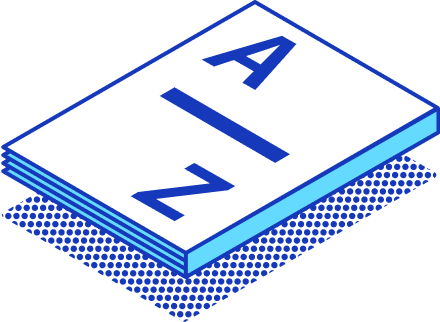
SQL Joins
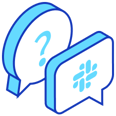
Join StarRocks Community on Slack
Connect on SlackWhat are SQL joins?
We'll explore different types of SQL joins, such as Inner Join, Left Join, Right Join, Full Outer Join, Cross Join, Self Join, Semi Join, and Anti Join. Additionally, we'll discuss join algorithms like Hash Join, Nested Loop Join, and Merge Sort Join, and join strategies including Local Joins, Distributed Joins, Broadcast Joins, Shuffle Joins, and Bucket Shuffle Joins.
What Are the Different Types of Joins in SQL?
employees
and salaries
. Below are the details for both tables, which we will use to demonstrate various types of SQL joins. Table 1: Employees
employee_id | name | department_id | manager_id |
1 | John Doe | 101 | 3 |
2 | Jane Smith | 102 | 3 |
3 | Alice Johnson | 103 | 1 |
4 | Chris Lee | 101 | 2 |
5 | Bob Brown | 104 | 1 |
Table 2: Salaries
employee_id | salary |
1 | 50000 |
2 | 60000 |
3 | 55000 |
4 | 58000 |
6 | 62000 |
What Is Inner Join?
employees
and salaries
tables:SELECT a.name, b.salary
FROM employees a
INNER JOIN salaries b ON a.employee_id = b.employee_id;
employees
and salaries
using the employee_id
column as the join condition. It will return rows only where there is a matching employee_id
in both tables, ensuring that only employees with corresponding salary records are listed.
What Is Left Outer Join (Left Join)?
SELECT a.name, b.salary
FROM employees a
LEFT JOIN salaries b ON a.employee_id = b.employee_id;
salaries
table. For employees without salary records, the salary
column in the result set will show NULL.What Is Right Outer Join (Right Join)?
SELECT a.name, b.salary
FROM employees a
RIGHT JOIN salaries b ON a.employee_id = b.employee_id;
name
field in the result will be NULL.What Is Full Outer Join (Full Join)?
SELECT a.name, b.salary
FROM employees a
FULL OUTER JOIN salaries b ON a.employee_id = b.employee_id;
What Is Cross Join?
SELECT a.name, b.salary
FROM employees a
CROSS JOIN salaries b;
employees
with each row from salaries
, leading to every possible combination.What Is Self Join?
SELECT A.employee_name AS Employee1, B.employee_name AS Employee2
FROM employees A, employees B
WHERE A.manager_id = B.employee_id;
employees
table is joined to itself to compare each employee against each other to find matching manager-employee relationships. The result lists pairs of employees where one is the manager of the other.What Is Semi Join?
SELECT a.name
FROM employees a
WHERE EXISTS (
SELECT 1
FROM salaries b
WHERE a.employee_id = b.employee_id
);
EXISTS
operator to check for the presence of at least one matching row in the salaries
table for each row in the employees
table. It returns only the names of those employees who have corresponding entries in the salaries
table.What Is ANTI Join?
SELECT a.name
FROM employees a
WHERE NOT EXISTS (
SELECT 1
FROM salaries b
WHERE a.employee_id = b.employee_id
);
NOT EXISTS
clause to check for the absence of matching rows in the salaries
table. It returns the names of employees who do not have corresponding salary entries, effectively performing an exclusion filter.
What are JOIN Algorithms?
Join algorithms are crucial for the performance of database operations involving joins, as they dictate how data from different tables is combined based on specific join conditions. The efficiency and effectiveness of SQL queries are significantly influenced by the choice of algorithm, which affects data access, comparison, and combination.
What Is Hash Join?
Implementation Details of Hash Joins
-
Creation of Hash Table: The smaller of the two tables is chosen to construct the hash table. This selection is crucial because a smaller table is more likely to fit entirely in memory, which is essential for the performance of hash joins.
-
Partitioning: If the smaller table cannot fit into memory, it may need to be partitioned. Though partitioning can help manage memory constraints, it generally detracts from performance as it introduces additional complexity and potential disk I/O.
-
Hash Bucket Formation: The hash values are calculated using a hash function, such as modulo 40, which results in the creation of a fixed number of hash buckets—in this case, 40. Each bucket contains items that share the same hash score, effectively grouping similar data points together.
-
Application to Join Column: The hash function is then applied to the join column of the larger table. The resultant hash values dictate which bucket the data from the larger table should be compared with, allowing the algorithm to look for potential matches in the corresponding buckets.
