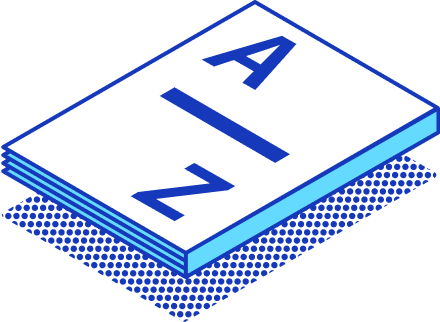
How to Boost Computational Speed with Vectorization
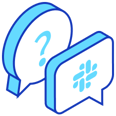
Join StarRocks Community on Slack
Connect on SlackVectorization processes multiple data points simultaneously, enabling faster computations. By replacing traditional loops with parallel operations, it reduces iteration overhead and boosts efficiency. For example, a Stanford study found vectorized matrix multiplication to be up to 25 times faster than nested loops. Similarly, Kaggle benchmarks show speed improvements of 2x to 100x in machine learning algorithms.
You encounter vectorization in real-world applications like natural language processing (NLP), image recognition, and recommendation systems. In NLP, it powers tasks such as sentiment analysis and language translation. In image processing, it accelerates feature extraction and object recognition. These examples highlight its critical role in performance-critical programming.
Key Takeaways
-
Vectorization handles many data points together, making tasks faster.
-
Tools like NumPy and Pandas in Python make this easy.
-
They help work with data quickly without writing many loops.
-
Find slow parts in your code, especially repeated loops, to fix.
-
Mixing vectorization with other tricks, like loop unrolling, helps more.
-
New compilers can speed up code, but learning manual ways gives control.
Understanding Vectorization
What Is Vectorization?
Vectorization is a programming technique that processes multiple data points simultaneously. Unlike traditional methods that handle one element at a time, vectorization applies the same operation to entire datasets in parallel. This approach relies on data parallelism, where identical instructions execute across multiple data elements at once. For example, instead of looping through an array to add a constant value to each element, vectorization performs the addition on all elements in a single step.
Key principles set vectorization apart from iterative processing:
-
It uses Single Instruction-Multiple Data (SIMD) instructions to handle multiple data elements in one operation.
-
It executes instructions on several elements per iteration, reducing the total number of iterations.
-
It optimizes memory access by working on contiguous blocks of data.
By leveraging these principles, vectorization minimizes computational overhead and accelerates execution.
How Vectorization Enhances Computational Efficiency
Vectorization significantly boosts performance by reducing the number of instructions your program needs to execute. SIMD instructions allow your CPU to process multiple data elements simultaneously, cutting down execution time. This efficiency becomes especially noticeable when working with large datasets or repetitive tasks like matrix operations.
Another advantage lies in memory optimization. SIMD improves cache locality by accessing contiguous memory blocks, which reduces cache misses and memory latency. This ensures faster data retrieval and smoother execution. Additionally, vectorization maximizes CPU core usage, minimizing idle time and enhancing energy efficiency. These benefits make vectorization a powerful tool for performance-critical applications.
Key Concepts: SIMD and Parallel Processing
SIMD and parallel processing form the backbone of vectorization. SIMD enables a single instruction to operate on multiple data points, streamlining execution. For instance, adding two arrays element-wise can be done in one step using SIMD, rather than looping through each element.
Parallel processing, on the other hand, involves dividing tasks across multiple processing units. While SIMD focuses on data-level parallelism, other techniques like Multiple Instruction-Multiple Data (MIMD) handle task-level parallelism. Here's a comparison of SIMD and MIMD:
Feature |
SIMD |
MIMD |
---|---|---|
Definition |
Single Instruction Multiple Data |
Multiple Instruction Multiple Data |
Memory Requirement |
Requires less memory |
Requires more memory |
Cost |
Less expensive |
More expensive |
Decoder |
Single decoder |
Multiple decoders |
Synchronization |
Latent synchronization |
Explicit synchronization |
SIMD's simplicity and efficiency make it ideal for vectorization, while MIMD excels in handling diverse computational tasks.
How Vectorization Works
The Role of SIMD in Vectorization
SIMD plays a central role in vectorization by enabling your processor to execute a single instruction across multiple data points simultaneously. This approach reduces processing time and enhances performance. For example, when adding two arrays element-wise, SIMD processes multiple elements in one step instead of iterating through each pair. This efficiency is particularly beneficial for performance-critical operations like matrix multiplication or image processing.
To implement SIMD vectorization effectively, you need to follow a structured approach:
-
Vectorizing the Code: Convert scalar operations into vectorized ones to handle multiple data points in a single operation.
-
Data Alignment: Ensure data is stored in memory in a way that aligns with SIMD requirements.
-
Loop Unrolling: Expand loop iterations to reduce overhead and improve efficiency.
-
Data Reordering: Optimize how data is accessed in memory to minimize cache misses.
