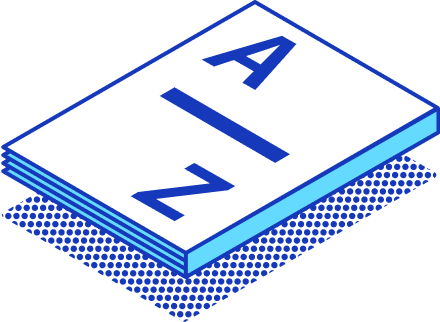
SQL Joins Explained: A Complete Resource
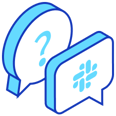
Join StarRocks Community on Slack
Connect on SlackUnderstanding SQL Joins
What are SQL Joins?
When you work with relational databases, you often need to combine data from multiple tables. SQL Joins provide the framework to do just that. They allow you to connect tables based on related columns, creating a cohesive dataset. This process is essential for effective data manipulation and extraction. By using SQL Joins, you can access data from different tables in a single query, which enhances your ability to analyze and interpret complex datasets.
SQL Joins act as the bridge between tables, enabling you to view data in a comprehensive manner rather than in isolation. For instance, if you have a table of customers and another table of orders, a Join Query can help you see which customers made which orders. This capability is crucial for gaining insights and making informed decisions based on relational data.
Understanding SQL Joins is not just about knowing how to write a Join Query. It's about mastering the art of combining data to create meaningful insights. As you progress in your journey to Learn SQL, mastering Joins becomes a fundamental skill.
Importance of SQL Joins in Data Management
In the world of data management, SQL Joins play a pivotal role. They are the magic that allows you to combine data from multiple tables, creating a comprehensive dataset. This capability is essential for anyone looking to extract valuable insights from complex datasets. By mastering SQL Joins, you gain the ability to manipulate data efficiently, which is crucial for effective database management.
Joins help you create a comprehensive dataset instead of viewing tables in isolation. This approach is vital for analyzing or extracting meaningful insights. When you use SQL Joins, you can connect and manipulate data spread across multiple tables. This ability is crucial for insightful data interpretation and effective database management.
As you Learn SQL Server, understanding the differences between various Joins is crucial for efficient data retrieval. Each type of Join serves a unique purpose, and knowing when to use each one can significantly enhance your data querying capabilities. Whether you're working with INNER JOIN, LEFT JOIN, or any other type, mastering these techniques will elevate your skills and enable you to make informed decisions based on relational data.
Types of SQL Joins
Understanding the different types of SQL Joins is crucial for anyone looking to master data manipulation. Each Join type serves a unique purpose, allowing you to retrieve data in various ways. Let's explore these types, starting with the INNER JOIN.
INNER JOIN
Syntax and Use Cases
The INNER JOIN is one of the most commonly used Joins in SQL. It retrieves rows that have matching values in both tables involved in the Join. This Join is ideal when you need to find records that exist in both datasets.
Syntax:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column = table2.column;
Use Cases:
-
When you want to find customers who have placed orders.
-
When you need to match employees with their respective departments.
Practical Example
Imagine you have two tables: Customers
and Orders
. You want to find all customers who have made purchases. Using an INNER JOIN, you can achieve this:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
INNER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
This query returns only those customers who have corresponding orders, providing a clear view of active transactions.
LEFT OUTER JOIN
Syntax and Use Cases
The LEFT OUTER JOIN, often referred to simply as LEFT JOIN, returns all rows from the left table and the matched rows from the right table. If no match exists, the result will contain NULLs for columns from the right table.
Syntax:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.column = table2.column;
Use Cases:
-
When you want to list all employees and their respective projects, including those without projects.
-
When you need to display all products, even those without sales.
Practical Example
Consider the Employees
and Projects
tables. To list all employees and their projects, including those not assigned to any project, use a LEFT JOIN:
SELECT Employees.EmployeeName, Projects.ProjectName
FROM Employees
LEFT JOIN Projects
ON Employees.EmployeeID = Projects.EmployeeID;
This query ensures that all employees are listed, with NULLs for those without projects.
RIGHT OUTER JOIN
Syntax and Use Cases
The RIGHT OUTER JOIN, or RIGHT JOIN, is similar to the LEFT JOIN but focuses on the right table. It returns all rows from the right table and the matched rows from the left table. If no match exists, the result will contain NULLs for columns from the left table.
Syntax:
SELECT columns
FROM table1
RIGHT JOIN table2
ON table1.column = table2.column;
Use Cases:
-
When you want to list all projects and their assigned employees, including projects without employees.
-
When you need to display all sales regions, even those without sales representatives.
Practical Example
Using the Projects
and Employees
tables again, you can list all projects and their assigned employees, including those without employees, with a RIGHT JOIN:
SELECT Projects.ProjectName, Employees.EmployeeName
FROM Projects
RIGHT JOIN Employees
ON Projects.EmployeeID = Employees.EmployeeID;
This query ensures that all projects are displayed, with NULLs for those without assigned employees.