Performance Considerations and Use Cases
-
Data Warehouse Queries: Hash joins are particularly advantageous in data warehouse settings where small dimension tables are frequently joined with larger fact tables. The ability to quickly locate and compare relevant rows based on hash buckets can significantly speed up query processing.
-
Lack of Indexes: In scenarios where appropriate indexes are not available, a hash table can serve as an alternative indexing mechanism, facilitating faster searches than would be possible by scanning the entire table.
-
Memory Requirements: Adequate memory allocation is critical for the success of hash joins. Insufficient memory can lead to performance degradation, as parts of the hash table might need to be stored on disk, increasing access times and reducing the overall efficiency of the join.
What Is Nested Loop Join?
Operational Mechanics
-
Outer Loop: The algorithm begins with the outer loop, which scans each row of the outer table.
-
Inner Loop: For each row of the outer table, the inner loop iterates over every row in the inner table.
-
Match Test: During the inner loop's iteration, each pairing of outer and inner rows is tested against the join condition. If the condition is met (e.g., the join key of the outer row matches the join key of the inner row), the rows are combined into the result set.
Use Cases and Advantages
-
Small Datasets: Nested Loop Joins are effective when at least one of the tables is small enough that the inner table's full scan does not impose a significant performance penalty.
-
Lack of Indexes: This type of join can be useful if the tables do not have indexes on the join columns. Since it does not rely on the pre-existence of indexes or sorted data, it can be implemented in scenarios where other join types might be less efficient.
-
Selective Queries: It is particularly advantageous when the outer table is substantially filtered by other query conditions, reducing the number of rows that need to be checked in the inner loop.
What Is Merge Sort Join?
Implementation of Merge Join
-
Pre-Sorted Data: For a merge join to be effective, both tables need to be sorted by the join column. This prerequisite allows the join process to efficiently merge the two tables, as each element from one table can be directly compared with elements from the other table without unnecessary backtracking.
-
Sorting Mechanisms:
-
Best Case: The ideal scenario for a merge join is when both tables already have an index on the join column that can be leveraged for an indexed order scan. This setup minimizes the overhead as no additional sorting is required.
-
Alternative Case: If the necessary indexes are not available, the SQL engine must sort the tables before initiating the join. This sorting introduces additional computational costs and can impact overall performance.
-
Performance Considerations and Use Cases
-
Optimal Conditions: Merge joins perform best when indexes are present on the join columns of both tables, allowing the database to utilize indexed order scans to efficiently merge the tables. The presence of indexes eliminates the need for separate sorting steps, significantly enhancing performance.
-
Handling Differently Sized Tables: This method is also advantageous when joining a large indexed table with a smaller table that may need to be sorted first. Sorting a smaller table is relatively less resource-intensive and can be quickly aligned with the larger table’s indexed order for the merge process.
Join Strategies
Local Joins vs. Distributed Joins
Local joins and distributed joins are two methodologies for handling database queries, differentiated by data location. Local joins occur when all required data resides on a single server, facilitating faster and more efficient query processing due to reduced network overhead and no need for data transfer. Conversely, distributed joins involve data spread across multiple servers, necessitating data movement over the network to execute the join, which introduces latency but allows for handling larger datasets and scaling beyond the capacity of a single server.
What are Local Joins?
Traditionally, "local joins" refer to join operations performed within a single database node using data that resides entirely on that node. These joins do not involve data interactions between different nodes or clusters, making them inherently isolated and independent of network considerations.
In the context of non-distributed systems, local joins are straightforward as they involve accessing and combining data that is stored locally, without external dependencies. These joins are efficient due to the absence of network latency and are typically used in scenarios where all necessary data is available within a single server or database instance.
Local Joins in Distributed Systems
In distributed systems, the traditional concept of local joins is less applicable because data is typically partitioned across multiple nodes. Here, the terminology often shifts to more specific types of operations that mimic the "local" nature of traditional joins:
Co-located Joins
- A co-located join is a specific strategy used within distributed databases where the join operation is executed in a way that emulates local joins.
- In co-located joins, both tables involved in the join are distributed across multiple nodes such that all rows with the same join key are colocated on the same node. This setup ensures that the join can be performed locally at each node without requiring data transfer between nodes.
- This strategy significantly reduces network overhead and improves performance by leveraging local processing on each node. However, it requires careful upfront data organization and distribution based on expected join keys.
How Co-located Joins Work
-
Data Distribution: For a co-located join to be possible, both tables must be organized such that rows with the same join key are located on the same node. This alignment ensures that all necessary data for the join is already present locally, eliminating the need for data shuffling between nodes.
-
Join Execution: Since the data resides locally, the join can be performed quickly and efficiently on each node independently, without waiting for data to be transferred from other parts of the system.
