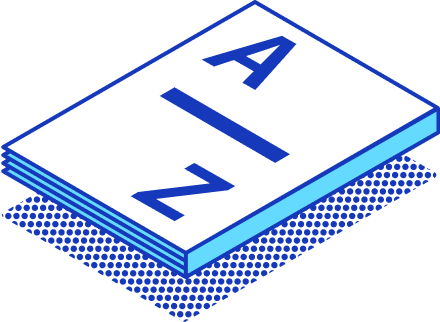
Data Encapsulation in Programming: A Beginner's Guide
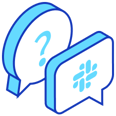
Join StarRocks Community on Slack
Connect on SlackUnderstanding Data Encapsulation
Definition of Data Encapsulation
Encapsulation is one of the fundamental principles of Object-Oriented Programming (OOP). Imagine a high-tech vault that stores sensitive documents. The vault has a secure locking mechanism, and only authorized personnel can access its contents through a specific procedure. This is exactly how encapsulation works in programming—it bundles data (the documents) and the methods that manipulate it (authorized procedures) into a single entity while restricting direct access.
In simple terms, encapsulation is the practice of hiding the internal details of an object and exposing only the necessary parts. This prevents unintended interference and ensures that an object’s internal workings are well protected.
Key Components of Data Encapsulation
Encapsulation is composed of three main elements:
-
Data Hiding: Only authorized methods can access specific data, preventing unintended modifications.
-
Abstraction: Complex logic is hidden behind simple interfaces, making it easier for programmers to use the object without needing to understand its internal workings.
-
Modularity: Code is divided into independent units, making development and debugging more manageable.
These principles ensure that software systems remain robust, maintainable, and secure, similar to how an ATM provides users with an interface to withdraw cash without exposing the complex transaction mechanisms inside.
Historical context and evolution
In the early days of programming, managing large codebases was a significant challenge. The introduction of OOP in the 1970s, pioneered by Alan Kay, emphasized encapsulation to enhance code organization and protect data integrity. This philosophy laid the groundwork for modern software development practices, where encapsulation is a key principle in designing scalable and maintainable applications.
How Data Encapsulation Works
Mechanisms of Encapsulation in Programming
Encapsulation is enforced using access modifiers and getter/setter methods:
-
Access Modifiers: These define which parts of the code can access a variable or method.
-
private
: Restricts access to within the class. -
protected
: Allows access within the same package or subclasses. -
public
: Grants access from anywhere.
-
-
Getters and Setters: These methods act as controlled gateways to an object’s data.
Examples of encapsulation in different programming languages
Encapsulation is a universal concept in OOP, but its implementation can vary across programming languages:
-
Java: You often use private fields with public getters and setters. This approach ensures that data remains protected while still being accessible through controlled methods.
public class Car {
private String model;
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
} -
Python: You use underscores to indicate private variables, and properties to manage access.
class Car:
def __init__(self):
self._model = None
@property
def model(self):
return self._model
@model.setter
def model(self, value):
self._model = value -
C++: You use similar techniques as Java, with private fields and public methods.
class Car {
private:
std::string model;
public:
std::string getModel() { return model; }
void setModel(std::string m) { model = m; }
};
These examples demonstrate how encapsulation can be implemented in various languages, each providing a unique way to protect and manage data.
Benefits of Data Encapsulation
Data Encapsulation offers numerous benefits that enhance your programming skills and improve the quality of your code. By understanding these advantages, you can create more efficient and secure applications.
Improved Code Maintainability
Encapsulation plays a vital role in maintaining clean and organized code. By bundling data and methods into a single unit, you create a clear structure that simplifies code management. This approach reduces complexity, making it easier for you to understand and modify your code. When you encapsulate data, you prevent external interference, which minimizes errors and enhances code reliability.
How Encapsulation Leads to Cleaner Code
-
Simplified Debugging: Encapsulation allows you to isolate issues within specific sections of your code, making debugging more straightforward.
-
Enhanced Readability: By organizing code into distinct units, encapsulation improves readability, aiding in collaboration and future development.
Real-World Examples of Maintainable Code
Consider a software application where encapsulation ensures that each module operates independently. For instance, in a banking system, you might encapsulate account details and transaction methods within a class. This separation allows you to update or expand features without disrupting the entire system.