Understanding these Basic SQL Join types enhances your ability to manipulate and retrieve data effectively. By mastering these Basic SQL techniques, you can handle complex queries with ease, making informed decisions based on comprehensive datasets.
FULL OUTER JOIN
Syntax and Use Cases
The FULL OUTER JOIN is a powerful tool in SQL that allows you to retrieve all rows from both tables involved in the join. If there is no match, the result will contain NULLs for columns where no match exists. This join type is particularly useful when you need a comprehensive view of data from two tables, including unmatched rows.
Syntax:
SELECT columns
FROM table1
FULL OUTER JOIN table2
ON table1.column = table2.column;
Use Cases:
-
When you want to see all authors and their books, even if some authors have not written any books.
-
When you need to list all suppliers and their products, including suppliers without products.
Practical Example
Imagine you have two tables: Authors Table
and Books
. You want to list all authors and their books, even if some authors have not written any books. Using a FULL OUTER JOIN, you can achieve this:
SELECT Authors.AuthorName, Books.BookTitle
FROM Authors
FULL OUTER JOIN Books
ON Authors.AuthorID = Books.AuthorID;
This query provides a complete list of authors and their books, including authors without books and books without authors.
CROSS JOIN
Syntax and Use Cases
The CROSS JOIN, also known as a Cartesian Join, combines every row from the first table with every row from the second table. This join type is useful when you need to explore all possible combinations of rows between two tables.
Syntax:
SELECT columns
FROM table1
CROSS JOIN table2;
Use Cases:
-
When you want to generate all possible combinations of products and sales regions.
-
When you need to explore all potential pairings of employees and projects.
Practical Example
Consider the Products
and Regions
tables. To generate all possible combinations of products and sales regions, use a CROSS JOIN:
SELECT Products.ProductName, Regions.RegionName
FROM Products
CROSS JOIN Regions;
This query results in a Cartesian product, showing every possible pairing of products and regions.
NATURAL JOIN
Syntax and Use Cases
The NATURAL JOIN automatically joins tables based on columns with the same name and compatible data types. This join type simplifies queries by eliminating the need to specify join conditions explicitly.
Syntax:
SELECT columns
FROM table1
NATURAL JOIN table2;
Use Cases:
-
When you want to join tables with common column names without specifying join conditions.
-
When you need to simplify queries involving tables with identical column names.
Practical Example
Suppose you have Employees
and Departments
tables with a common column DepartmentID
. To join these tables using a NATURAL JOIN, you can write:
SELECT EmployeeName, DepartmentName
FROM Employees
NATURAL JOIN Departments;
This query automatically joins the tables on the DepartmentID
column, providing a list of employees and their departments.
SELF JOIN
Syntax and Use Cases
A SELF JOIN is a unique type of join where a table is joined with itself. This technique is particularly useful when you need to compare rows within the same table. By using a SELF JOIN, you can analyze relationships between records in a single dataset, such as finding hierarchical data or comparing employees within the same department.
Syntax:
SELECT a.column_name, b.column_name
FROM table_name a, table_name b
WHERE a.common_column = b.common_column;
Use Cases:
-
When you want to find pairs of employees who work in the same department.
-
When you need to compare sales figures for different months within the same dataset.
Practical Example
Imagine you have an Employees
table with columns for EmployeeID
, EmployeeName
, and ManagerID
. You want to find out which employees report to the same manager. A SELF JOIN can help you achieve this:
SELECT e1.EmployeeName AS Employee, e2.EmployeeName AS Manager
FROM Employees e1, Employees e2
WHERE e1.ManagerID = e2.EmployeeID;
This query lists each employee alongside their manager, allowing you to see the reporting structure within the organization. By using SELF JOINs, you can effectively analyze and interpret complex relationships within a single table, enhancing your ability to Combine Data with SQL.
Comparing SQL Join Types
Understanding the differences between SQL Join types is crucial for effective data manipulation. Each Join type serves a unique purpose, allowing you to retrieve data in various ways. This section will guide you through the distinctions and help you choose the right Join for your query.
Differences Between Join Types
SQL Joins come in several varieties, each with its own characteristics and use cases. Here's a breakdown of the main types:
-
INNER JOIN: This Join retrieves only the rows with matching values in both tables. It's ideal when you need precise matches between datasets.
-
LEFT OUTER JOIN: Also known as LEFT JOIN, it returns all rows from the left table and the matched rows from the right table. If no match exists, the result includes NULLs for columns from the right table. This Join is useful when you want to include all records from the left table, even if they don't have corresponding matches.
-
RIGHT OUTER JOIN: Similar to the LEFT JOIN, but it focuses on the right table. It returns all rows from the right table and the matched rows from the left table. Use this Join when you need to include all records from the right table.
-
FULL OUTER JOIN: This Join combines the results of both LEFT and RIGHT Joins, returning all rows from both tables, with NULLs where no match exists. It's particularly helpful for comprehensive data analysis, where you need a complete view of the data, regardless of where matches exist.