-
Algorithm Optimization: Simplify calculations and reduce unnecessary memory access to maximize performance.
By following these steps, you can unlock the full potential of SIMD and achieve significant performance improvements.
Parallel Processing and Its Impact on Performance
Parallel processing divides tasks into smaller chunks and executes them simultaneously across multiple processing units. This approach complements SIMD by further reducing processing time. For instance, a Stanford study demonstrated that vectorized matrix multiplication could be up to 25 times faster than traditional nested loops. Similarly, Kaggle benchmarks revealed speed improvements ranging from 2x to 100x in machine learning algorithms. These examples highlight how parallel processing amplifies the benefits of vectorization.
In real-world applications, parallel processing reduces response times for tasks like recommendation systems. An e-commerce company reported a 70% decrease in response time after implementing vectorized operations. This demonstrates how parallel processing can transform performance-critical operations.
Examples of Vectorized Operations
Python Libraries (e.g., NumPy, Pandas)
Python libraries like NumPy and Pandas make vectorizing operators and expressions straightforward. NumPy, for example, allows you to perform element-wise operations on arrays without writing explicit loops. Consider this example:
import numpy as np
array = np.array([1, 2, 3, 4])
result = array * 2 # Vectorized operation
This code multiplies each element of the array by 2 in a single step, reducing processing time. Pandas offers similar functionality for dataframes, enabling efficient data manipulation.
C++ Libraries (e.g., SIMD Intrinsics, Intel MKL)
In C++, libraries like SIMD Intrinsics and Intel MKL provide low-level control for vectorized operations. SIMD Intrinsics allow you to write code that directly utilizes SIMD instructions, while Intel MKL offers pre-optimized routines for tasks like matrix multiplication. These tools enable you to achieve high performance in computationally intensive applications.
Modern CPUs and GPUs exploit built-in parallelization when using these libraries, further enhancing performance. By leveraging these tools, you can optimize your code for tasks requiring high computational efficiency.
Implementing Vectorization in Programming
Compiler Vectorization Techniques
Modern compilers play a crucial role in enabling vectorization. They use various techniques to optimize your code for better performance. These techniques allow you to process data in parallel, reducing execution time and improving efficiency. Here's a breakdown of the most widely used compiler vectorization techniques:
Technique Type |
Description |
---|---|
Automatic Vectorization |
Modern compilers can automatically identify opportunities for vectorization without programmer input. |
Explicit Vectorization |
Programmers can specify parallelism using directives like OpenMP or intrinsics. |
SIMD-enabled Functions |
Functions that explicitly describe SIMD behavior, allowing for targeted vectorization. |
SIMD Loops |
Loops that explicitly describe SIMD behavior, including variable usage and idioms like reductions. |
Data Alignment |
Ensures data is aligned in memory to optimize vectorization performance. |
Unit Stride |
Accessing memory in a sequential manner to assist vectorization. |
Loop Optimizations |
Techniques like loop interchange and unrolling that can enhance vectorization potential. |
By understanding these techniques, you can leverage compiler vectorization to improve code performance and achieve parallel optimizations.
Using Vectorization Libraries and APIs
Python Tools (e.g., NumPy, Pandas)
Python offers powerful libraries like NumPy and Pandas to simplify vectorization. These tools allow you to perform operations on entire datasets without writing explicit loops. For example, NumPy enables you to execute mathematical operations on arrays efficiently:
import numpy as np
data = np.array([1, 2, 3, 4])
result = data + 5 # Vectorized addition
Pandas, on the other hand, excels in handling structured data. You can apply vectorized operations to entire columns of a dataframe, making data analysis faster and more efficient.
C++ Tools (e.g., Intel MKL)
In C++, libraries like Intel MKL provide pre-optimized routines for vectorized operations. These libraries take advantage of SIMD instructions to enhance performance. For example, Intel MKL offers optimized functions for matrix multiplication, which is a common task in scientific computing. By using these tools, you can achieve significant performance gains in computationally intensive applications.
Writing Efficient Vectorized Code
Writing efficient vectorized code requires careful planning and adherence to best practices. Here are some tips to help you optimize your code:
-
Reserve capacity using
reserve()
to minimize reallocations. -
Use
at()
for safe element access instead of[]
. -
Apply
std::move()
to avoid unnecessary copies and transfer data efficiently. -
Understand the principles of vectorization to save time and improve performance.
-
Utilize libraries like NumPy and Pandas for numerical and data analysis tasks.
By following these practices, you can write code that takes full advantage of vectorization, ensuring faster execution and better performance.
Benefits and Challenges of Vectorization
Benefits of Vectorization
Faster Execution and Improved Performance
Vectorization accelerates computations by processing multiple data points simultaneously. This approach can make operations 10–100 times faster compared to non-vectorized methods. For example, in machine learning, vectorization improves database performance by efficiently utilizing hardware resources. By processing more data per instruction, vectorization achieves performance boosts of up to 16 times. These improvements are especially noticeable in tasks like matrix operations or large-scale data analysis, where speed is critical.