-
Prior Knowledge Requirement: A critical aspect of implementing a co-located join is the requirement for advance knowledge of the join conditions. This knowledge is essential because it dictates how data must be distributed across nodes during the initial data ingestion process.
Characteristics and Considerations
-
Speed and Efficiency: Co-located joins are highly efficient due to the lack of network traffic and the ability to leverage local processing power. This efficiency makes them particularly suitable for scenarios where network bandwidth is a limitation or where rapid query response is necessary.
-
Flexibility: While co-located joins are fast, they lack flexibility. The data distribution strategy must be determined based on the anticipated join conditions, which means changes in query requirements might necessitate a reorganization of the data distribution, a potentially costly and time-consuming process.
What are Distributed Joins?
-
Minimizing Network Utilization: It’s essential to reduce the amount of data transferred between nodes to lower network traffic and enhance performance.
-
Minimizing Memory Utilization: Efficient memory use ensures that the system can handle large datasets without excessive consumption of resources, preventing bottlenecks.
Shuffling in Distributed Joins:
-
Purpose: Shuffling is the process of redistributing data across all participating nodes based on the join key. This is critical because it aligns the data from the joining tables on the same nodes, making the join operation possible.
-
Importance: Shuffling is vital for scalability in distributed joins. Without proper shuffling, the join might not scale efficiently as data grows because each node must have access to relevant pieces of data to perform its part of the join computation.
Distributed Joins - Broadcast Join
How Broadcast Joins Work
-
Data Broadcasting: In this approach, the smaller right table is replicated and sent to each worker node in the cluster. This means that each node receives a complete copy of the right table, allowing it to perform the join locally with its segment of the larger left table.
-
Network Overhead: For instance, if there are three nodes in a cluster and the right table consists of a thousand rows, the total network overhead would involve broadcasting these thousand rows to each of the three nodes, effectively multiplying the data transmitted across the network to three thousand rows. This can be resource-intensive and costly in terms of network bandwidth.
-
Memory Requirements: A critical limitation of the broadcast join is that the entire right table must fit into the memory of each worker node. If the right table is too large to fit into memory, the join cannot proceed, making this approach feasible only with sufficiently small right tables.
Suitability and Limitations
-
Cluster Size: Broadcast joins are more suitable for smaller clusters where the duplication of the right table across multiple nodes does not overwhelm network or memory resources.
-
Use Cases: This method is ideal for scenarios where the smaller right table can be easily accommodated in memory across all nodes, such as with small lookup tables that are frequently joined with larger transactional data.
-
Platform Specifics: Certain database systems like ClickHouse, which do not support data shuffling, are limited to using broadcast joins for distributing joins. This restriction makes understanding the memory and network implications of broadcast joins particularly important for users of such systems.
Distributed Joins - Shuffle Join
How Shuffle Joins Operate
-
Data Redistribution: In a shuffle join, both tables involved in the join are broken down into smaller segments according to their join keys. These segments are then shuffled across the cluster so that rows with the same join key from both tables end up on the same node.
-
Scalability: One of the significant advantages of shuffle joins is their scalability. As the number of nodes in the system increases, the join operation can distribute the workload more evenly across the cluster. This distribution allows for handling larger datasets and more complex queries without a proportional increase in memory demand per node.
-
Performance Enhancement: The distributed nature of shuffle joins means that as you add more nodes to the system, the performance of the join operation improves. Since each node handles a smaller portion of the data, the overall query execution times can decrease, making this approach highly effective for scaling large applications.
Key Advantages
-
Efficient Data Handling: Shuffle joins are designed to manage large volumes of data by effectively utilizing the distributed architecture of modern data systems. By ensuring that related data points are co-located on the same node, these joins minimize unnecessary data movement and optimize query performance.
-
Adaptability to Cluster Size: This join type adapts well to changes in cluster size, offering flexibility in resource management and planning. Whether expanding or contracting the number of nodes, shuffle joins maintain efficient operation, aligning well with dynamic computing environments.
Bucket Shuffle Join
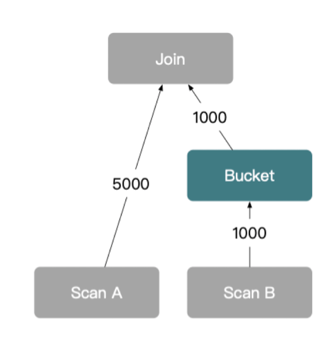
Operational Mechanics:
-
Selective Shuffling: If the join key matches the distribution key of the left table, only the right table needs to be shuffled. This selective shuffling reduces the amount of data that needs to be moved across the network.
-
Automatic Optimization: This strategy is automated in systems that support it, requiring no additional configuration from the user. It leverages existing data organization to minimize network traffic and maximize join efficiency.
Key Benefit:
-
Reduced Network Overhead: By only shuffling the necessary table, the network overhead is significantly reduced, making this strategy ideal for large datasets and high-query environments.
Conclusion