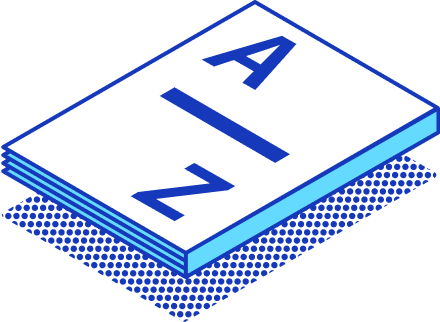
Data Partitioning
What is Data Partitioning?
Advantages of Data Partitioning
-
Scalability: Initially, most software projects start with simple, single-instance databases such as MySQL or Postgres. As these projects scale, the limitations of a single instance become apparent. Data partitioning facilitates horizontal scaling by allowing additional servers to integrate seamlessly, distributing the database load effectively and economically.
-
Availability: By distributing data across multiple partitions, the risk of a single point of failure decreases significantly. If one server or partition fails, the rest of the database can continue operating, thus maintaining the application's overall availability.
-
Performance: Partitioning can boost performance by reducing server load contention and localizing data, which minimizes latency. For example, geographic partitioning ensures that data is closer to users, reducing access times and improving user experience.
Common Methods of Data Partitioning
Vertical Partitioning
Vertical partitioning involves splitting a table by its columns, grouping related columns into separate tables. This allows each table to be stored and optimized for its specific access patterns. For instance, frequently updated transactional data can be separated from infrequently updated user demographic data.
-
Scenario: A financial services company stores user profiles and transaction records in a single database.
-
Use Case: User profile data (e.g., name, address, age) is updated less frequently than transaction data (e.g., purchases, balances), which changes multiple times a day.
-
Benefits:
- Performance: Queries that only need profile or transaction data can run faster by scanning smaller, more focused tables.
- Storage Optimization: Different storage technologies can be used for different types of data, such as faster storage for transactional data and slower, cost-efficient storage for profile data.
Horizontal Partitioning (Sharding)
Horizontal partitioning, commonly referred to as sharding, splits rows across different tables or servers based on a defined key, such as a range of values or hash. This method is useful for distributing large datasets across multiple machines, improving performance and scalability.
-
Scenario: An international e-commerce platform with millions of global transactions daily.
-
Use Case: The database must handle high transaction volumes and queries across multiple geographic regions efficiently.
-
Benefits:
- Scalability: Shards can be scaled independently to handle growth in specific regions or segments.
- Performance: Reduces latency by storing data closer to the users or systems accessing it frequently, balancing query loads across shards.
List, Range, and Hash Partitioning
These methods divide data based on key values, such as specific categories, value ranges, or hash functions. Each method distributes data to optimize performance and balance workloads.
List Partitioning
List partitioning divides data based on predefined list values. It's useful when data can be categorized into distinct groups, such as product types or geographic regions.
-
Scenario: A mobile app company that segments data based on app categories.
-
Use Case: App usage data is partitioned based on app type (e.g., social media, gaming, productivity), optimizing query performance for category-specific operations.
Range Partitioning
Range partitioning divides data based on a continuous range of values. This method is often applied to time-series or numeric data to improve query performance on range-based queries.
-
Scenario: A company managing sales data over multiple years.
-
Use Case: Partitioning sales data by year or month improves performance for historical data queries.
Hash Partitioning
Hash partitioning uses a hash function to distribute data evenly across partitions. This is particularly effective for systems with high write and read throughput, ensuring an even load distribution across database nodes.
-
Scenario: An online service handling high volumes of user-generated content and interactions.
-
Use Case: Hash partitioning ensures that user data is evenly distributed across nodes, preventing any single node from becoming a performance bottleneck.
-
Benefits of List, Range, and Hash Partitioning:
- Load Balancing: These methods distribute data and workload evenly across partitions, preventing performance bottlenecks.
- Query Performance: Localizing data based on keys (such as time or category) improves query efficiency and response times, especially for large datasets.
Each of these partitioning strategies can significantly enhance database performance, scalability, and manageability. The appropriate choice depends on specific data characteristics and business requirements. Vertical partitioning optimizes storage and access patterns, horizontal partitioning scales databases across regions, and list, range, or hash partitioning provides granular control for load balancing and query performance optimization.
Examples and Best Practices
Best Practices for Data Partitioning
-
Understand Your Data Access Patterns
Before implementing partitioning, it's crucial to analyze how your data is accessed and updated. Understanding data access patterns helps in choosing the most appropriate partitioning strategy. For example, if queries frequently access data from a specific time period, range partitioning by date might be the most effective. Analyze query logs and performance metrics to identify common access patterns.
-
Choose the Right Partition Key
Selecting the right partition key is pivotal. The key should divide the data into evenly sized partitions to prevent data skew, which can lead to unbalanced server loads and poor performance. Common partition keys include timestamps for time-based data, geographic location for distributed systems, or other business-specific identifiers that ensure even data distribution.
-
Keep Partitions Manageable in Size
Partitions should be large enough to reduce the overhead of managing many small partitions but small enough to improve query performance and maintenance tasks such as backups or data purges. The optimal size of a partition often depends on the specific database system and hardware capabilities but typically ranges from gigabytes to terabytes in size.
-
Use Partitioning and Indexing Together
While partitioning effectively narrows down the data that needs to be scanned for queries, indexing within those partitions can further accelerate access times. Indexes should be thoughtfully placed on columns that are often used in WHERE clauses or as JOIN keys.
-
Monitor and Adjust Partitions Regularly
As data grows and access patterns change, the initial partitioning strategy may need adjustment. Regular monitoring and maintenance of partitions are necessary to ensure they continue to meet performance expectations. This might include splitting, merging, or re-partitioning data as needed.
-
Automate Partition Management
For systems that support dynamic or automatic partitioning, like modern distributed databases, consider leveraging these features to reduce the administrative burden. Automatic partitioning can adjust to changing data volumes and patterns without manual intervention.
-
Test Partitioning Strategies Before Full Implementation
Implementing partitioning in a testing or staging environment first allows you to observe the impacts on performance without risking production stability. This practice is crucial, especially when dealing with large datasets or critical systems.
-
Consider Future Scalability
Design your partitioning schema with future growth in mind. The partitioning logic should accommodate increasing data volumes and potentially new types of queries or business requirements without significant rework.
-
Handle Cross-Partition Queries Efficiently
Queries that span multiple partitions can negate the benefits of partitioning if not handled carefully. Optimize these queries or redesign application logic to minimize the need for cross-partition access, which can be costly in terms of performance.
How StarRocks Supports Data Partitioning
Key Features of Data Partitioning in StarRocks
Dynamic Partitioning
StarRocks facilitates dynamic partitioning where data can be segmented automatically based on predefined rules. This allows for flexible handling of data as it grows, ensuring efficient data management without manual intervention.
Diverse Partitioning Methods
The platform supports several partitioning methods including range, list, and hash partitioning. This diversity allows users to choose the partitioning strategy that best fits their data structure and query needs, optimizing performance and resource utilization.
Range Partitioning
Data can be partitioned based on specific ranges of values, which is ideal for datasets that are logically segmented by date, price ranges, or other sequentially ordered metrics.
List Partitioning
For datasets that contain categorical data, list partitioning is ideal as it groups data into partitions based on predefined lists of key values, enhancing query performance on those specific segments.
Advanced Bucketing
In addition to basic partitioning, StarRocks offers advanced bucketing options, including random and hash bucketing, to further enhance data distribution and query efficiency within partitions.