-
CROSS JOIN: This Join produces a Cartesian product of the two tables, meaning every row from the first table is combined with every row from the second table. It's useful for exploring all possible combinations of rows between two tables.
-
NATURAL JOIN: This Join automatically joins tables based on columns with the same name and compatible data types. It simplifies queries by eliminating the need to specify join conditions explicitly.
-
SELF JOIN: A unique Join where a table is joined with itself. It's useful for comparing rows within the same table, such as finding hierarchical data or comparing employees within the same department.
Choosing the Right Join for Your Query
Selecting the appropriate Join type depends on your specific data retrieval needs. Here are some tips to help you make the right choice:
-
Use INNER JOIN when you need to find records that exist in both datasets. It's perfect for precise data matching.
-
Opt for LEFT OUTER JOIN if you want to include all records from the left table, even those without matches in the right table. This is useful for ensuring no data from the left table is omitted.
-
Choose RIGHT OUTER JOIN when you need to include all records from the right table, even if they lack matches in the left table.
-
Select FULL OUTER JOIN for a comprehensive view of data from both tables, including unmatched rows. This is ideal for thorough data analysis.
-
Apply CROSS JOIN when you want to explore all possible combinations of rows between two tables. Be cautious, as this can result in large datasets.
-
Use NATURAL JOIN to simplify queries involving tables with common column names. It automatically joins tables based on these columns.
-
Implement SELF JOIN when you need to compare rows within the same table. This is useful for analyzing relationships within a single dataset.
By understanding these differences and choosing the right Join type, you can effectively manipulate and retrieve data, enhancing your ability to perform in-depth analyses. Whether you're a beginner or an advanced user, mastering these techniques will elevate your SQL skills.
Best Practices for Using SQL Joins
Mastering SQL Joins can significantly enhance your data manipulation skills. By following best practices, you can optimize your queries and avoid common pitfalls. This section provides valuable tips to help you use SQL Joins effectively.
Tips for Optimizing SQL Joins
-
Understand Your Data: Before writing a Join query, familiarize yourself with the tables and their relationships. Knowing the structure and content of your data helps you choose the right Join type.
-
Use Indexes Wisely: Indexes can speed up Join operations by allowing the database to quickly locate matching rows. Ensure that the columns used in Join conditions are indexed for optimal performance.
-
Select Only Necessary Columns: Retrieve only the columns you need. This reduces the amount of data processed and improves query performance. Use the
SELECT
statement to specify the required columns. -
Filter Early: Apply filters as early as possible in your query. Use the
WHERE
clause to limit the data before performing the Join. This reduces the dataset size and speeds up the Join operation. -
Choose the Right Join Type: Different Join types serve different purposes. Use INNER JOIN for precise matches, LEFT JOIN to include all records from the left table, and FULL OUTER JOIN for a comprehensive view. Understanding these distinctions helps you select the most efficient Join for your needs.
-
Avoid Cartesian Products: Be cautious with CROSS JOINs, as they produce a Cartesian product of the tables. This can result in large datasets and slow performance. Use CROSS JOINs only when necessary.
Common Mistakes to Avoid
-
Ignoring Data Types: Ensure that the columns used in Join conditions have compatible data types. Mismatched data types can lead to errors or inefficient queries.
-
Overlooking NULL Values: Be aware of how NULL values affect your Join results. For example, INNER JOINs exclude rows with NULLs in the Join columns, while FULL OUTER JOINs include them.
-
Using Too Many Joins: Avoid using excessive Joins in a single query. Complex queries with multiple Joins can become difficult to manage and slow to execute. Break down complex queries into simpler parts if possible.
-
Neglecting Performance Testing: Always test the performance of your Join queries. Use tools like query analyzers to identify bottlenecks and optimize your queries accordingly.
-
Forgetting to Document: Document your Join queries, especially if they are complex. Clear documentation helps others understand your logic and makes future maintenance easier.
-
Not Considering Self Joins: Self Joins can be powerful for comparing rows within the same table. Use them to analyze hierarchical data or relationships within a dataset.
By following these best practices, you can effectively use SQL Joins to manipulate and retrieve data. Whether you're preparing for Big Tech Interviews or working on a complex project, mastering these techniques will enhance your SQL skills and improve your data analysis capabilities.
Conclusion
Mastering SQL Joins is crucial for effective data analysis. By understanding different Join types, you can efficiently retrieve and manipulate data from multiple tables. This skill is essential for tackling Analyst SQL Interview Questions and Data Engineer Interview Questions. To further enhance your knowledge, explore various resources, including Books and online courses. Using the right Join type, such as Full Outer Join, significantly impacts database performance. Whether you're preparing for a Data Analyst SQL Interview or an Amazon SQL Interview Questions session, a solid grasp of Joins will set you apart. Keep learning and refining your skills to excel in any Data Engineer SQL Interview.