Case Study: Banking Application Encapsulation helps maintain code by isolating account management from transaction processing. This separation ensures that changes in one area do not affect others, promoting stability and scalability.
Enhanced Security
Encapsulation significantly enhances data protection by restricting access to sensitive information. By using access modifiers like private
and protected
, you control who can view or modify data. This approach safeguards data integrity, ensuring that only authorized methods can alter it.
Case Study: Healthcare System A healthcare system uses encapsulation to secure patient data. By encapsulating medical records within classes, the system ensures that only authorized users can access or modify sensitive information, enhancing data protection and complying with privacy regulations.
Implementing Data Encapsulation
Best Practices for Beginners
Common Pitfalls to Avoid
-
Overexposing Data: Making all data public for easy access can lead to security risks.
-
Ignoring Constructor Initialization: Failing to initialize data members can lead to unexpected behavior.
-
Neglecting Getters and Setters: Direct access to data members bypasses validation.
-
Complex Constructor Logic: Avoid placing complex logic within the constructor method to ensure proper initialization.
Tools and Resources
Recommended programming languages and tools
Several programming languages and tools can help you enforce encapsulation:
-
Java: Known for its robust OOP features, Java provides strong support for encapsulation through access modifiers and constructor methods.
-
Python: While Python uses naming conventions for encapsulation, it offers decorators like
@property
to create getters and setters. -
C++: This language supports encapsulation with access specifiers and allows for detailed control over data access.
-
Kotlin: Offers modern OOP features, including encapsulation, with keywords like
private
,protected
, andinternal
.
Online resources and tutorials
To deepen your understanding of data encapsulation, explore these online resources:
-
Coursera: Offers comprehensive courses on OOP and encapsulation. You can learn from industry experts and gain practical experience.
-
OSI Model Tutorials: Understanding the OSI model can help you grasp how encapsulation works in networking, particularly in protocols like TCP.
-
TCP/IP Guides: These guides explain how encapsulation is used in network communication, offering a practical perspective on the concept.
By following these best practices and utilizing the recommended tools and resources, you can effectively implement data encapsulation in your programming projects. This will not only improve your coding skills but also enhance the security and maintainability of your applications.
Encapsulation and Access Modifiers
Understanding Access Modifiers
Private, Protected, and Public
Access modifiers are keywords in object-oriented programming languages that define the level of access to the members of a class. They play a vital role in Encapsulation and access modifiers, allowing you to control who can view or modify your data. Here are the three primary access modifiers:
- Private: This modifier restricts access to the class itself. When you create private fields, only methods within the same class can access them. This ensures that sensitive data remains protected from external interference。
- Protected: This modifier allows access within the same package and subclasses. It provides a balance between security and flexibility, enabling subclasses to inherit and use the data while keeping it hidden from other classes.
- Public: This modifier grants access to all classes. Use it sparingly, as it exposes your data to the entire program, potentially compromising security.
Implementing Access Modifiers in Code
Examples in Java and JavaScript
Let's explore how to use access modifiers in different programming languages, such as Java and JavaScript.
-
Java: In Java, you can create private fields and use getters and setters to manage access. This method ensures that data remains protected while still being accessible through controlled methods.
public class Tree {
private String commonName;
private String scientificName;
public String getCommonName() {
return commonName;
}
public void setCommonName(String commonName) {
this.commonName = commonName;
}
public String getScientificName() {
return scientificName;
}
public void setScientificName(String scientificName) {
this.scientificName = scientificName;
}
} -
JavaScript: Although JavaScript does not have built-in access modifiers, you can simulate them using closures or ES6 classes.
class Tree {
constructor() {
let commonName = '';
let scientificName = '';
this.getCommonName = function() {
return commonName;
};
this.setCommonName = function(name) {
commonName = name;
};
this.getScientificName = function() {
return scientificName;
};
this.setScientificName = function(name) {
scientificName = name;
};
}
}
Best Practices for Using Access Modifiers
-
Use Private Fields: Always create private fields for sensitive data. This practice prevents unauthorized access and accidental modifications.
-
Implement Getters and Setters: Use these methods to control how data is accessed and modified. They allow you to enforce rules and validations whenever data is accessed or changed.
