private Connection getConnection() throws SQLException {
Properties props = new Properties();
try (InputStream input = new FileInputStream("database.properties")) {
props.load(input);
} catch (IOException ex) {
ex.printStackTrace();
}
String url = props.getProperty("db.url");
String user = props.getProperty("db.user");
String password = props.getProperty("db.password");
return DriverManager.getConnection(url, user, password);
}
}
CRUD Operations
Implement the CRUD operations in the DAO class. Use SQL queries to interact with the database. This example demonstrates the createUser
method.
@Override
public void createUser(User user) {
String sql = "INSERT INTO users (name, email) VALUES (?, ?)";
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, user.getName());
pstmt.setString(2, user.getEmail());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
Example Code
Complete the implementation of the DAO class with all CRUD operations.
@Override
public User getUserById(int id) {
String sql = "SELECT * FROM users WHERE id = ?";
User user = null;
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
user = new User(rs.getInt("id"), rs.getString("name"), rs.getString("email"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return user;
}
@Override
public void updateUser(User user) {
String sql = "UPDATE users SET name = ?, email = ? WHERE id = ?";
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, user.getName());
pstmt.setString(2, user.getEmail());
pstmt.setInt(3, user.getId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void deleteUser(int id) {
String sql = "DELETE FROM users WHERE id = ?";
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
By following these steps, you can implement a robust DAO layer in your project. This approach ensures a clean separation between business logic and data access logic.
Best Practices and Common Pitfalls
Best Practices
Consistent Naming Conventions
Use consistent naming conventions in your DAO classes and methods. This practice ensures that code remains readable and maintainable. For example, use names like createUser
, getUserById
, updateUser
, and deleteUser
for CRUD operations. Consistent naming helps developers understand the purpose of each method quickly.
Error Handling
Implement robust error handling in your DAO classes. Catch exceptions and provide meaningful error messages. Log errors to help diagnose issues during development and production. Proper error handling prevents the application from crashing and makes debugging easier.
Documentation
Document your DAO classes and methods thoroughly. Include comments that explain the purpose of each method and any important details. Good documentation helps other developers understand and use your code effectively. It also aids in maintaining the codebase over time.
Common Pitfalls
Overcomplicating the DAO
Avoid overcomplicating your DAO classes. Keep the DAO focused on simple CRUD operations and basic data manipulations. Complex business logic should reside in the service layer, not in the DAO. Overcomplicating the DAO can make the code harder to maintain and understand.
Ignoring Performance Considerations
Consider performance when designing your DAO. Optimize SQL queries to reduce execution time. Use indexing and caching strategies to improve data retrieval speed. Ignoring performance considerations can lead to slow and inefficient applications.
Lack of Testing
Test your DAO classes thoroughly. Write unit tests to cover all CRUD operations and edge cases. Testing ensures that your DAO works correctly and helps catch bugs early. Lack of testing can result in unreliable code and difficult-to-diagnose issues.
By following these best practices and avoiding common pitfalls, you can create efficient and maintainable DAO layers in your projects.
Conclusion
A Data Access Object (DAO) provides an abstract interface to databases, isolating data access from business logic. This separation enhances code maintainability and reusability. Implementing a DAO involves setting up the environment, creating the DAO interface, and implementing the DAO class. Following best practices ensures efficient and maintainable code. Using DAOs simplifies data operations and improves productivity. Apply this knowledge to real-world projects to create scalable and efficient applications.
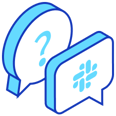
Join StarRocks Community on Slack
Connect on Slack