Reduced Resource Usage
Database vectorization optimizes resource usage by minimizing computational overhead. It reduces the number of instructions your processor needs to execute, which lowers energy consumption. Additionally, vectorization improves database performance by enhancing memory efficiency. It accesses contiguous memory blocks, reducing cache misses and ensuring smoother execution. This makes vectorization an excellent choice for applications requiring high efficiency, such as real-time data processing.
Challenges and Trade-offs
Hardware Dependencies
Vectorization relies heavily on hardware capabilities. Not all processors support advanced SIMD instructions, which can limit its effectiveness. You must ensure that your hardware aligns with the requirements of vectorized operations. This dependency can pose challenges when working with older systems or heterogeneous environments.
Power Consumption
While vectorization improves database performance, it can increase power consumption during intensive tasks. High-performance processors often consume more energy when executing vectorized operations. You need to balance the trade-off between speed and energy efficiency, especially in power-sensitive applications.
Debugging Complexity
Vectorized code can be dense and harder to debug. Managing dependencies and conditions within vectorized logic adds complexity. Handling large datasets also requires careful memory management to avoid slowdowns. Ensuring code readability and maintainability becomes crucial. Preprocessing data, such as cleaning and normalizing, is essential for accurate results. These challenges demand a structured approach to implementation.
Tips for Optimizing Code with Vectorization
Identifying Bottlenecks in Code
To optimize your code with vectorization, you must first identify the bottlenecks slowing it down. Start by analyzing loops that run for thousands or millions of iterations. These loops often present the best opportunities for parallel computing. Use tools like cProfile
to profile functions and pinpoint the slowest parts of your code. For more granular insights, line_profiler
can measure the execution time of individual lines.
Focus on loops that are computationally intensive and avoid those with dependencies that make them hard to parallelize. Benchmark specific code snippets using timeit
to understand their runtime. By targeting these areas, you can prioritize sections of your code that will benefit most from vectorization.
Using Profiling Tools to Measure Performance
Profiling tools help you measure the performance of your vectorized code and identify areas for improvement. Mojo's profiling ecosystem provides time-based measurements and hardware event monitoring. This allows you to correlate your algorithm's behavior with the physical hardware's response, enabling targeted optimizations.
Other tools like Intel® VTune™ Amplifier XE and TAU Performance System* offer advanced insights into parallel computing. Gprof* and ThreadSpotter* are also popular for analyzing execution patterns and memory usage. These tools give you a clear picture of how your code performs, helping you refine your vectorization strategies.
Combining Vectorization with Other Optimizations
Vectorization works best when combined with other optimization techniques. Loop transformations, such as loop interchange and loop peeling, can improve parallel computing by making your code more SIMD-friendly. Unrolling loops increases instruction-level parallelism by merging iterations, reducing overhead. Loop fission, which splits loops into smaller parts, eliminates dependencies that hinder vectorization.
These techniques complement vectorization by enhancing its effectiveness. For example, unrolling a loop before applying SIMD instructions can maximize the use of your processor's parallel computing capabilities. By combining these methods, you can achieve significant performance gains in your applications.
Vectorization transforms how you approach computational tasks by enabling parallel data processing. It improves efficiency, making operations 10–100 times faster, and enhances scalability for large datasets. Applications like image processing, NLP, and recommendation systems showcase its real-world impact. For instance, vectorized word embeddings streamline sentiment analysis, while pixel manipulation accelerates object recognition. By simplifying code and boosting performance, vectorization allows you to focus on high-level design. Explore vectorization tools and techniques to unlock faster execution and reduced resource usage in your performance-critical projects.
FAQ
What is the main benefit of vectorization for your CPU?
Vectorization allows your CPU to process multiple data points simultaneously. This reduces execution time and improves cpu performance. By optimizing how your code interacts with the cpu cache, vectorization ensures faster data retrieval and smoother execution.
Can vectorization improve database performance?
Yes, vectorization enhances database performance by reducing computational overhead. It processes large datasets efficiently and minimizes cache misses. This leads to faster query execution and better resource utilization.
How does vectorization affect algorithms?
Vectorization accelerates algorithms by enabling parallel data processing. It reduces the number of instructions your CPU executes, which boosts performance. This is especially useful for tasks like matrix operations or machine learning algorithms.
Do you need special hardware for vectorization?
Most modern CPUs support vectorization through SIMD instructions. However, older processors may lack this capability. To achieve performance boosts, ensure your hardware supports vectorized operations.
How can you optimize memory management for vectorization?
Efficient memory management optimization involves aligning data in memory and accessing it sequentially. This reduces cache misses and improves cpu performance. Tools like profiling software can help you identify areas for improvement.