-
Limit Public Access: Only use public access when necessary. Exposing too much data can lead to security vulnerabilities.
-
Leverage Protected Access: Use protected access to share data with subclasses while keeping it hidden from other classes. This approach promotes code reusability and modularity.
By understanding and applying access modifiers, you can enhance the security and maintainability of your code. This knowledge will empower you to create robust and efficient software solutions.
Practical Applications of Data Encapsulation
Data encapsulation serves as a cornerstone in the realm of software development. By bundling data and methods into a single unit, typically a class, you can protect sensitive information and control access to it. This approach not only enhances security but also promotes modularity and maintainability. Let's explore how encapsulation finds its place in various domains of software development and what the future holds for this fundamental concept.
Use Cases in Software Development
Examples in Web Development
In web development, encapsulation plays a pivotal role in creating robust applications. By encapsulating data within classes, you can ensure that only authorized methods access sensitive information. For instance, when developing a user authentication system, you might encapsulate user credentials within a class. This approach prevents unauthorized access and maintains data integrity.
-
User Authentication: Encapsulating user credentials ensures that only specific methods can access or modify them, enhancing security.
-
API Development: Encapsulation allows you to present a consistent interface to external systems, hiding the complex internal workings.
-
Session Management: Web applications often use encapsulated session objects to store user data securely and prevent direct manipulation.
Examples in Mobile App Development
Mobile app development also benefits significantly from encapsulation. By encapsulating data and behaviors within classes, you can create modular and maintainable code. This approach simplifies updates and feature additions, as changes to one part of the app do not affect others.
-
Data Management: Encapsulating data within classes ensures that only authorized methods can access or modify it, protecting user information.
-
UI Components: Encapsulation allows you to create reusable UI components, promoting code reusability and consistency across the app.
-
State Management: Mobile applications often encapsulate user preferences, app state, and settings into well-defined classes to prevent unintended alterations.
Examples in Enterprise Applications
Enterprise applications, such as large-scale business software, rely heavily on encapsulation for security, maintainability, and performance optimization.
-
Financial Systems: Banking and financial applications encapsulate transaction logic, ensuring that only approved operations can modify account balances.
-
Healthcare Systems: Medical records and patient information are encapsulated within classes, limiting access based on roles and permissions.
-
E-Commerce Applications: Shopping cart functionality, order processing, and payment systems use encapsulation to manage user transactions securely.
Future Trends and Innovations
Emerging Technologies Utilizing Encapsulation
As technology evolves, encapsulation continues to play a vital role in emerging fields. In areas like artificial intelligence and machine learning, encapsulation helps manage complex data structures and algorithms. By encapsulating data and methods, you can create modular and scalable systems that adapt to changing requirements.
-
AI and Machine Learning: Encapsulation enables the creation of modular algorithms that can be easily updated or replaced.
-
Internet of Things (IoT): Encapsulation helps manage data from various sensors and devices, ensuring secure and efficient communication.
-
Microservices Architecture: Modern software development is moving towards encapsulated microservices, where each service operates independently and communicates through well-defined interfaces.
Predictions for the Future of Encapsulation
The future of encapsulation looks promising, with advancements in programming languages and methodologies. As developers continue to embrace encapsulation, we can expect more secure and maintainable applications. The rise of functional programming and advanced information systems will further enhance the role of encapsulation in software development.
-
Functional Programming: Encapsulation will complement functional programming paradigms, offering a balance between data protection and simplicity.
-
Advanced Information Systems: Encapsulation will play a crucial role in managing complex data structures and ensuring data integrity.
By understanding and applying encapsulation, you can create robust and secure applications that stand the test of time. As you continue your journey in computer science, remember that encapsulation is not just a concept but a powerful tool that empowers you to build better software.
Conclusion
Data encapsulation is a cornerstone of OOP, providing a robust framework for managing data within a class. By bundling data and methods, you protect sensitive information and maintain code integrity. This practice encourages you to organize code logically, making it easier to understand and modify. As a beginner, embrace encapsulation to enhance your programming skills. Practice implementing private access to data, and use getters and setters to control interactions. Remember, encapsulation not only secures data but also streamlines code management. Enter fullscreen mode in your learning journey, and exit fullscreen mode with confidence in your coding abilities.